MongoDB is a product between a relational database and a non-relational database. It is the most feature-rich among non-relational databases and is most like a relational database. The data structure it supports is very loose and is a bson format similar to json, so it can store more complex data types. The biggest feature of Mongo is that the query language it supports is very powerful. Its syntax is somewhat similar to an object-oriented query language. It can almost implement most functions similar to single-table queries in relational databases, and it also supports indexing of data.
<?php namespace League; use League\Monga\Connection; use MongoBinData; use MongoCode; use MongoConnectionException; use MongoDate; use MongoId; use MongoRegex; class Monga { public static function data($data, $type = null) { $type === null && $type = MongoBinData::BYTE_ARRAY; return new MongoBinData($data, $type); } public static function id($id) { return new MongoId($id); } public static function code($code, array $scope = []) { return new MongoCode($code, $scope); } public static function date($sec = null, $usec = 0) { $sec === null && $sec = time(); return new MongoDate($sec, $usec); } public static function regex($regex) { return new MongoRegex($regex); } public static function connection($server = null, array $options = [], array $driverOptions = []) { return new Connection($server, $options, $driverOptions); } }
All resources on this site are contributed by netizens or reprinted by major download sites. Please check the integrity of the software yourself! All resources on this site are for learning reference only. Please do not use them for commercial purposes. Otherwise, you will be responsible for all consequences! If there is any infringement, please contact us to delete it. Contact information: admin@php.cn
Related Article
30 Sep 2016
Looking for a php/python library management program (similar to Baidu library, managing doc/pdf and other libraries)~~ It mainly needs to have search functions, especially file classification retrieval/file tag retrieval functions, no need for online conversion, online browsing!
08 Aug 2016
:This article mainly introduces the database abstraction layer function library for mysql. Students who are interested in PHP tutorials can refer to it.
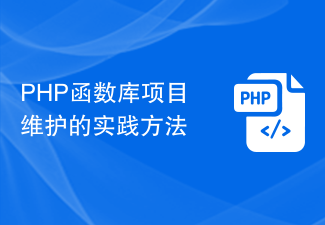
15 Jun 2023
In development projects, the use of PHP function libraries is very extensive. With the continuous maintenance of the project, the maintenance and management of PHP function libraries have become more and more important. This article will introduce some practical methods of PHP function library maintenance to help project developers better manage and maintain function libraries. 1. Standardized naming Standardized naming of each function in the function library can make the function library easier to use and manage. When naming functions, try to use meaningful words and follow the following conventions: 1. Function names should use lowercase letters and the following
21 Jul 2016
A database abstraction layer function library for mysql. ?php // // SourceForge: Breaking Down the Barriers to Open Source Development // Copyright 1999-2000 (c) The SourceForge Crew // http://sourceforge.net // // $Id: database.php,v 1.6
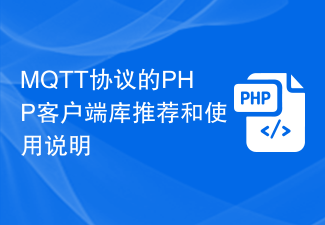
09 Jul 2023
PHP client library recommendations and usage instructions for the MQTT protocol MQTT (MessageQueuingTelemetryTransport) is a lightweight message transmission protocol that is widely used in fields such as the Internet of Things and sensor networks. In PHP development, in order to facilitate communication with the MQTT server using the MQTT protocol, we can choose to use some PHP client libraries to simplify this process. In this article, we will recommend several commonly used PHP client libraries and provide usage instructions.
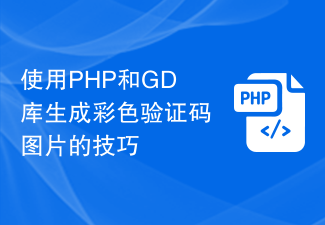
14 Jul 2023
Tips for generating colorful verification code images using PHP and GD libraries Introduction: Verification code is a common network security technology. By requiring users to enter a verification code when logging in, registering, or submitting a form, you can effectively prevent automated attacks from robots and malicious programs. This article will introduce the techniques of using PHP and GD libraries to generate colorful verification code images, helping developers to add a certain degree of recognizability and artistry when creating verification codes. 1. Environment preparation Before starting, make sure that PHP and GD libraries have been installed in your development environment. Can


Hot Tools

PHP library for dependency injection containers
PHP library for dependency injection containers
A collection of 50 excellent classic PHP algorithms
Classic PHP algorithm, learn excellent ideas and expand your thinking
Small PHP library for optimizing images
Small PHP library for optimizing images
