First of all, point out a problem: construction BigDecimal 的时候,尽量不要使用浮点数(double,float), because there is a lack of precision in the storage of floating point numbers in computers. For example, the code you wrote:
public static void main(String[] args) throws Exception {
double a = 1.505;
BigDecimal bd = new BigDecimal(a);
System.out.println("bd: " + bd.toString());
}
Run results:
You can see that the floating point number 1.505 cannot be stored in the computer - if you don’t know the specific reason, please search for it yourself "The problem of missing precision of floating point numbers"
Second, if you need to output 1.51, which is often called "rounding", then you need to specify the rounding mode of DecimalFormat:
public static void main(String[] args) throws Exception {
BigDecimal bd = new BigDecimal("1.505");
System.out.println("bd: " + bd.toString());
DecimalFormat df = new DecimalFormat("#.00");
df.setRoundingMode(RoundingMode.HALF_UP);
System.out.println("format: " + df.format(bd));
}
Run results:
If you don’t understand the usage of RoundingMode, please search for "Usage of RoundingMode"
Third, regarding the meaning of the DecimalFormat strings in brackets, please search "Usage of DecimalFormat"
First of all, point out a problem: construction
BigDecimal
的时候,尽量不要使用浮点数(double
,float
), because there is a lack of precision in the storage of floating point numbers in computers. For example, the code you wrote:Run results:

You can see that the floating point number 1.505 cannot be stored in the computer - if you don’t know the specific reason, please search for it yourself "The problem of missing precision of floating point numbers"
Second, if you need to output 1.51, which is often called "rounding", then you need to specify the rounding mode of
DecimalFormat
:Run results:
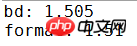
If you don’t understand the usage of
RoundingMode
, please search for "Usage of RoundingMode"Third, regarding the meaning of the
DecimalFormat
strings in brackets, please search "Usage of DecimalFormat"