The count in the DOM is two-way bound to the A Controller, and the count value in the A Controller is taken from the Service. The B Controller can change the value in the Service.
Please tell me how to realize that after B changes the value in Service, the DOM can also change in real time
As for the situation mentioned by the poster, let me be more careful. It can be discussed in the following two situations:
The value shared by service is an object
At this time, you only need to assign the value to the scope normally, because the object is passed by reference, see the example:
html code:
service code:
controller code:
Page effect:
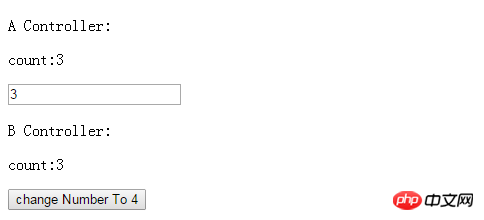

After clicking the change Number To 4 button, the page effect is:
You can see that the data is updated synchronously. Why can such an effect occur? There are two very important reasons:
One thing to note is that any change in scope or service will cause the other to change!
The value shared by service is the basic type
When the shared value is a basic type, the above method cannot be achieved. Because assignment of basic types passes values, not references. At this time, the interaction between the two controllers must be event-driven. Look at the code:
html code:
service code:
controller code:
The page effect is the same as the first one.
$emit and $broadcast between peers cannot pass events, and events are broadcast downwards on the rootScope.
That’s all. It involves a lot of things.
hope help you!
You need to look at the difference between service and factory. One is constructed and the other is singleton. If you use factory, processing in B will affect A. Or you use broadcasting, Angular has a function for broadcasting.
I understand what you want to ask is, how to synchronize DOM with Scope data?
In fact, {{count}} is a monitoring function, which is equivalent to calling
$scope.$watch('count',watchFunc)
Angular detects whether $scope.count changes every time it is updated, and triggers watchFunc once it changes
Specific to your DOM, watchFunc updates textContent
As for when angular will be updated, after ng-click, or $http.get or input onblur,
I recently looked at angular and imitated it. There is a chapter in the code base that explains how to implement binding