Simple iPhone Keychain Access
The keychain is about the only place that an iPhone application can safely store data that will be preserved across a re-installation of the application. Each iPhone application gets its own set of keychain items which are backed up whenev
The keychain is about the only place that an iPhone application can safely store data that will be preserved across a re-installation of the application. Each iPhone application gets its own set of keychain items which are backed up whenever the user backs up the device via iTunes. The backup data is encrypted as part of the backup so that it remains secure even if somebody gets access to the backup data. This makes it very attractive to store sensitive data such as passwords, license keys, etc.
The only problem is that accessing the keychain services is complicated and even the GenericKeychain example code is hard to follow. I hate to include cut and pasted code into my application, especially when I do not understand it. Instead I have gone back to basics to build up a simple iPhone keychain access example that does just what I want and not much more.
In fact all I really want to be able to do is securely store a password string for my application and be able to retrieve it a later date.
Getting Started
A couple of housekeeping items to get started:
- Add the “Security.framework” framework to your iPhone application
- Include the header file
Note that the security framework is a good old fashioned C framework so no Objective-C style methods calls. Also it will only work on the device not in in the iPhone Simulator.
The Basic Search Dictionary
All of the calls to the keychain services make use of a dictionary to define the attributes of the keychain item you want to find, create, update or delete. So the first thing we will do is define a function to allocate and construct this dictionary for us:
<code>static NSString *serviceName = @"com.mycompany.myAppServiceName"; - (NSMutableDictionary *)newSearchDictionary:(NSString *)identifier { NSMutableDictionary *searchDictionary = [[NSMutableDictionary alloc] init]; [searchDictionary setObject:(id)kSecClassGenericPassword forKey:(id)kSecClass]; NSData *encodedIdentifier = [identifier dataUsingEncoding:NSUTF8StringEncoding]; [searchDictionary setObject:encodedIdentifier forKey:(id)kSecAttrGeneric]; [searchDictionary setObject:encodedIdentifier forKey:(id)kSecAttrAccount]; [searchDictionary setObject:serviceName forKey:(id)kSecAttrService]; return searchDictionary; } </code>
The dictionary contains three items. The first with key kSecClass defines the class of the keychain item we will be dealing with. I want to store a password in the keychain so I use the value kSecClassGenericPassword for the value.
The second item in the dictionary with key kSecAttrGeneric is what we will use to identify the keychain item. It can be any value we choose such as “Password” or “LicenseKey”, etc. To be clear this is not the actual value of the password just a label we will attach to this keychain item so we can find it later. In theory our application could store a number of passwords in the keychain so we need to have a way to identify this particular one from the others. The identifier has to be encoded before being added to the dictionary
The combination of the final two attributes kSecAttrAccount and kSecAttrService should be set to something unique for this keychain. In this example I set the service name to a static string and reuse the identifier as the account name.
You can use multiple attributes for a given class of item. Some of the other attributes that we could also use for the kSecClassGenericPassword item include an account name, description, etc. However by using just a single attribute we can simplify the rest of the code.
Searching the keychain
To find out if our password already exists in the keychain (and what the value of the password is) we use the SecItemCopyMatching function. But first we add a couple of extra items to our basic search dictionary:
<code>- (NSData *)searchKeychainCopyMatching:(NSString *)identifier { NSMutableDictionary *searchDictionary = [self newSearchDictionary:identifier]; // Add search attributes [searchDictionary setObject:(id)kSecMatchLimitOne forKey:(id)kSecMatchLimit]; // Add search return types [searchDictionary setObject:(id)kCFBooleanTrue forKey:(id)kSecReturnData]; NSData *result = nil; OSStatus status = SecItemCopyMatching((CFDictionaryRef)searchDictionary, (CFTypeRef *)&result); [searchDictionary release]; return result; } </code>
The first attribute we add to the dictionary is to limit the number of search results that get returned. We are looking for a single entry so we set the attribute kSecMatchLimit to kSecMatchLimitOne.
