General mysql database connection class code_PHP tutorial
General mysql tutorial database tutorial connection class code
Database connection is a limited and expensive resource, and database connection affects the performance indicators of the program. The database connection pool was proposed to address this problem. The database connection pool is responsible for allocating, managing and releasing database connections. It allows applications to reuse an existing database connection instead of re-establishing one; release database connections whose idle time exceeds the maximum idle time to avoid errors due to failure to release the database connection. Caused by missing database connection. This technology can significantly improve the performance of database operations.
/*
* created on 2010-3-8
* make by:suniteboy
* my first mysql class
*
*/
class mysql{
private $server ="";
private $user ="";
private $pwd ="";
private $database="";
private $linkmode = 1; //Connection mode, 0 means normal connection, 1 means permanent connection
private $conn = 0;
private $sql =""; //sql statement
private $result =""; //query results
private $record =""; //Save record
//============================================
//Constructor
//============================================
public function __construct($server,$user,$pwd,$database,$charset="utf8",$linkmode=0)
{
if(empty ( $server )| empty( $user ) | empty( $database ))
{
$this->show_error("The connection information is incomplete, please check whether the server address, user name and connected database information are provided");
Return 0;
}
$this->server = $server;
$this->user = $user;
$this->pwd = $pwd;
$this->database = $database;
$this->charset = $charset;
$this->linkmode = $linkmode;
$this->connect();
}
//============================================
//Connection function
//============================================
public function connect()
{
$this->conn = $this->linkmode?mysql_pconnect($this->server,$this->user,$this->pwd):
mysql_connect($this->server,$this->user,$this->pwd);
if(!$this->conn)
{
$this->show_error('Unable to connect to server');
Return 0;
}
if(!mysql_select_db($this->database))
{
$this->show_error('Unable to connect to database'.$this->database);
Return 0;
}
// $this->query('set names '.$this->charset);
return $this->conn;
}
//============================================
// mysql query function
//============================================
public function query($sql)
{
if(empty($sql))
{
$this->show_error('sql statement is empty');
Return 0;
}
$this->sql = preg_replace('/ {2,}/',' ',trim($sql));
$this->result = mysql_query($this->sql,$this->conn);
if(!$this->result)
{
$this->show_error('sql statement error',true);
Return 0;
}
return $this->result;
}
//============================================
// Function
//============================================
public function select_db($dbname)
{
Return mysql_select_db($dbname);
}
public function fetch_array($query,$result_type=mysql_assoc)
{
Return mysql_fetch_array($query,$result_type);
}
public function fetch_row($query)
{
Return mysql_fetch_row($query);
}
//============================================
// Get the number of record rows affected by the previous mysql operation
//============================================
public function affected_rows()
{
Return mysql_affected_rows();
}
public function num_fields($query)
{
Return mysql_num_fields($query);
}
public function num_rows($query)
{
Return @mysql_num_rows($query);
}
public function insert_id()
{
Return mysql_insert_id();
}
public function close()
{
Return mysql_close();
}
//============================================
// Get a result from the record
//============================================
public function getone($sql)
{
$res = $this->query($sql);
if($res!==false)
{
$row = mysql_fetch_row($res);
if($row!==false)
{
Return $row;
}
else
{
Return '';
}
}
else
{
Return false;
}
}
//============================================
// Get all results from the record
//============================================
public function getall($sql)
{
$res = $this->query($sql);
if($res!==false)
{
$arr = array();
while($row = mysql_fetch_assoc($res))
{
$arr[] = $row;
}
Return $arr;
}
else
{
Return false;
}
}
//============================================
// Error prompt function
//============================================
public function show_error($msg='',$sql=false)
{
$err = '['.mysql_errno().']'.mysql_error();
if($sql) $sql='sql statement:'.$this->sql;
if($msg=='')
{
echo $err;
echo "";
}
elseif($sql &&$msg)
{
echo $msg;
echo "";
echo $sql;
}
else
{
echo $msg;
echo "";
}
}
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
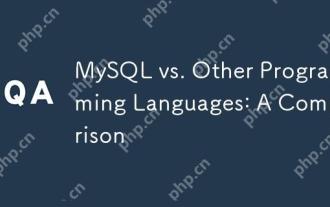
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.

Oracle is not only a database company, but also a leader in cloud computing and ERP systems. 1. Oracle provides comprehensive solutions from database to cloud services and ERP systems. 2. OracleCloud challenges AWS and Azure, providing IaaS, PaaS and SaaS services. 3. Oracle's ERP systems such as E-BusinessSuite and FusionApplications help enterprises optimize operations.
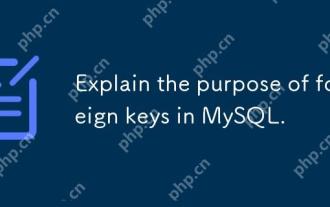
In MySQL, the function of foreign keys is to establish the relationship between tables and ensure the consistency and integrity of the data. Foreign keys maintain the effectiveness of data through reference integrity checks and cascading operations. Pay attention to performance optimization and avoid common errors when using them.
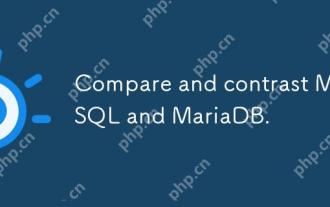
The main difference between MySQL and MariaDB is performance, functionality and license: 1. MySQL is developed by Oracle, and MariaDB is its fork. 2. MariaDB may perform better in high load environments. 3.MariaDB provides more storage engines and functions. 4.MySQL adopts a dual license, and MariaDB is completely open source. The existing infrastructure, performance requirements, functional requirements and license costs should be taken into account when choosing.

SQL is a standard language for managing relational databases, while MySQL is a database management system that uses SQL. SQL defines ways to interact with a database, including CRUD operations, while MySQL implements the SQL standard and provides additional features such as stored procedures and triggers.
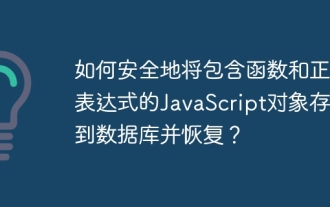
Safely handle functions and regular expressions in JSON In front-end development, JavaScript is often required...
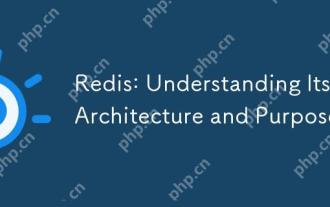
Redis is a memory data structure storage system, mainly used as a database, cache and message broker. Its core features include single-threaded model, I/O multiplexing, persistence mechanism, replication and clustering functions. Redis is commonly used in practical applications for caching, session storage, and message queues. It can significantly improve its performance by selecting the right data structure, using pipelines and transactions, and monitoring and tuning.
