


java - Please help me convert this DES encryption code into PHP. Is this the DES algorithm?
<code>import sun.misc.BASE64Decoder; import sun.misc.BASE64Encoder; import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.SecretKeyFactory; import javax.crypto.spec.DESKeySpec; import java.io.IOException; import java.security.SecureRandom; public class PHPDESEncrypt { String key; public PHPDESEncrypt() { } public PHPDESEncrypt(String key) { this.key = key; } public byte[] desEncrypt(byte[] plainText) throws Exception { SecureRandom sr = new SecureRandom(); DESKeySpec dks = new DESKeySpec(key.getBytes()); SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES"); SecretKey key = keyFactory.generateSecret(dks); Cipher cipher = Cipher.getInstance("DES"); cipher.init(Cipher.ENCRYPT_MODE, key, sr); byte data[] = plainText; byte encryptedData[] = cipher.doFinal(data); return encryptedData; } public byte[] desDecrypt(byte[] encryptText) throws Exception { SecureRandom sr = new SecureRandom(); DESKeySpec dks = new DESKeySpec(key.getBytes()); SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES"); SecretKey key = keyFactory.generateSecret(dks); Cipher cipher = Cipher.getInstance("DES"); cipher.init(Cipher.DECRYPT_MODE, key, sr); byte encryptedData[] = encryptText; byte decryptedData[] = cipher.doFinal(encryptedData); return decryptedData; } public String encrypt(String input) throws Exception { return base64Encode(desEncrypt(input.getBytes())).replaceAll("\\s*", ""); } public String decrypt(String input) throws Exception { byte[] result = base64Decode(input); return new String(desDecrypt(result)); } public String base64Encode(byte[] s) { if (s == null) return null; BASE64Encoder b = new sun.misc.BASE64Encoder(); return b.encode(s); } public byte[] base64Decode(String s) throws IOException { if (s == null) { return null; } BASE64Decoder decoder = new BASE64Decoder(); byte[] b = decoder.decodeBuffer(s); return b; } public static void main(String[] args) { try { PHPDESEncrypt d = new PHPDESEncrypt("12345678"); String p = d.encrypt("love"); System.out.println("密文:" + p); } catch (Exception e) { e.printStackTrace(); } } public String getKey() { return key; } public void setKey(String key) { this.key = key; } } </code>
回复内容:
<code>import sun.misc.BASE64Decoder; import sun.misc.BASE64Encoder; import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.SecretKeyFactory; import javax.crypto.spec.DESKeySpec; import java.io.IOException; import java.security.SecureRandom; public class PHPDESEncrypt { String key; public PHPDESEncrypt() { } public PHPDESEncrypt(String key) { this.key = key; } public byte[] desEncrypt(byte[] plainText) throws Exception { SecureRandom sr = new SecureRandom(); DESKeySpec dks = new DESKeySpec(key.getBytes()); SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES"); SecretKey key = keyFactory.generateSecret(dks); Cipher cipher = Cipher.getInstance("DES"); cipher.init(Cipher.ENCRYPT_MODE, key, sr); byte data[] = plainText; byte encryptedData[] = cipher.doFinal(data); return encryptedData; } public byte[] desDecrypt(byte[] encryptText) throws Exception { SecureRandom sr = new SecureRandom(); DESKeySpec dks = new DESKeySpec(key.getBytes()); SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES"); SecretKey key = keyFactory.generateSecret(dks); Cipher cipher = Cipher.getInstance("DES"); cipher.init(Cipher.DECRYPT_MODE, key, sr); byte encryptedData[] = encryptText; byte decryptedData[] = cipher.doFinal(encryptedData); return decryptedData; } public String encrypt(String input) throws Exception { return base64Encode(desEncrypt(input.getBytes())).replaceAll("\\s*", ""); } public String decrypt(String input) throws Exception { byte[] result = base64Decode(input); return new String(desDecrypt(result)); } public String base64Encode(byte[] s) { if (s == null) return null; BASE64Encoder b = new sun.misc.BASE64Encoder(); return b.encode(s); } public byte[] base64Decode(String s) throws IOException { if (s == null) { return null; } BASE64Decoder decoder = new BASE64Decoder(); byte[] b = decoder.decodeBuffer(s); return b; } public static void main(String[] args) { try { PHPDESEncrypt d = new PHPDESEncrypt("12345678"); String p = d.encrypt("love"); System.out.println("密文:" + p); } catch (Exception e) { e.printStackTrace(); } } public String getKey() { return key; } public void setKey(String key) { this.key = key; } } </code>
des加密有好几种。。不知道你这种是哪个。。。这里有两种,你可以对照一下php Des加密
这个……看你贴的代码是java
吧!你要用php
的DES
解密需要至少得需要知道加密key
和对应的加密模式例如是CBC
,CFB
,ECB
等!确认好之后,用楼上的给你的dome应该没问题的

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










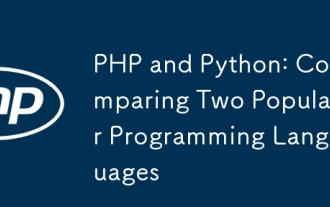
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
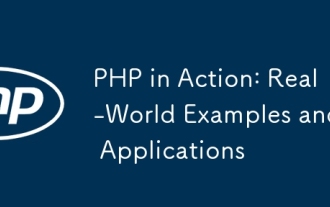
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
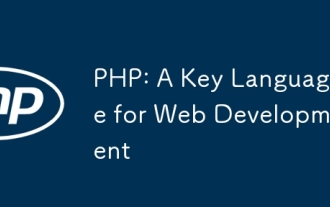
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
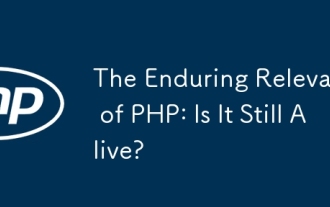
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
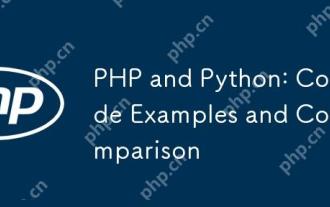
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
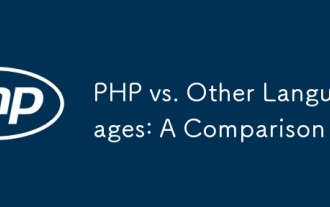
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
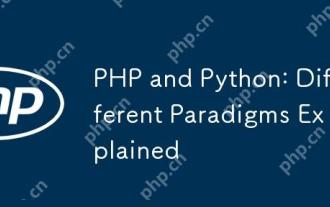
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
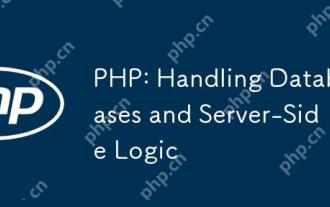
PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
