Cocos2d-x3.1中ClippingNode的使用实例
#include 2d/CCNode.h//用到节点头文件和OPENGL深度缓冲定义头文件#include CCGL.h#include renderer/CCGroupCommand.h//用到GroupCommand和CustomCommand渲染#include renderer/CCCustomCommand.hNS_CC_BEGIN/** ClippingNode is a subclass of Node. It dr
#include "2d/CCNode.h"//用到节点头文件和OPENGL深度缓冲定义头文件 #include "CCGL.h" #include "renderer/CCGroupCommand.h"//用到GroupCommand和CustomCommand渲染 #include "renderer/CCCustomCommand.h" NS_CC_BEGIN /** ClippingNode is a subclass of Node. It draws its content (childs) clipped using a stencil. The stencil is an other Node that will not be drawn. The clipping is done using the alpha part of the stencil (adjusted with an alphaThreshold). */ class CC_DLL ClippingNode : public Node { public: /** Creates and initializes a clipping node without a stencil. */ static ClippingNode* create();//静态创建对象 /** Creates and initializes a clipping node with an other node as its stencil. The stencil node will be retained. */ static ClippingNode* create(Node *stencil);//用模板创建裁剪节点 /** The Node to use as a stencil to do the clipping. The stencil node will be retained. This default to nil. */ Node* getStencil() const;//获取节点模板 void setStencil(Node *stencil);//设置节点模板 /** The alpha threshold. The content is drawn only where the stencil have pixel with alpha greater than the alphaThreshold. Should be a float between 0 and 1. This default to 1 (so alpha test is disabled). *///ALPHA测试参考值,用于进行ALPHA测试比较,一般比较算法为小于此值的像素会直接被舍弃,这样就实现了图像的镂空 GLfloat getAlphaThreshold() const;//获取ALPHT的测试参考值 void setAlphaThreshold(GLfloat alphaThreshold);//设置ALPHA的测试参考值 /** Inverted. If this is set to true, the stencil is inverted, so the content is drawn where the stencil is NOT drawn. This default to false. */ bool isInverted() const;//获取遮罩运算是否取反设置 void setInverted(bool inverted);//设置遮罩运算取反 // Overrides//继承的函数 /** * @js NA * @lua NA */ virtual void onEnter() override; /** * @js NA * @lua NA */ virtual void onEnterTransitionDidFinish() override; /** * @js NA * @lua NA */ virtual void onExitTransitionDidStart() override; /** * @js NA * @lua NA */ virtual void onExit() override; virtual void visit(Renderer *renderer, const Mat4 &parentTransform, bool parentTransformUpdated) override; CC_CONSTRUCTOR_ACCESS: ClippingNode(); /** * @js NA * @lua NA */ virtual ~ClippingNode(); /** Initializes a clipping node without a stencil. */ virtual bool init(); /** Initializes