CI框架中redis缓存相关操作文件示例代码,ciredis
CI框架中redis缓存相关操作文件示例代码,ciredis
本文实例讲述了CI框架中redis缓存相关操作文件。分享给大家供大家参考,具体如下:
redis缓存类文件位置:
'ci\system\libraries\Cache\drivers\Cache_redis.php'
<?php /** * CodeIgniter * * An open source application development framework for PHP 5.2.4 or newer * * NOTICE OF LICENSE * * Licensed under the Open Software License version 3.0 * * This source file is subject to the Open Software License (OSL 3.0) that is * bundled with this package in the files license.txt / license.rst. It is * also available through the world wide web at this URL: * http://opensource.org/licenses/OSL-3.0 * If you did not receive a copy of the license and are unable to obtain it * through the world wide web, please send an email to * licensing@ellislab.com so we can send you a copy immediately. * * @package CodeIgniter * @author EllisLab Dev Team * @copyright Copyright (c) 2008 - 2014, EllisLab, Inc. (http://ellislab.com/) * @license http://opensource.org/licenses/OSL-3.0 Open Software License (OSL 3.0) * @link http://codeigniter.com * @since Version 3.0 * @filesource */ defined('BASEPATH') OR exit('No direct script access allowed'); /** * CodeIgniter Redis Caching Class * * @package CodeIgniter * @subpackage Libraries * @category Core * @author Anton Lindqvist <anton@qvister.se> * @link */ class CI_Cache_redis extends CI_Driver { /** * Default config * * @static * @var array */ protected static $_default_config = array( /* 'socket_type' => 'tcp', 'host' => '127.0.0.1', 'password' => NULL, 'port' => 6379, 'timeout' => 0 */ ); /** * Redis connection * * @var Redis */ protected $_redis; /** * Get cache * * @param string like *$key* * @return array(hash) */ public function keys($key) { return $this->_redis->keys($key); } /** * Get cache * * @param string Cache ID * @return mixed */ public function get($key) { return $this->_redis->get($key); } /** * mGet cache * * @param array Cache ID Array * @return mixed */ public function mget($keys) { return $this->_redis->mget($keys); } /** * Save cache * * @param string $id Cache ID * @param mixed $data Data to save * @param int $ttl Time to live in seconds * @param bool $raw Whether to store the raw value (unused) * @return bool TRUE on success, FALSE on failure */ public function save($id, $data, $ttl = 60, $raw = FALSE) { return ($ttl) ? $this->_redis->setex($id, $ttl, $data) : $this->_redis->set($id, $data); } /** * Delete from cache * * @param string Cache key * @return bool */ public function delete($key) { return ($this->_redis->delete($key) === 1); } /** * hIncrBy a raw value * * @param string $id Cache ID * @param string $field Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hincrby($key, $field, $value = 1) { return $this->_redis->hIncrBy($key, $field, $value); } /** * hIncrByFloat a raw value * * @param string $id Cache ID * @param string $field Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hincrbyfloat($key, $field, $value = 1) { return $this->_redis->hIncrByFloat($key, $field, $value); } /** * lpush a raw value * * @param string $key Cache ID * @param string $value value * @return mixed New value on success or FALSE on failure */ public function lpush($key, $value) { return $this->_redis->lPush($key, $value); } /** * rpush a raw value * * @param string $key Cache ID * @param string $value value * @return mixed New value on success or FALSE on failure */ public function rpush($key, $value) { return $this->_redis->rPush($key, $value); } /** * rpop a raw value * * @param string $key Cache ID * @param string $value value * @return mixed New value on success or FALSE on failure */ public function rpop($key) { return $this->_redis->rPop($key); } /** * brpop a raw value * * @param string $key Cache ID * @param string $ontime 阻塞等待时间 * @return mixed New value on success or FALSE on failure */ public function brpop($key,$ontime=0) { return $this->_redis->brPop($key,$ontime); } /** * lLen a raw value * * @param string $key Cache ID * @return mixed Value on success or FALSE on failure */ public function llen($key) { return $this->_redis->lLen($key); } /** * Increment a raw value * * @param string $id Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function increment($id, $offset = 1) { return $this->_redis->exists($id) ? $this->_redis->incr($id, $offset) : FALSE; } /** * incrby a raw value * * @param string $key Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function incrby($key, $value = 1) { return $this->_redis->incrby($key, $value); } /** * set a value expire time * * @param string $key Cache ID * @param int $seconds expire seconds * @return mixed New value on success or FALSE on failure */ public function expire($key, $seconds) { return $this->_redis->expire($key, $seconds); } /** * Increment a raw value * * @param string $id Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hset($alias,$key, $value) { return $this->_redis->hset($alias,$key, $value); } /** * Increment a raw value * * @param string $id Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hget($alias,$key) { return $this->_redis->hget($alias,$key); } /** * Increment a raw value * * @param string $id Cache ID * @return mixed New value on success or FALSE on failure */ public function hkeys($alias) { return $this->_redis->hkeys($alias); } /** * Increment a raw value * * @param string $id Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hgetall($alias) { return $this->_redis->hgetall($alias); } /** * Increment a raw value * * @param string $id Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hmget($alias,$key) { return $this->_redis->hmget($alias,$key); } /** * del a key value * * @param string $id Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hdel($alias,$key) { return $this->_redis->hdel($alias,$key); } /** * del a key value * * @param string $id Cache ID * @return mixed New value on success or FALSE on failure */ public function hvals($alias) { return $this->_redis->hvals($alias); } /** * Increment a raw value * * @param string $id Cache ID * @param int $offset Step/value to add * @return mixed New value on success or FALSE on failure */ public function hmset($alias,$array) { return $this->_redis->hmset($alias,$array); } /** * Decrement a raw value * * @param string $id Cache ID * @param int $offset Step/value to reduce by * @return mixed New value on success or FALSE on failure */ public function decrement($id, $offset = 1) { return $this->_redis->exists($id) ? $this->_redis->decr($id, $offset) : FALSE; } /** * Clean cache * * @return bool * @see Redis::flushDB() */ public function clean() { return $this->_redis->flushDB(); } /** * Get cache driver info * * @param string Not supported in Redis. * Only included in order to offer a * consistent cache API. * @return array * @see Redis::info() */ public function cache_info($type = NULL) { return $this->_redis->info(); } /** * Get cache metadata * * @param string Cache key * @return array */ public function get_metadata($key) { $value = $this->get($key); if ($value) { return array( 'expire' => time() + $this->_redis->ttl($key), 'data' => $value ); } return