The official code is as follows. When the parent component changes the value in the input, the text of the span tag of the child component will change.
<p id="app">
<input v-model="parentMsg">
<br>
<child v-bind:my-message="parentMsg"></child>
</p>
<script>
Vue.component('child', {
props: ['myMessage'],
template: '<span>{{ myMessage }}</span><br>'
});
var vm = new Vue({
el: '#app',
data: {
parentMsg: 'hello you are a good boy!'
}
});
</script>
Browser effect
Next, I added the data attribute to the subcomponent, and changed the field bound in the <span> tag to {{msg}}. Now after inputting the value outside, the < in the subcomponent The text of the span> tag will not change. Why is this?
Vue.component('child', {
props: ['myMessage'],
template: '<span>{{ msg }}</span><br>',
data: function () {
return {
msg: this.myMessage
}
}
});
Browser effect
Arrays and objects in the data attribute can follow changes, but strings cannot. But if you use the computed attribute instead, the string can follow the changes.
May I ask all Vue masters, did I write the code wrong? Or for some reason.
You have misunderstood the meaning of the official vue document. Your approach can only let the msg in the data get the initial value of prop, and then the change of prop will not affect the msg. See this section of the document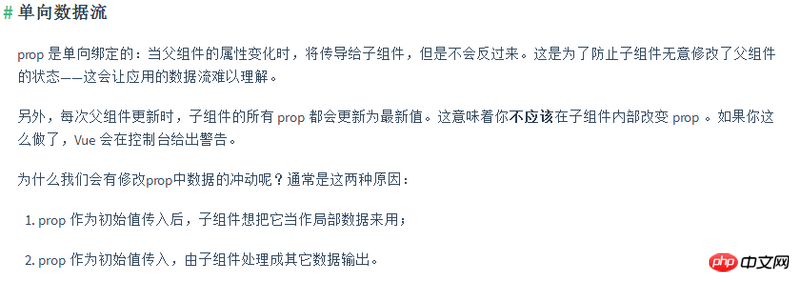
In your way of writing, msg gets the initial value from prop, which will be used as a local variable later. Modifying prop will not change the value of this local variable.
If you want to achieve the effect you want, just compute or $watch.
The parent component passes a msg to the child component
The child component assigns the msg parameter to the msg in its own data attribute
Then {{msg}} binds its own msg and not the parent component's.
It is equivalent to using The parent component’s msg initializes its own msg
Needs to be changed to {{myMessage}}
When you define an attribute with the same name in data, this causes a conflict, because through
Method, you can get both the props from the parent component and the data defined in the current component. This will cause confusion for Vue. Therefore, do not repeatedly define properties with the same name as props. If you want to use it, you can write like this
Then use defaultValue in the child component