The next attribute determines how the result is returned. Since in our simple case we are expecting only a single attribute to be returned (the password) we can set the attribute kSecReturnData to kCFBooleanTrue. This means we will get an NSData reference back that we can access directly.
If we were storing and searching for a keychain item with multiple attributes (for example if we were storing an account name and password in the same keychain item) we would need to add the attribute kSecReturnAttributes and the result would be a dictionary of attributes.
Now with the search dictionary set up we call the SecItemCopyMatching function and if our item exists in the keychain the value of the password is returned to in the NSData block. To get the actual decoded string you could do something like:
<code> NSData *passwordData = [self searchKeychainCopyMatching:@"Password"]; if (passwordData) { NSString *password = [[NSString alloc] initWithData:passwordData encoding:NSUTF8StringEncoding]; [passwordData release]; } </code>
Creating an item in the keychain
Adding an item is almost the same as the previous examples except that we need to set the value of the password we want to store.
<code>- (BOOL)createKeychainValue:(NSString *)password forIdentifier:(NSString *)identifier { NSMutableDictionary *dictionary = [self newSearchDictionary:identifier]; NSData *passwordData = [password dataUsingEncoding:NSUTF8StringEncoding]; [dictionary setObject:passwordData forKey:(id)kSecValueData]; OSStatus status = SecItemAdd((CFDictionaryRef)dictionary, NULL); [dictionary release]; if (status == errSecSuccess) { return YES; } return NO; } </code>
To set the value of the password we add the attribute kSecValueData to our search dictionary making sure we encode the string and then call SecItemAdd passing the dictionary as the first argument. If the item already exists in the keychain this will fail.
Updating a keychain item
Updating a keychain is similar to adding an item except that a separate dictionary is used to contain the attributes to be updated. Since in our case we are only updating a single attribute (the password) this is easy:
<code>- (BOOL)updateKeychainValue:(NSString *)password forIdentifier:(NSString *)identifier { NSMutableDictionary *searchDictionary = [self newSearchDictionary:identifier]; NSMutableDictionary *updateDictionary = [[NSMutableDictionary alloc] init]; NSData *passwordData = [password dataUsingEncoding:NSUTF8StringEncoding]; [updateDictionary setObject:passwordData forKey:(id)kSecValueData]; OSStatus status = SecItemUpdate((CFDictionaryRef)searchDictionary, (CFDictionaryRef)updateDictionary); [searchDictionary release]; [updateDictionary release]; if (status == errSecSuccess) { return YES; } return NO; } </code>
