An in-depth analysis of the command pattern in PHP
In the previous article "Understanding the Prototype Mode in PHP" we introduced the prototype mode in PHP. This article will take you to understand the command mode in PHP.
Command mode, also known as action or transaction mode, many textbooks will use restaurants as an example. As customers, we are the order givers, the waiters are the recipients of this order, the menu is the actual order, and the chef is the executor of this order.
So, what does this model solve? When you want to modify the menu, you only need to tell the waiter and she will convey it to the chef. In other words, we have achieved the decoupling of customers and chefs. That is the decoupling of callers and implementers.
Of course, many design patterns can do this, but what the command pattern can do is to let a command receiver implement multiple commands (the waiter places an order, gets drinks, serves food), or puts a command Relayed to multiple implementers (hot cooks, cold cooks, main course chefs). This is where the command pattern really comes into play! !
Gof class diagram and explanation
GoF definition: Encapsulate a request as an object, so that you can use different requests to the customer Parameterization; queuing or logging requests, and supporting undoable operations
GoF Class Diagram
Code implementation
class Invoker { public $command; public function __construct($command) { $this->command = $command; } public function exec() { $this->command->execute(); } }
First we define a receiver of a command, or more appropriately, a requester of a command. The English definition of this word in the class diagram is "supplicant". That is, it initiates and operates commands.
abstract class Command { protected $receiver; public function __construct(Receiver $receiver) { $this->receiver = $receiver; } abstract public function execute(); } class ConcreteCommand extends Command { public function execute() { $this->receiver->action(); } }
The next step is the command, which is our "menu". The purpose of this command is to define who the real executor is.
class Receiver { public $name; public function __construct($name) { $this->name = $name; } public function action() { echo $this->name . '命令执行了!', PHP_EOL; } }
The taker, that is, the executor, is the person who actually executes the order.
// 准备执行者 $receiverA = new Receiver('A'); // 准备命令 $command = new ConcreteCommand($receiverA); // 请求者 $invoker = new Invoker($command); $invoker->exec();
To call the client, we need to contact the executor, that is, select a restaurant with a good chef (Receiver), and then prepare the order, which is the menu (Command), and finally hand it to the waiter (Invoker).
- In fact, the example of this restaurant is very clear. It is a perfect analysis of the command pattern.
- What about the promise that you can place multiple orders or give them to multiple chefs? Don’t worry, the following code helps us solve this problem
Full code: https://github.com/zhangyue0503/designpatterns-php/blob/master/09.command/source/ command.php
<?php class Invoker { private $command = []; public function setCommand(Command $command) { $this->command[] = $command; } public function exec() { if(count($this->command) > 0){ foreach ($this->command as $command) { $command->execute(); } } } public function undo() { if(count($this->command) > 0){ foreach ($this->command as $command) { $command->undo(); } } } } abstract class Command { protected $receiver; protected $state; protected $name; public function __construct(Receiver $receiver, $name) { $this->receiver = $receiver; $this->name = $name; } abstract public function execute(); } class ConcreteCommand extends Command { public function execute() { if (!$this->state || $this->state == 2) { $this->receiver->action(); $this->state = 1; } else { echo $this->name . '命令正在执行,无法再次执行了!', PHP_EOL; } } public function undo() { if ($this->state == 1) { $this->receiver->undo(); $this->state = 2; } else { echo $this->name . '命令未执行,无法撤销了!', PHP_EOL; } } } class Receiver { public $name; public function __construct($name) { $this->name = $name; } public function action() { echo $this->name . '命令执行了!', PHP_EOL; } public function undo() { echo $this->name . '命令撤销了!', PHP_EOL; } } // 准备执行者 $receiverA = new Receiver('A'); $receiverB = new Receiver('B'); $receiverC = new Receiver('C'); // 准备命令 $commandOne = new ConcreteCommand($receiverA, 'A'); $commandTwo = new ConcreteCommand($receiverA, 'B'); $commandThree = new ConcreteCommand($receiverA, 'C'); // 请求者 $invoker = new Invoker(); $invoker->setCommand($commandOne); $invoker->setCommand($commandTwo); $invoker->setCommand($commandThree); $invoker->exec(); $invoker->undo(); // 新加一个单独的执行者,只执行一个命令 $invokerA = new Invoker(); $invokerA->setCommand($commandOne); $invokerA->exec(); // 命令A已经执行了,再次执行全部的命令执行者,A命令的state判断无法生效 $invoker->exec();
- This time we solved the problem of multiple orders and multiple chefs at once, and also solved the problem of undoing the wrong order if it was placed
- It can be seen that the command mode decouples the object that calls the operation from the object that knows how to implement the operation.
- This implementation of multiple commands and multiple executors is a bit like the combination mode Implementation
- In this case, adding new commands will not affect the executor or the client. When a new client requires a new command, only the command and requester need to be added. Even if there is a need for modification, it is only the modification requester.
