RT,遇到一个奇怪的问题,我想要仪表盘在离开主页面的时候停止,回到主页面继续转,我现在做出来的只能一直转,离开页面了他也在转,跟吃了炫迈似的,根本停不下来,不知道是什么原因,麻烦大神们帮忙看看是什么原因
demo我放上网盘了仪表盘demo下载
代码如下
//
// TableViewController.m
// 仪表盘主页
//
// Created by liu on 15/5/29.
// Copyright (c) 2015年 fcar. All rights reserved.
//
#import "TableViewController.h"
#import "GagueCell.h"
static NSString * CELLID = @"cell";
@interface TableViewController ()
@property(nonatomic,assign)BOOL rota;
@end
@implementation TableViewController
- (void)viewDidLoad
{
[super viewDidLoad];
[self.tableView registerClass:[GagueCell class] forCellReuseIdentifier:CELLID];
self.tableView.bounces = NO;
self.rota = YES;
[self.tableView reloadRowsAtIndexPaths:@[[NSIndexPath indexPathForRow:0 inSection:0]] withRowAnimation:UITableViewRowAnimationFade];
NSLog(@"viewDidLoad");
}
-(void)viewWillAppear:(BOOL)animated
{
[super viewWillAppear:animated];
self.rota =YES;
[self.tableView reloadRowsAtIndexPaths:@[[NSIndexPath indexPathForRow:0 inSection:0]] withRowAnimation:UITableViewRowAnimationFade];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
// Return the number of rows in the section.
return 1;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
GagueCell*cell = [tableView dequeueReusableCellWithIdentifier:CELLID forIndexPath:indexPath];
cell.userInteractionEnabled = NO;
cell.isRefresh = self.rota;
NSLog(@"cellForRowAtIndexPath");
return cell;
}
-(CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
{
return (self.view.bounds.size.height-88-64)/3;
}
-(CGFloat)tableView:(UITableView *)tableView heightForFooterInSection:(NSInteger)section
{
return 0.1f;
}
-(CGFloat)tableView:(UITableView *)tableView heightForHeaderInSection:(NSInteger)section
{
return 100.f;
}
-(void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
NSLog(@"点击了屏幕");
NSLog(@"didSelectRowAtIndexPath");
}
//这里是storyboard创建的一个导航按钮事件
- (IBAction)nextVC:(UIBarButtonItem *)sender
{
self.rota = NO;
[self.tableView reloadRowsAtIndexPaths:@[[NSIndexPath indexPathForRow:0 inSection:0]] withRowAnimation:UITableViewRowAnimationFade];
[self performSegueWithIdentifier:@"next" sender:nil];
NSLog(@"nextVC");
}
@end
//接下来是仪表盘的cell
// GagueCell.m
// CarBaby
//
// Created by liu on 15/5/26.
// Copyright (c) 2015年 fcar. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface GagueCell : UITableViewCell
@property(nonatomic,assign)BOOL isRefresh;
@end
#import "GagueCell.h"
#import <QuartzCore/CADisplayLink.h>
#define WIDTH self.bounds.size.width
#define HEIGH self.bounds.size.height
@interface GagueCell ()
@property (strong, nonatomic)UIImageView *speed;//仪表盘图片
@property (strong, nonatomic)UISlider *speedSlider;//转盘转动的进度条
@property (strong, nonatomic)UIImageView * pin;//指针图片
@property(nonatomic,strong)UIView * view;//放着仪表盘的View
@property(nonatomic,strong)UILabel * speedLabel;
@property(nonatomic,strong)UILabel * consumption;
@property (nonatomic, strong) CADisplayLink *link;
@end
@implementation GagueCell
- (void)setSelected:(BOOL)selected animated:(BOOL)animated {
[super setSelected:selected animated:animated];
// Configure the view for the selected state
}
-(void)start
{
if (self.link) return;
CADisplayLink *link = [CADisplayLink displayLinkWithTarget:self selector:@selector(update)];
[link addToRunLoop:[NSRunLoop mainRunLoop] forMode:NSRunLoopCommonModes];
self.link = link;
NSLog(@"start");
}
- (void)stopRotating
{
[self.link invalidate];
self.link = nil;
NSLog(@"stopRotating");
}
- (void)update
{
if (self.speedSlider.value >= 30 )
{
self.speedSlider.value ++;
}
if(self.speedSlider.value>=330)
{
self.speedSlider.value = 0;
}
NSLog(@"转盘还在转");
