Libbson
Libbson is a new shared library written in C for developers wanting to work with the BSON serialization format. Its API will feel natural to C programmers but can also be used as the base of a C extension in higher-level MongoDB drivers. T
Libbson is a new shared library written in C for developers wanting to work with the BSON serialization format.
Its API will feel natural to C programmers but can also be used as the base of a C extension in higher-level MongoDB drivers.
The library contains everything you would expect from a BSON implementation. It has the ability to work with documents in their serialized form, iterating elements within a document, overwriting fields in place, Object Id generation, JSON conversion, data validation, and more. Some lessons were learned along the way that are beneficial for those choosing to implement BSON themselves.
Improving small document performance
A common use case of BSON is for relatively small documents. This has a profound impact on the memory allocator in userspace, causing what is commonly known as “memory fragmentation”. Memory fragmentation can make it more difficult for your allocator to locate a contiguous region of memory.
In addition to increasing allocation latency, it increases the memory requirements of your application to overcome that fragmentation.
To help with this issue, the bson_t structure contains 120 bytes of inline space that allows BSON documents to be built directly on the stack as opposed to the heap.
When the document size grows past 120 bytes it will automatically migrate to a heap allocation.
Additionally, bson_t will grow it’s buffers in powers of two. This is standard when working with buffers and arrays as it amortizes the overhead of growing the buffer versus calling realloc() every time data is appended. 120 bytes was chosen to align bson_t to the size of two sequential cachelines on x86_64 (each 64 bytes).
This may change based on future research, but not before a stable ABI has been reached.
Single allocation for nested documents
One strength of BSON is it’s ability to nest objects and arrays. Often times when serializing these nested documents, each sub-document is serialized independently and then appended to the parents buffer.
As you might imagine, this takes quite the toll on the allocator. It can generate many small allocations which were only created to have been immediately discarded after appending to the parents buffer. Libbson allows for building sub-documents directly into the parent documents buffer.
Doing so helps avoid this costly fragmentation. The topmost document will grow its underlying buffers in powers of two each time the allocation would overflow.
Parsing BSON documents from network buffers
Another common area for allocator fragmentation is during BSON document parsing. Libbson allows parsing and iteration of BSON documents directly from your incoming network buffer.
This means the only allocations created are those needed for your higher level language such as a PyDict if writing a Python extension.
Developers writing C extensions for their driver may choose to implement a “generator” style parsing of documents to help keep memory fragmentation low.
A technique we’re yet to explore is implementing a hashtable-esque structure backed by BSON, only deserializing the entire buffer after a threshold of keys have been accessed.
Generating BSON documents into network buffers
Much like parsing BSON documents, generating documents and placing them into your network buffers can be hard on your memory allocator. To help keep this fragmentation down, Libbson provides support for serializing your document to BSON directly within a buffer of your choosing.
This is ideal for situations such as writing a sequence of BSON documents into a MongoDB message.
Generating Object Ids without Synchronization
Applications are often doing ObjectId generation, especially in high insert environments. The uniqueness of generated ObjectIds is critical to avoiding duplicate key errors across multiple nodes.
Highly threaded environments create a local contention point slowing the rate of generation. This is because the threads must synchronize on the increment counter of each sequential ObjectId. Failure to do so could cause collisions that would not be detected until after a network round-trip. Most drivers implement the synchronization with an atomic increment or a mutex if atomics are not available.
Libbson will use atomic increments and in some cases avoid synchronization altogether if possible. One such case is a non-threaded environment.
Another is when running on Linux as both threads and processes are in the same namespace.
This allows the use of the thread identifier as the pid within the ObjectId.
You can find Libbson at https://github.com/mongodb/libbson and discuss design choices with its author, Christian Hergert, who can be found on twitter as @hergertme.
