


Brief analysis of php natsort kernel function Page 1/2_PHP Tutorial
Official manual (http://us.php.net/manual/en/function.natsort.php)
bool natsort ( array &$array )
This function implements a sort algorithm that orders alphanumeric strings in the way a human being would while maintaining key/value associations. This function implements a sort algorithm that orders alphanumeric strings in the way a human being would while maintaining key/value associations. is described as a "natural ordering". An example of the difference between this algorithm and the regular computer string sorting algorithms (used in sort()) can be seen in the example below.
According to the official The manual also gives you something like this:
img1.png img2.png img10.png img12.png
Obviously this is great for sorting similar filenames. Judging from the results, this natural algorithm should remove the non-numeric parts of the head and tail, and then sort the remaining numeric parts. Whether it is true or not, let's take a look at the PHP source code.
//The relevant code extracted from ext/standard/array.c is as follows
static int php_array_natural_general_compare(const void *a, const void *b, int fold_case) /* {{{ */
{
Bucket *f, *s;
zval *fval, *sval;
zval first, second;
int result;
f = *((Bucket **) a);
s = *((Bucket **) b);
fval = *(( zval **) f->pData);
sval = *((zval **) s->pData);
first = *fval;
second = *sval;
if (Z_TYPE_P(fval) != IS_STRING) {
zval_copy_ctor(&first); zval_copy_ctor(&second );
convert_to_string(&second);
}
result = strnatcmp_ex(Z_STRVAL(first), Z_STRLEN(first), Z_STRVAL(second), Z_STRLEN(second), fold_case);
if (Z_TYPE_P (fval) != IS_STRING) {
zval_dtor(&first);
}
if (Z_TYPE_P(sval) != IS_STRING) {
zval_dtor(&second);
}
return result;
}
/* }}} */
static int php_array_natural_compare(const void *a, const void *b TSRMLS_DC) /* {{{ */
{
return php_array_natural_general_compare (a, b, 0);
}
/* }}} */
static void php_natsort(INTERNAL_FUNCTION_PARAMETERS, int fold_case) /* {{{ */
{
zval * array;
if (zend_parse_parameters(ZEND_NUM_ARGS() TSRMLS_CC, "a", &array) == FAILURE) {
return;
}
if (fold_case) {
if (zend_hash_ sort(Z_ARRVAL_P (array), zend_qsort, php_array_natural_case_compare, 0 TSRMLS_CC) == FAILURE) {
y), zend_qsort, php_array_natural_compare, 0 TSRMLS_CC) == FAILURE) {
return; &array_arg)
Sort an array using natural sort */
PHP_FUNCTION(natsort)
{
php_natsort(INTERNAL_FUNCTION_PARAM_PASSTHRU, 0);
}
/* }}} */
Although this is the first time to view the core code of PHP, with many years of experience in reading code, it is easy to find that the core of this natural sorting algorithm is the function: strnatcmp_ex (located in the ext/standard/strnatcmp.c file).

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










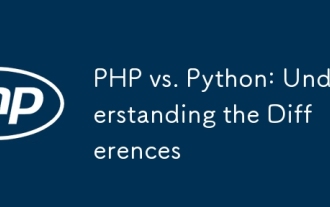
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
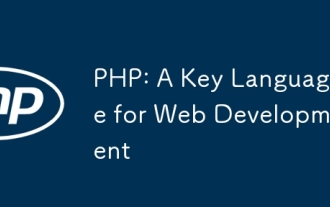
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
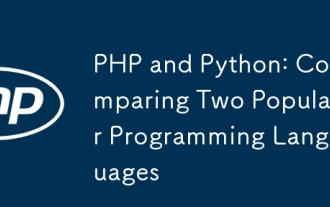
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
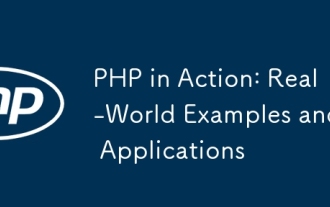
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
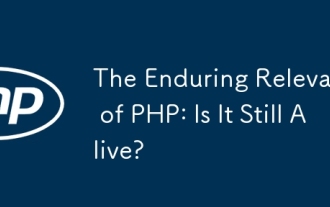
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
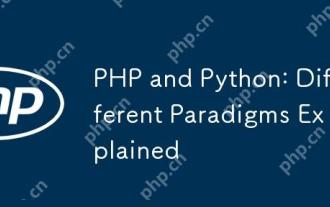
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
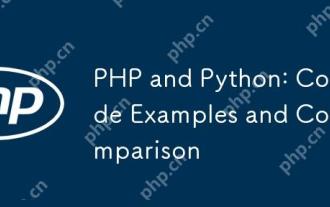
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
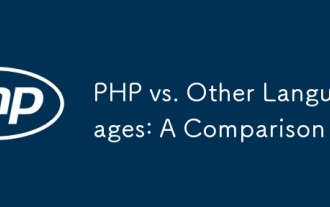
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