a clipping node with an other node as its stencil. The stencil node will be retained, and its parent will be set to this clipping node. */ virtual bool init(Node *stencil); protected: /**draw fullscreen quad to clear stencil bits */ void drawFullScreenQuadClearStencil(); Node* _stencil; GLfloat _alphaThreshold; bool _inverted; //renderData and callback void onBeforeVisit(); void onAfterDrawStencil(); void onAfterVisit(); GLboolean _currentStencilEnabled; GLuint _currentStencilWriteMask; GLenum _currentStencilFunc; GLint _currentStencilRef; GLuint _currentStencilValueMask; GLenum _currentStencilFail; GLenum _currentStencilPassDepthFail; GLenum _currentStencilPassDepthPass; GLboolean _currentDepthWriteMask; GLboolean _currentAlphaTestEnabled; GLenum _currentAlphaTestFunc; GLclampf _currentAlphaTestRef; GLint _mask_layer_le; GroupCommand _groupCommand; CustomCommand _beforeVisitCmd; CustomCommand _afterDrawStencilCmd; CustomCommand _afterVisitCmd; private: CC_DISALLOW_COPY_AND_ASSIGN(ClippingNode); }; NS_CC_END
3、使用实例
<pre name="code" class="cpp">class NewClippingNode : public Layer { public: CREATE_FUNC(NewClippingNode); virtual bool init(); };
boolNewClippingNode::init()
{
auto s =Director::getInstance()->getWinSize();
bool bRet = false;
do{
CC_BREAK_IF(!Layer::init());
auto clipper = ClippingNode::create();
clipper->setTag(kTagClipperNode);
clipper->setContentSize(Size(200,200));//设置剪裁区域大小
clipper->ignoreAnchorPointForPosition(false);
clipper->setAnchorPoint(Vec2(0.5,0.5));//设置锚点
clipper->setPosition(Vec2(s.width/2, s.height/2));
this->addChild(clipper);
clipper->setAlphaThreshold(0.05f);//设置透明度的阈值ALPHA值
//设置剪裁模板
auto stencil = Sprite::create("grossini.png");
stencil->setScale(2);
stencil->setPosition(Vec2(s.width/2, s.height/2));
clipper->setStencil(stencil);//设置剪裁模板
//TODO Fix draw node as clip node
// auto stencil = NewDrawNode::create();
// Vec2 rectangle[4];
// rectangle[0] = Vec2(0, 0);
// rectangle[1] = Vec2(clipper->getContentSize().width, 0);
// rectangle[2] = Vec2(clipper->getContentSize().width, clipper->getContentSize().height);
// rectangle[3] = Vec2(0, clipper->getContentSize().height);
//
// Color4F white(1, 1, 1, 1);
// stencil->drawPolygon(rectangle, 4, white, 1, white);
// clipper->setStencil(stencil);
//设置剪裁节点内容
auto content = Sprite::create("background2.png");
content->setTag(kTagContentNode);