Deleting an item from the keychain
The final (and easiest) operation is to delete an item from the keychain using the SecItemDelete function and our usual search dictionary:
<code>- (void)deleteKeychainValue:(NSString *)identifier { NSMutableDictionary *searchDictionary = [self newSearchDictionary:identifier]; SecItemDelete((CFDictionaryRef)searchDictionary); [searchDictionary release]; } </code>

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
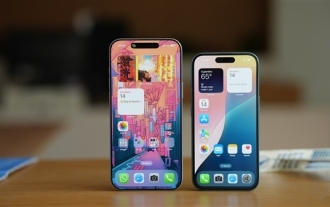
苹果iPhone17或将迎来重大升级,以应对国内华为、小米等强劲竞争对手的冲击。据数码博主@数码闲聊站爆料,iPhone17标准版有望首次搭载高刷新率屏幕,显著提升用户体验。此举标志着苹果历经五年,终于将高刷新率技术下放至标准版机型。目前,iPhone16作为6000元价位段唯一一款配备60Hz屏幕的旗舰手机,显得有些落后。虽然iPhone17标准版将拥有高刷新率屏幕,但与Pro版相比仍存在差异,例如边框设计仍未达到Pro版的超窄边框效果。更值得关注的是,iPhone17Pro系列将采用全新、更
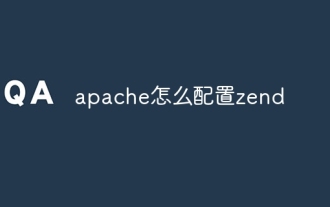
如何在 Apache 中配置 Zend?在 Apache Web 服務器中配置 Zend Framework 的步驟如下:安裝 Zend Framework 並解壓到 Web 服務器目錄中。創建 .htaccess 文件。創建 Zend 應用程序目錄並添加 index.php 文件。配置 Zend 應用程序(application.ini)。重新啟動 Apache Web 服務器。
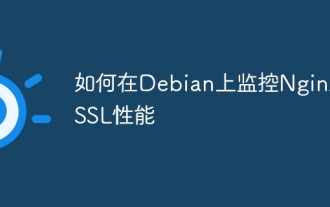
本文介紹如何在Debian系統上有效監控Nginx服務器的SSL性能。我們將使用NginxExporter將Nginx狀態數據導出到Prometheus,再通過Grafana進行可視化展示。第一步:配置Nginx首先,我們需要在Nginx配置文件中啟用stub_status模塊來獲取Nginx的狀態信息。在你的Nginx配置文件(通常位於/etc/nginx/nginx.conf或其包含文件中)中添加以下代碼段:location/nginx_status{stub_status
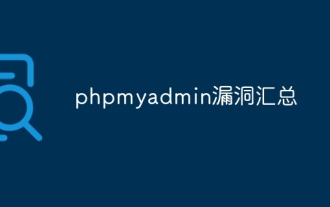
PHPMyAdmin安全防禦策略的關鍵在於:1. 使用最新版PHPMyAdmin及定期更新PHP和MySQL;2. 嚴格控制訪問權限,使用.htaccess或Web服務器訪問控制;3. 啟用強密碼和雙因素認證;4. 定期備份數據庫;5. 仔細檢查配置文件,避免暴露敏感信息;6. 使用Web應用防火牆(WAF);7. 進行安全審計。 這些措施能夠有效降低PHPMyAdmin因配置不當、版本過舊或環境安全隱患導致的安全風險,保障數據庫安全。
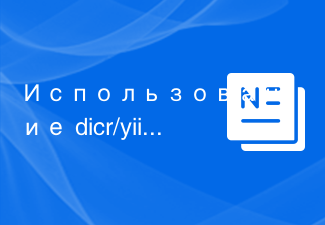
vProcesserazrabotkiveb被固定,мнелостольностьстьс粹餾標д都LeavallySumballanceFriablanceFaumDoptoMatification,Čtookazalovnetakprosto,kakaožidal.posenesko
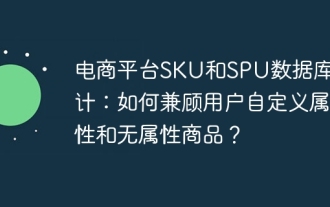
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
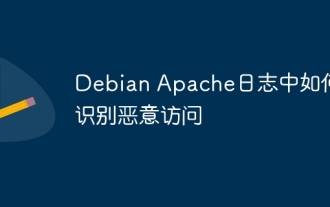
有效監控和防禦惡意網站訪問對於Debian系統的Apache服務器至關重要。 Apache訪問日誌是識別此類威脅的關鍵信息來源。本文將指導您如何分析日誌並採取防禦措施。識別惡意訪問行為Debian系統的Apache訪問日誌通常位於/var/log/apache2/access.log。您可以通過多種方法分析日誌:日誌文件位置確認:首先,請確認您的Apache訪問日誌的準確位置,它可能因係統配置而略有不同。命令行工具分析:使用grep命令搜索特定模式,例如grep"404"
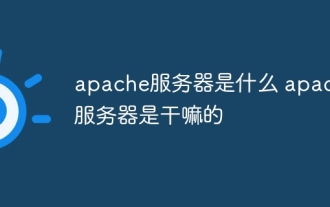
Apache服務器是強大的Web服務器軟件,充當瀏覽器與網站服務器間的橋樑。 1. 它處理HTTP請求,根據請求返回網頁內容;2. 模塊化設計允許擴展功能,例如支持SSL加密和動態網頁;3. 配置文件(如虛擬主機配置)需謹慎設置,避免安全漏洞,並需優化性能參數,例如線程數和超時時間,才能構建高性能、安全的Web應用。