CGFloat angle = self.speedSlider.value/180*M_PI;
[UIView animateWithDuration:1 animations:^{
self.pin.transform = CGAffineTransformMakeRotation(angle);
}];
self.consumption.text = [NSString stringWithFormat:@"当前油耗:%.1f L",3.0];
self.speedLabel.text = [NSString stringWithFormat:@"%@ km/h",@(self.speedSlider.value-30)];
}
-(instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier
{
if (self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]) {
self.highlighted = NO;
self.view = [[UIView alloc] initWithFrame:CGRectZero];
[self addSubview:self.view];
self.speed = [[UIImageView alloc] initWithFrame:CGRectMake(0, 0, 320,10)];
self.pin = [[UIImageView alloc] initWithFrame:CGRectMake(0, 0, 320,10)];
self.pin.image = [UIImage imageNamed:@"pin"];
self.speed.image = [UIImage imageNamed:@"speed"];
self.speedSlider = [[UISlider alloc] initWithFrame:CGRectZero];
[self.speedSlider addTarget:self action:@selector(rotaSliderChange:) forControlEvents:UIControlEventValueChanged];
self.speedSlider.maximumValue = 330;
self.speedSlider.minimumValue = 30;
self.speedSlider.value = 30;
self.speedSlider.hidden = YES;
self.backgroundView = nil;
self.backgroundColor = nil;
self.selectionStyle = UITableViewCellSelectionStyleNone;
self.speedLabel = [[UILabel alloc] initWithFrame:CGRectZero];
self.consumption = [[UILabel alloc] initWithFrame:CGRectZero];
self.speedLabel.textAlignment = NSTextAlignmentCenter;
self.consumption.textAlignment = NSTextAlignmentCenter;
self.speedLabel.textColor = [UIColor whiteColor];
self.consumption.textColor = [UIColor whiteColor];
[self.view addSubview:self.speed];
[self.view addSubview:self.pin];
[self addSubview:self.speedSlider];
[self addSubview:self.speedLabel];
[self addSubview:self.consumption];
self.view.userInteractionEnabled = NO;
self.speed.userInteractionEnabled = NO;
self.pin.userInteractionEnabled = NO;
}
return self;
}
- (void)rotaSliderChange:(UISlider *)sender
{
}
-(void)layoutSubviews
{
[super layoutSubviews];
CGFloat num =WIDTH>HEIGH?HEIGH:WIDTH;
//CGPoint center = self.center;
self.view.frame =CGRectMake((WIDTH-num)/2, 0, num,num);
self.view.backgroundColor = [UIColor clearColor];
self.speed.center = CGPointMake(num/2, num/2);
self.pin.center = CGPointMake(num/2, num/2);
self.speed.bounds = self.view.bounds;
self.pin.bounds = self.view.bounds;
NSLog(@"layoutSubviews");
self.speedSlider.frame = CGRectMake(0,0, 320, 30);
self.speedLabel.frame = CGRectMake((WIDTH-num)/2, num-70, num, 25);
self.consumption.frame = CGRectMake((WIDTH-num)/2, num-25, num, 25);
self.consumption.text = [NSString stringWithFormat:@"当前油耗:%.1f L",0.f];
self.speedLabel.text = [NSString stringWithFormat:@"%@ km/h",@(0)];
self.consumption.text = [NSString stringWithFormat:@"当前油耗:%.1f L",3.0];
self.speedLabel.text = [NSString stringWithFormat:@"%@ km/h",@(self.speedSlider.value-30)];
}
-(void)setIsRefresh:(BOOL)isRefresh
{
_isRefresh = isRefresh;
NSLog(@"setIsRefresh");
if (self.isRefresh)
{
[self start];
}
else
{
[self stopRotating];
}
}
@end
界面跳转就是跳转到一个空白页面
Reloading a row causes the table view to ask its data source for a new cell for that row.
Add these 2 lines and you will find that the cell is no longer the same. You have lost control of the original cell and the original displayer. If you NSLog() you will find that the displayer is null
So the correct approach is:
There is a problem with your settings when entering the next page. You just use self.rota. It is completely useless to cell.isRefresh.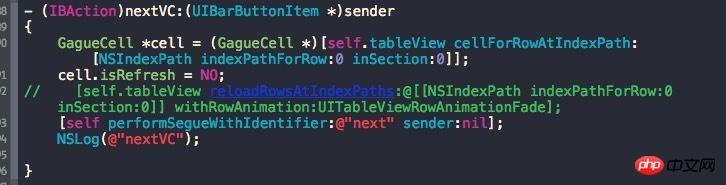
And you refreshed the cell again...
Look at the picture