Python's powerful string formatting function-format
Since python2.6, a new function str.format() for formatting strings has been added, which is very powerful. So, what advantages does it have compared with the previous % formatted string? Let's unveil its shyness.
Syntax
It uses {} and: instead of %
Positional method formatting
>>> '{}.{}'.format('pythontab', 'com') 'pythontab.com' >>> '{}.{}.{}'.format('www', 'pythontab', 'com') 'www.pythontab.com' >>> '{1}.{2}'.format('www', 'pythontab', 'com') 'pythontab.com' >>> '{1}.{2} | {0}.{1}.{2}'.format('www', 'pythontab', 'com') 'pythontab.com | www.pythontab.com'
The format function of the string can accept unlimited parameters, the parameter positions can be out of order, and the parameters can be used or not. Multiple times, very flexible
Note: It cannot be empty {} under python2.6, but it can be used in python2.7 or above.
By keyword parameters
>>> '{domain}, {year}'.format(domain='www.pythontab.com', year=2016) 'www.pythontab.com, 2016' >>> '{domain} ### {year}'.format(domain='www.pythontab.com', year=2016) 'www.pythontab.com ### 2016' >>> '{domain} ### {year}'.format(year=2016,domain='www.pythontab.com') 'www.pythontab.com ### 2016'
By object properties
>>> class website: def __init__(self,name,type): self.name,self.type = name,type def __str__(self): return 'Website name: {self.name}, Website type: {self.type} '.format(self=self) >>> print str(website('pythontab.com', 'python')) Website name: pythontab.com, Website type: python >>> print website('pythontab.com', 'python') Website name: pythontab.com, Website type: python
By subscripts
>>> '{0[1]}.{0[0]}.{1}'.format(['pyhtontab', 'www'], 'com') 'www.pyhtontab.com'
With these convenient "mapping" methods, we have a lazy tool. Basic python knowledge tells us that lists and tuples can be "broken" into ordinary parameters for functions, while dict can be broken into keyword parameters for functions (through and *). So you can easily pass a list/tuple/dict to the format function, which is very flexible.
Format qualifiers
It has a wealth of "format qualifiers" (the syntax is {} with a symbol in it), such as:
Padding and alignment
Padding is often used together with alignment
^ , <、> are centered, left-aligned and right-aligned respectively, followed by width
: The padding character after the sign can only be one character. If not specified, it will be filled with spaces by default
Code example:
>>> '{:>10}'.format(2016) ' 2016' >>> '{:#>10}'.format(2016) '######2016' >>> '{:0>10}'.format(2016) '0000002016'
Number precision Precision is often used with type f
precision is often used together with type f
>>> '{:.2f}'.format(2016.0721) '2016.07'
where .2 represents the precision of length 2, and f represents float type.
Other types
are mainly based on base. b, d, o, and x are binary, decimal, octal, and hexadecimal respectively.
>>> '{:b}'.format(2016) '11111100000' >>> '{:d}'.format(2016) '2016' >>> '{:o}'.format(2016) '3740' >>> '{:x}'.format(2016) '7e0' >>>
Used, numbers can also be used as thousands separators for amounts.
>>> '{:,}'.format(20160721) '20,160,721'
format is so powerful and has many functions. You can try it out.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










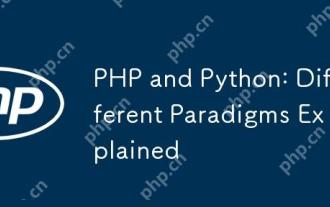
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
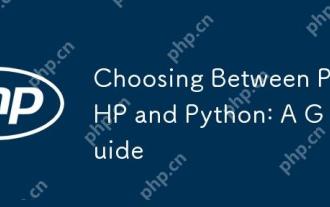
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
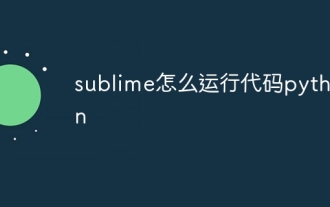
To run Python code in Sublime Text, you need to install the Python plug-in first, then create a .py file and write the code, and finally press Ctrl B to run the code, and the output will be displayed in the console.
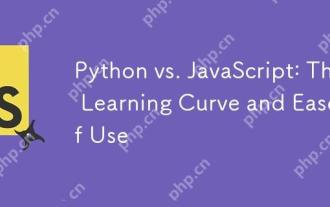
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
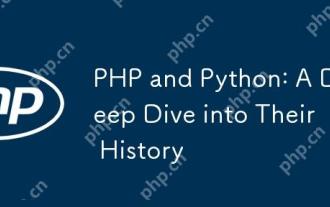
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
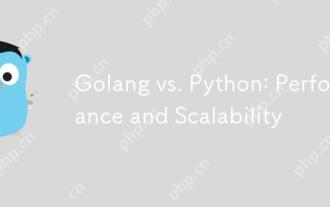
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
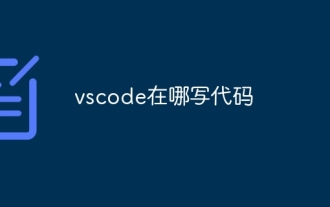
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
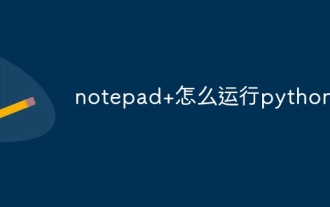
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