FALSE; } /** * Check if Redis driver is supported * * @return bool */ public function is_supported() { if (extension_loaded('redis')) { return $this->_setup_redis(); } else { log_message('debug', 'The Redis extension must be loaded to use Redis cache.'); return FALSE; } } /** * Setup Redis config and connection * * Loads Redis config file if present. Will halt execution * if a Redis connection can't be established. * * @return bool * @see Redis::connect() */ protected function _setup_redis() { $config = array(); $CI =& get_instance(); if ($CI->config->load('redis', TRUE, TRUE)) { $config += $CI->config->item('redis'); } $config = array_merge(self::$_default_config, $config); $config = !empty($config['redis'])?$config['redis']:$config; $this->_redis = new Redis(); try { if ($config['socket_type'] === 'unix') { $success = $this->_redis->connect($config['socket']); } else // tcp socket { $success = $this->_redis->connect($config['host'], $config['port'], $config['timeout']); } if ( ! $success) { log_message('debug', 'Cache: Redis connection refused. Check the config.'); return FALSE; } } catch (RedisException $e) { log_message('debug', 'Cache: Redis connection refused ('.$e->getMessage().')'); return FALSE; } if (isset($config['password'])) { $this->_redis->auth($config['password']); } return TRUE; } /** * Class destructor * * Closes the connection to Redis if present. * * @return void */ public function __destruct() { if ($this->_redis) { $this->_redis->close(); } } } /* End of file Cache_redis.php */ /* Location: ./system/libraries/Cache/drivers/Cache_redis.php */
更多关于CodeIgniter相关内容感兴趣的读者可查看本站专题:《codeigniter入门教程》、《CI(CodeIgniter)框架进阶教程》、《php优秀开发框架总结》、《Yii框架入门及常用技巧总结》、《php缓存技术总结》、《php面向对象程序设计入门教程》、《php+mysql数据库操作入门教程》及《php常见数据库操作技巧汇总》
希望本文所述对大家基于CodeIgniter框架的PHP程序设计有所帮助。

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
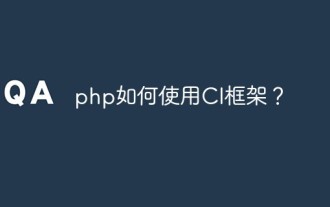
隨著網路技術的發展,PHP成為了Web開發的重要工具之一。而其中一款流行的PHP框架——CodeIgniter(以下簡稱CI)也得到了越來越多的關注與使用。今天,我們就來看看如何使用CI框架。一、安裝CI框架首先,我們需要下載CI框架並安裝。在CI的官網(https://codeigniter.com/)上下載最新版本的CI框架壓縮包。下載完成後,解壓縮
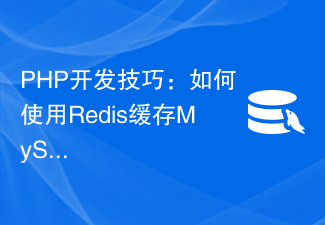
PHP開發技巧:如何使用Redis快取MySQL查詢結果引言:在Web開發過程中,資料庫查詢是常見操作之一。然而,頻繁的資料庫查詢會導致效能問題,影響網頁的載入速度。為了提高查詢效率,我們可以使用Redis作為緩存,將經常被查詢的資料放入Redis中,從而減少對MySQL的查詢次數,提高網頁的回應速度。本文將介紹如何使用Redis快取MySQL查詢結果的開發
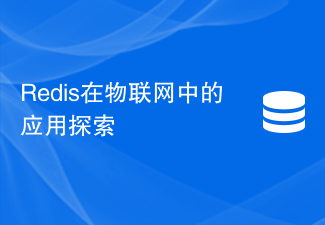
Redis在物聯網的應用探索在現今物聯網(InternetofThings,IoT)快速發展的時代,海量的設備連結在一起,為我們提供了豐富的資料資源。而隨著物聯網應用越來越廣泛,大規模資料的處理和儲存成為了亟需解決的問題。 Redis作為一種高效能的記憶體資料儲存系統,具有出色的資料處理能力和低延遲的特點,為物聯網應用帶來了許多的優勢。 Redis是一個開
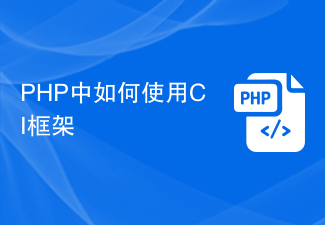
PHP是一種流行的程式語言,廣泛應用於Web開發。 CI(CodeIgniter)框架是PHP中最受歡迎的框架之一,它提供了一整套現成的工具和函數庫,以及一些流行的設計模式,讓開發人員能夠更有效率地開發Web應用程式。本文將介紹使用CI框架開發PHP應用程式的基本步驟和方法。了解CI架構的基本概念和結構在使用CI架構之前,我們需要先了解一些基本的概念和結構。下
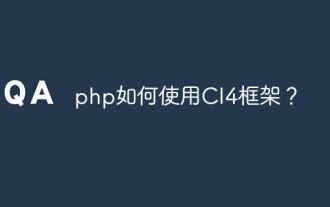
PHP是一種廣泛使用的伺服器端腳本語言,而CodeIgniter4(CI4)是一個流行的PHP框架,它提供了一種快速而優秀的方法來建立Web應用程式。在這篇文章中,我們將透過引導您了解如何使用CI4框架,讓您開始使用此框架來開發出眾的網路應用程式。 1.下載並安裝CI4首先,您需要從官方網站(https://codeigniter.com/downloa
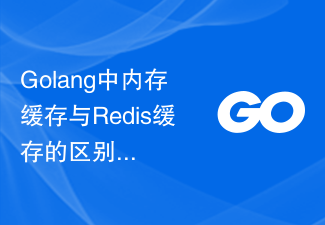
隨著應用程式規模的不斷擴大,對數據的需求也越來越大。快取作為資料讀寫的最佳化方式,已經成為現代應用程式中不可或缺的組成部分。在快取的選擇方面,Golang中自帶的記憶體快取與Redis快取都是比較常見的選擇。本文將對兩者進行比較與分析,幫助讀者做出更適合的選擇。一、記憶體快取與Redis快取的區別資料持久性記憶體快取與Redis快取最大的差別在於資料的持久性。
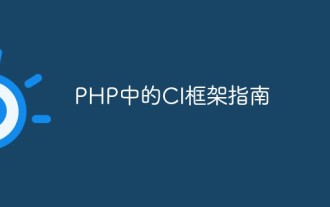
隨著網路的發展和不斷地融入人們的生活,網路應用的開發變得越來越重要。 PHP作為一種眾所周知的程式語言,已經成為了開發網路應用程式的首選語言之一。而開發人員可以使用眾多的PHP框架來簡化開發過程,其中最受歡迎的之一是CodeIgniter(CI)框架。 CI是一個強大的PHPweb應用框架,它擁有輕量級、簡單易用、優化性能等特點,可以讓開發人員快速構建

CI框架中引入CSS樣式的步驟如下:1、準備CSS檔案;2、將CSS檔案儲存在CI框架專案的適當位置;3、在需要使用CSS樣式的頁面中,透過HTML的<link>標籤引入CSS文件;4、在HTML元素中使用CSS類別或ID名稱來套用對應的樣式即可。