原文地址:Libbson, 感谢原作者分享。

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
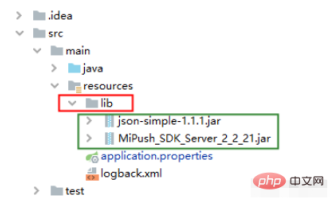
前言:工作中,碰到過springboot框架建構的javaweb項目,需要整合第三方推送功能,於是使用到了小米推送服務,下載了相關jar包。專案中引入本地jar,問題不大,寫完程式碼後,通過測試類別測試,也沒問題。然後就準備打包部署到開發服上。由於專案是透過tomcat部署的,所以打包方式是打成war包。打包後上傳到開發服,啟動成功後去測試編寫的推送接口,發現失敗了。透過分析發現,打包後的war中存放項目依賴jar的lib目錄中並沒有本地引入的推送相關的jar包。折騰了半小時,才解決了問題。解決
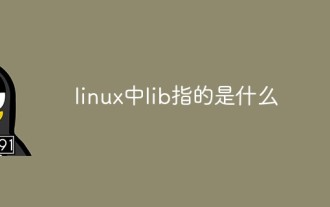
在linux中,lib是函式庫檔案目錄,包含了所有對系統有用的函式庫檔案;函式庫檔案是應用程式、指令或行程正確執行所需的檔案。 lib的作用類似Windows裡的DLL文件,幾乎所有的應用程式都需要用到lib目錄裡的共用函式庫檔案。 lib是Library(函式庫)的縮寫這個目錄裡存放著系統最基本的動態連線共享函式庫,其作用類似Windows裡的DLL檔。幾乎所有的應用程式都需要用到這些共享庫。 /lib資料夾是庫檔案目錄,包含了所有對系統有用的庫檔案。簡單來說,它是應用程式、命令或進程正確執行所需的檔案。在/bi
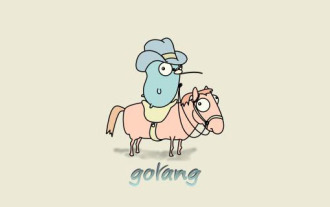
區別:1、make只能用來分配及初始化類型為slice、map、chan的資料;而new可以分配任意類型的資料。 2.new分配返回的是指針,即類型「*Type」;而make返回引用,即Type。 3.new分配的空間會被清除;make分配空間後,會初始化。
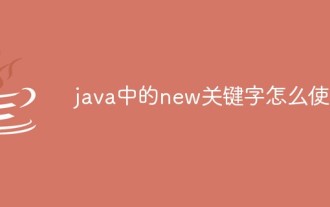
1.概念在Java語言裡,「new」表達式是負責建立實例的,其中會呼叫構造器去對實例做初始化;構造器本身的回傳值類型是void,並不是「建構器回傳了新建立的物件的參考”,而是new表達式的值是新建立的物件的參考。 2.用途新建類別的物件3.工作機制為物件成員分配記憶體空間,並指定預設值對成員變數進行明確初始化執行建構方法計算並傳回參考值4.實例new操作往往意味著記憶體中的開啟新的記憶體空間,這個記憶體空間分配在記憶體中的堆區,受到jvm控制,自動進行記憶體管理。這裡我們就是用String這個類別來舉例說明。 pu
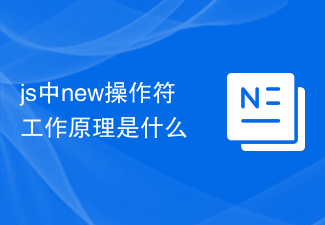
js中new運算元工作原理是什麼,需要具體程式碼範例js中的new操作符是用來建立物件的關鍵字。它的作用是根據指定的建構函數建立一個新的實例對象,並傳回該對象的參考。在使用new運算元時,實際上進行了以下幾個步驟:建立一個新的空物件;將該空物件的原型指向建構函式的原型物件;將建構函式的作用域賦給新物件(因此this指向了新物件);執行建構函式中的程式碼,並給新對
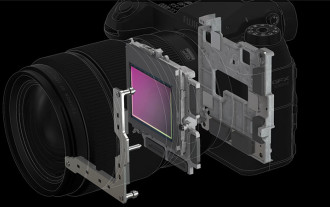
近年來,富士膠片取得了巨大的成功,這主要歸功於其膠片模擬技術以及其緊湊型測距相機在社交媒體上的流行。然而,據 Fujirumors 稱,它似乎並沒有滿足於現狀。你

linux找不到lib的解決方法:1、將程式中的lib庫複製到「/lib」或「/usr/local/lib」目錄下,然後執行「ldconfig」;2、在「ld.so.conf 」中加入庫檔案所在目錄,然後更新「ld.so.cache」檔案即可。
