


Detailed explanation of PHP quick sort algorithm, detailed explanation of sorting algorithm_PHP tutorial
Detailed explanation of PHP quick sort algorithm, detailed explanation of sorting algorithm
Concept
Here is a picture borrowed from Baidu Encyclopedia, which is very vivid:
The quick sort algorithm is an optimization of the bubble algorithm. His idea is to split the array first, put the large element values into a temporary array, and put the small element values into another temporary array (the dividing point can be any element value in the array, generally Use the first element, i.e. $array[0]), then continue to repeat the above splitting of these two temporary arrays, and finally merge the small array elements and the large array elements. The idea of recursion is used here.
PHP implementation
/*
Quick sort
*/
function quickSort($array)
{
If(!isset($array[1]))
return $array;
$mid = $array[0]; //Get a keyword for splitting, usually the first element
$leftArray = array();
$rightArray = array();
foreach($array as $v)
{
if($v > $mid)
$rightArray[] = $v; //Put numbers larger than $mid into an array
if($v < $mid)
$leftArray[] = $v; //Put the number smaller than $mid into another array
}
$leftArray = quickSort($leftArray); //Split the smaller array again
$leftArray[] = $mid; //Add the divided elements to the end of the small array, don’t forget it
$rightArray = quickSort($rightArray); //Split the larger array again
Return array_merge($leftArray,$rightArray); //Combine two results
}
Comparison with bubble algorithm
Here I compare the sorting implemented by the bubble algorithm I wrote before. It can be seen that this algorithm is much more efficient than the bubble algorithm.
$a = array_rand(range(1,3000), 1500); //Even when the bubble algorithm exceeds 1600 elements, a message of insufficient memory will appear, but here in order to measure the difference between the two, just set It becomes 1500, which ensures that the bubble algorithm can be completed.
shuffle($a); //Get the array that has been shuffled
$t1 = microtime(true);
quickSort($a); //Quick sort
$t2 = microtime(true);
echo (($t2-$t1)*1000).'ms
';
require('./maopao.php'); //What is quoted here is the bubble algorithm sorting I wrote before
$t1 = microtime(true);
maoPao($a); //Bubble
$t2 = microtime(true);
echo (($t2-$t1)*1000).'ms';
Run result:
12.10880279541ms
772.64094352722ms

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










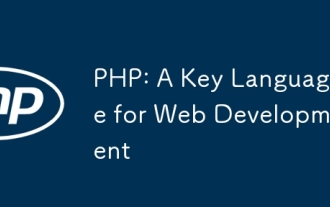
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
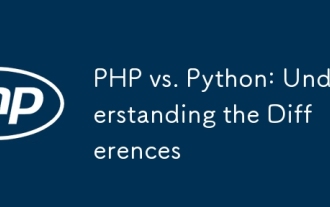
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
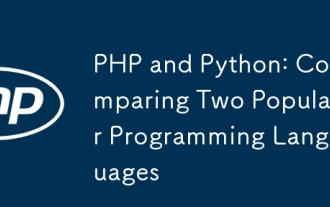
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
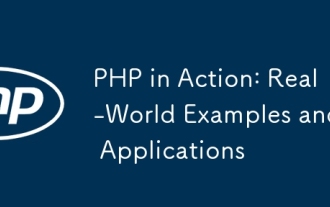
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
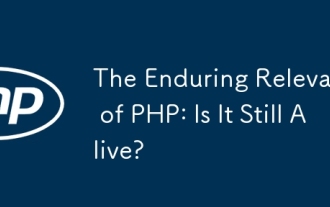
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
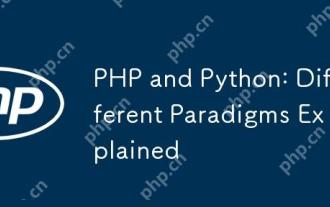
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
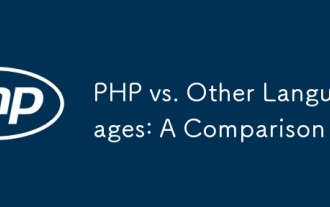
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
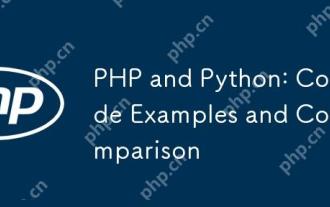
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