content->ignoreAnchorPointForPosition(false);
content->setAnchorPoint(Vec2(0.5,0.5));
content->setPosition(Vec2(s.width/2,200));
clipper->addChild(content);
bRet =true;
}while(0);
return bRet;
}

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
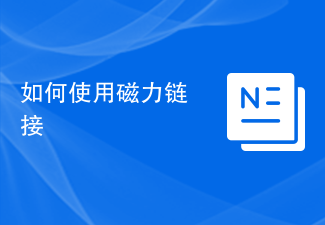
磁力連結是一種用於下載資源的連結方式,相較於傳統的下載方式更為便利和有效率。使用磁力連結可以透過點對點的方式下載資源,而不需要依賴中介伺服器。本文將介紹磁力連結的使用方法及注意事項。一、什麼是磁力連結磁力連結是一種基於P2P(Peer-to-Peer)協定的下載方式。透過磁力鏈接,使用者可以直接連接到資源的發布者,從而完成資源的共享和下載。與傳統的下載方式相比,磁
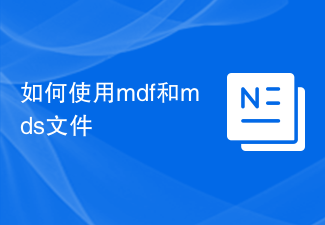
mdf檔案和mds檔案怎麼用隨著電腦科技的不斷進步,我們可以透過多種方式來儲存和共享資料。在數位媒體領域,我們經常會遇到一些特殊的文件格式。在這篇文章中,我們將討論一種常見的文件格式—mdf和mds文件,並介紹它們的使用方法。首先,我們需要了解mdf檔案和mds檔案的含義。 mdf是CD/DVD鏡像檔的副檔名,而mds檔則是mdf檔的元資料檔。
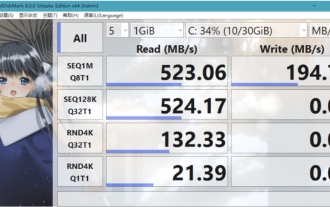
CrystalDiskMark是一款適用於硬碟的小型HDD基準測試工具,可快速測量順序和隨機讀取/寫入速度。接下來就讓小編為大家介紹一下CrystalDiskMark,以及crystaldiskmark如何使用吧~一、CrystalDiskMark介紹CrystalDiskMark是一款廣泛使用的磁碟效能測試工具,用於評估機械硬碟和固態硬碟(SSD)的讀取和寫入速度和隨機I/O性能。它是一款免費的Windows應用程序,並提供用戶友好的介面和各種測試模式來評估硬碟效能的不同方面,並被廣泛用於硬體評
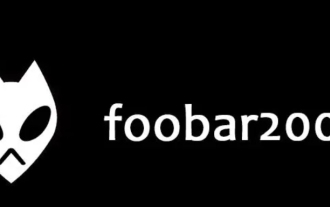
foobar2000是一款能隨時收聽音樂資源的軟體,各種音樂無損音質帶給你,增強版本的音樂播放器,讓你得到更全更舒適的音樂體驗,它的設計理念是將電腦端的高級音頻播放器移植到手機上,提供更便捷高效的音樂播放體驗,介面設計簡潔明了易於使用它採用了極簡的設計風格,沒有過多的裝飾和繁瑣的操作能夠快速上手,同時還支持多種皮膚和主題,根據自己的喜好進行個性化設置,打造專屬的音樂播放器支援多種音訊格式的播放,它還支援音訊增益功能根據自己的聽力情況調整音量大小,避免過大的音量對聽力造成損害。接下來就讓小編為大
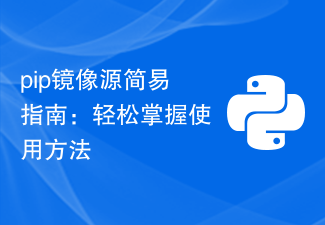
輕鬆上手:如何使用pip鏡像來源隨著Python在全球的普及,pip成為了Python套件管理的標準工具。然而,許多開發者在使用pip安裝套件時面臨的常見問題是速度慢。這是因為預設情況下,pip從Python官方來源或其他外部來源下載包,而這些來源可能位於海外伺服器,導致下載速度緩慢。為了提高下載速度,我們可以使用pip鏡像來源。什麼是pip鏡像來源?簡單來說,就
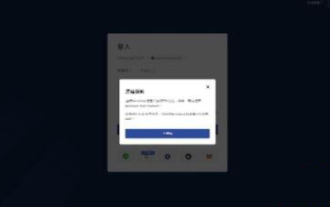
MetaMask(中文也叫小狐狸錢包)是一款免費的、廣受好評的加密錢包軟體。目前,BTCC已支援綁定MetaMask錢包,綁定後可使用MetaMask錢包進行快速登錄,儲值、買幣等,且首次綁定還可獲得20USDT體驗金。在BTCCMetaMask錢包教學中,我們將詳細介紹如何註冊和使用MetaMask,以及如何在BTCC綁定並使用小狐狸錢包。 MetaMask錢包是什麼? MetaMask小狐狸錢包擁有超過3,000萬用戶,是當今最受歡迎的加密貨幣錢包之一。它可免費使用,可作為擴充功能安裝在網絡
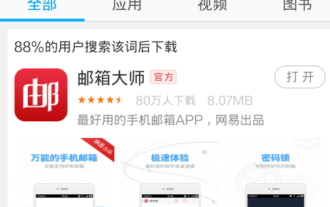
網易郵箱,作為中國網友廣泛使用的一種電子郵箱,一直以來以其穩定、高效的服務贏得了用戶的信賴。而網易信箱大師,則是專為手機使用者打造的信箱軟體,它大大簡化了郵件的收發流程,讓我們的郵件處理變得更加便利。那麼網易信箱大師該如何使用,具體又有哪些功能呢,下文中本站小編將為大家帶來詳細的內容介紹,希望能幫助到大家!首先,您可以在手機應用程式商店搜尋並下載網易信箱大師應用程式。在應用寶或百度手機助手中搜尋“網易郵箱大師”,然後按照提示進行安裝即可。下載安裝完成後,我們打開網易郵箱帳號並進行登錄,登入介面如下圖所示
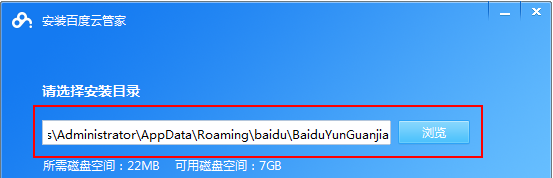
在如今雲端儲存已成為我們日常生活和工作中不可或缺的一部分。百度網盤作為國內領先的雲端儲存服務之一,憑藉其強大的儲存功能、高效的傳輸速度以及便捷的操作體驗,贏得了廣大用戶的青睞。而且無論你是想要備份重要文件、分享資料,還是在線上觀看影片、聽取音樂,百度網盤都能滿足你的需求。但很多用戶可能對百度網盤app的具體使用方法還不了解,那麼這篇教學就將為大家詳細介紹百度網盤app如何使用,還有疑惑的用戶們就快來跟著本文詳細了解一下吧!百度雲網盤怎麼用:一、安裝首先,下載並安裝百度雲軟體時,請選擇自訂安裝選