- In the event scheduling mechanism of the Laravel framework, in addition to the observer mode, the shadow of the command mode can also be clearly seen
Our mobile phone factories and restaurants are actually There is no difference. When we need a foundry to make a mobile phone, we also place an order first. This order can be regarded as an order. In this order, we will specify the accessories that need to be used, what type of CPU, what type of memory, what system is pre-installed, etc. Then the workers in the foundry will produce according to this order. In this process, I don’t have to worry about whether a certain worker or a group of workers executes the order. I only need to hand over the order to the person who interfaces with us, and then just wait for the mobile phone to be produced for acceptance! !
https://github.com/zhangyue0503/designpatterns-php/blob/master/09.command/source/command-up.php
Example
The SMS function is back. We found that in addition to the factory mode, the command mode seems to be a good way to implement it. Here, we are still using those SMS and push interfaces. Without further ado, let’s implement another one using command mode. Of course, interested friends can then implement our SMS withdrawal function. Think about how the above command cancellation is implemented.
SMS sending class diagram
https://github.com/zhangyue0503/designpatterns-php/blob/ master/09.command/source/command-message.php
<?php class SendMsg { private $command = []; public function setCommand(Command $command) { $this->command[] = $command; } public function send($msg) { foreach ($this->command as $command) { $command->execute($msg); } } } abstract class Command { protected $receiver = []; public function setReceiver($receiver) { $this->receiver[] = $receiver; } abstract public function execute($msg); } class SendAliYun extends Command { public function execute($msg) { foreach ($this->receiver as $receiver) { $receiver->action($msg); } } } class SendJiGuang extends Command { public function execute($msg) { foreach ($this->receiver as $receiver) { $receiver->action($msg); } } } class SendAliYunMsg { public function action($msg) { echo '【阿X云短信】发送:' . $msg, PHP_EOL; } } class SendAliYunPush { public function action($msg) { echo '【阿X云推送】发送:' . $msg, PHP_EOL; } } class SendJiGuangMsg { public function action($msg) { echo '【极X短信】发送:' . $msg, PHP_EOL; } } class SendJiGuangPush { public function action($msg) { echo '【极X推送】发送:' . $msg, PHP_EOL; } } $aliMsg = new SendAliYunMsg(); $aliPush = new SendAliYunPush(); $jgMsg = new SendJiGuangMsg(); $jgPush = new SendJiGuangPush(); $sendAliYun = new SendAliYun(); $sendAliYun->setReceiver($aliMsg); $sendAliYun->setReceiver($aliPush); $sendJiGuang = new SendJiGuang(); $sendAliYun->setReceiver($jgMsg); $sendAliYun->setReceiver($jgPush); $sendMsg = new SendMsg(); $sendMsg->setCommand($sendAliYun); $sendMsg->setCommand($sendJiGuang); $sendMsg->send('这次要搞个大活动,快来注册吧!!');
Description
- In this example, it is still a multi-command and multi-executor mode
- You can compare this example with the abstract factory. The same function is implemented using different design patterns, but please note What's more, the abstract factory is more about producing objects and returning objects, while the command mode is a choice of behavior
- We can see that the command mode is very suitable for forming a command queue. Multiple commands allow commands to be made one by one. Continue to execute
- It allows the receiving party to decide whether to veto the request, and the Receiver as the implementer has more say
Original address: https://juejin .cn/post/6844903950768930823
Author: Hardcore Project Manager
Recommended learning: "PHP Video Tutorial"
The above is the detailed content of An in-depth analysis of the command pattern in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










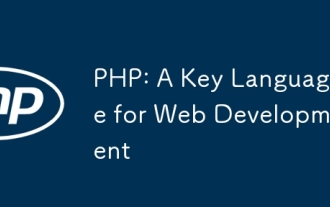
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
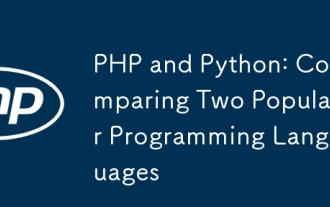
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
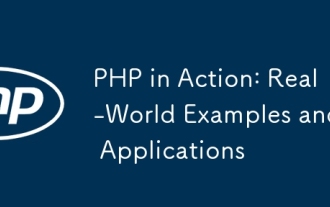
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
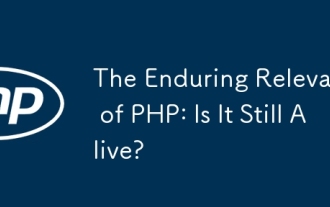
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
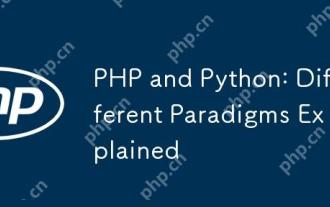
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
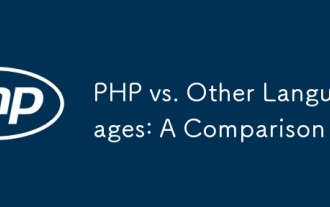
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
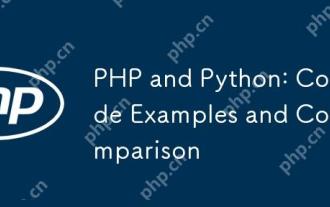
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
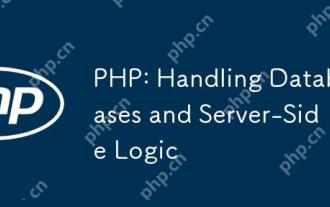
PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
