import React from 'react';
import SQLiteStorage from 'react-native-sqlite-storage';
SQLiteStorage.DEBUG(true);
var database_name = "promo.db";
var database_version = "1.0";
var database_displayname = "MySQLite";
var database_size = -1;
var db;
const Product_TABLE_NAME = "Product";//收藏表
const SQLite = React.createClass({
render(){
return null;
},
componentWillUnmount(){
if(db){
this._successCB('close');
db.close();
}else {
console.log("SQLiteStorage not open");
}
},
open(){
db = SQLiteStorage.openDatabase(
database_name,
database_version,
database_displayname,
database_size,
()=>{
this._successCB('open');
},
(err)=>{
this._errorCB('open',err);
});
},
createTable(){
if (!db) {
open();
}
//创建表
db.transaction((tx)=> {
tx.executeSql('CREATE TABLE IF NOT EXISTS ' + Product_TABLE_NAME + '(' +
'id INTEGER PRIMARY KEY NOT NULL,' +
'name VARCHAR,' +
'jan VARCHAR,' +
'price VARCHAR,' +
'img VARCHAR,' +
'url VARCHAR,' +
'title VARCHAR'
+ ');'
, [], ()=> {
this._successCB('executeSql');
}, (err)=> {
this._errorCB('executeSql', err);
});
}, (err)=> {
this._errorCB('transaction', err);
}, ()=> {
this._successCB('transaction');
})
},
close(){
if(db){
this._successCB('close');
db.close();
}else {
console.log("SQLiteStorage not open");
}
db = null;
},
_successCB(name){
console.log("SQLiteStorage "+name+" success");
},
_errorCB(name, err){
console.log("SQLiteStorage "+name+" error:"+err);
}
});
module.exports = SQLite;
How can I configure the path of the database so that I can read the local sqlite.db on the mobile terminal without creating a new one every time?
No one answered and I answered it~
In the external component react-native-sqlite-storage, the source code supports reading and writing SD cards, so writing the path directly is ok
The location of the file on the mobile terminal is as shown in the picture:
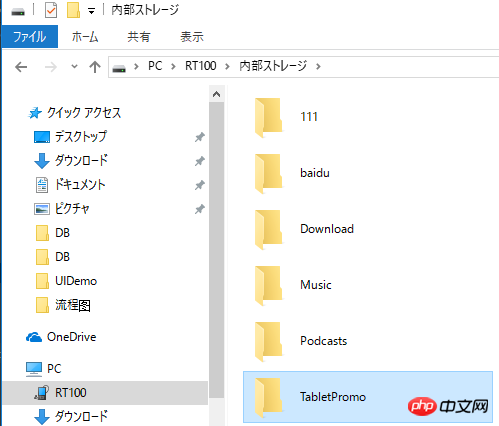
Continue to study how to dynamically read data, welcome to discuss