ajax php多文件上传代码
ajax php教程多文件上传代码
<script><br /> (function(){<br /> <br /> var d = document, w = window; <p>/**<br /> * get element by id<br /> */ <br /> function get(element){<br /> if (typeof element == "string")<br /> element = d.getelementbyid(element);<br /> return element;<br /> } <p>/**<br /> * attaches event to a dom element<br /> */<br /> function addevent(el, type, fn){<br /> if (w.addeventlistener){<br /> el.addeventlistener(type, fn, false);<br /> } else if (w.attachevent){<br /> var f = function(){<br /> fn.call(el, w.event);<br /> }; <br /> el.attachevent('on' + type, f)<br /> }<br /> } <p><br /> /**<br /> * creates and returns element from html chunk<br /> */<br /> var toelement = function(){<br /> var div = d.createelement('div');<br /> return function(html){<br /> div.innerhtml = html;<br /> var el = div.childnodes[0];<br /> div.removechild(el);<br /> return el;<br /> }<br /> }(); <p>function hasclass(ele,cls){<br /> return ele.classname.match(new regexp('(s|^)'+cls+'(s|$)'));<br /> }<br /> function addclass(ele,cls) {<br /> if (!hasclass(ele,cls)) ele.classname += " "+cls;<br /> }<br /> function removeclass(ele,cls) {<br /> var reg = new regexp('(s|^)'+cls+'(s|$)');<br /> ele.classname=ele.classname.replace(reg,' ');<br /> } <p>// getoffset function copied from jquery lib (http://jquery.com/)<br /> if (document.documentelement["getboundingclientrect"]){<br /> // get offset using getboundingclientrect<br /> // http://ejohn.org/blog/getboundingclientrect-is-awesome/<br /> var getoffset = function(el){<br /> var box = el.getboundingclientrect(),<br /> doc = el.ownerdocument,<br /> body = doc.body,<br /> docelem = doc.documentelement,<br /> <br /> // for ie <br /> clienttop = docelem.clienttop || body.clienttop || 0,<br /> clientleft = docelem.clientleft || body.clientleft || 0,<br /> <br /> // in internet explorer 7 getboundingclientrect property is treated as physical,<br /> // while others are logical. make all logical, like in ie8. <br /> <br /> zoom = 1;<br /> <br /> if (body.getboundingclientrect) {<br /> var bound = body.getboundingclientrect();<br /> zoom = (bound.right - bound.left)/body.clientwidth;<br /> }<br /> <br /> if (zoom > 1){<br /> clienttop = 0;<br /> clientleft = 0;<br /> }<br /> <br /> var top = box.top/zoom + (window.pageyoffset || docelem && docelem.scrolltop/zoom || body.scrolltop/zoom) - clienttop,<br /> left = box.left/zoom + (window.pagexoffset|| docelem && docelem.scrollleft/zoom || body.scrollleft/zoom) - clientleft;<br /> <br /> return {<br /> top: top,<br /> left: left<br /> };<br /> }<br /> <br /> } else {<br /> // get offset adding all offsets <br /> var getoffset = function(el){<br /> if (w.jquery){<br /> return jquery(el).offset();<br /> } <br /> <br /> var top = 0, left = 0;<br /> do {<br /> top += el.offsettop || 0;<br /> left += el.offsetleft || 0;<br /> }<br /> while (el = el.offsetparent);<br /> <br /> return {<br /> left: left,<br /> top: top<br /> };<br /> }<br /> } <p>function getbox(el){<br /> var left, right, top, bottom; <br /> var offset = getoffset(el);<br /> left = offset.left;<br /> top = offset.top;<br /> <br /> right = left + el.offsetwidth;<br /> bottom = top + el.offsetheight; <br /> <br /> return {<br /> left: left,<br /> right: right,<br /> top: top,<br /> bottom: bottom<br /> };<br /> } <p>/**<br /> * crossbrowser mouse coordinates<br /> */<br /> function getmousecoords(e){ <br /> // pagex/y is not supported in ie<br /> // http://www.quirksmode.org/dom/w3c_css教程om.html <br /> if (!e.pagex && e.clientx){<br /> // in internet explorer 7 some properties (mouse coordinates) are treated as physical,<br /> // while others are logical (offset).<br /> var zoom = 1; <br /> var body = document.body;<br /> <br /> if (body.getboundingclientrect) {<br /> var bound = body.getboundingclientrect();<br /> zoom = (bound.right - bound.left)/body.clientwidth;<br /> } <p> return {<br /> x: e.clientx / zoom + d.body.scrollleft + d.documentelement.scrollleft,<br /> y: e.clienty / zoom + d.body.scrolltop + d.documentelement.scrolltop<br /> };<br /> }<br /> <br /> return {<br /> x: e.pagex,<br /> y: e.pagey<br /> }; <p>}<br /> /**<br /> * function generates unique id<br /> */ <br /> var getuid = function(){<br /> var id = 0;<br /> return function(){<br /> return 'valumsajaxupload' + id++;<br /> }<br /> }(); <p>function filefrompath(file){<br /> return file.replace(/.*(/|)/, ""); <br /> } <p>function getext(file){<br /> return (/[.]/.exec(file)) ? /[^.]+$/.exec(file.tolowercase()) : '';<br /> } <p>/**<br /> * cross-browser way to get xhr object <br /> */<br /> var getxhr = function(){<br /> var xhr;<br /> <br /> return function(){<br /> if (xhr) return xhr;<br /> <br /> if (typeof xmlhttprequest !== 'undefined') {<br /> xhr = new xmlhttprequest();<br /> } else {<br /> var v = [<br /> "microsoft.xmlhttp",<br /> "msxml2.xmlhttp.5.0",<br /> "msxml2.xmlhttp.4.0",<br /> "msxml2.xmlhttp.3.0",<br /> "msxml2.xmlhttp.2.0" <br /> ];<br /> <br /> for (var i=0; i < v.length; i++){<br /> try {<br /> xhr = new activexobject(v[i]);<br /> break;<br /> } catch (e){}<br /> }<br /> } <p> return xhr;<br /> }<br /> }(); <p>// please use ajaxupload , ajax_upload will be removed in the next version<br /> ajax_upload = ajaxupload = function(button, options){<br /> if (button.jquery){<br /> // jquery object was passed<br /> button = button[0];<br /> } else if (typeof button == "string" && /^#.*/.test(button)){ <br /> button = button.slice(1); <br /> }<br /> button = get(button); <br /> <br /> this._input = null;<br /> this._button = button;<br /> this._disabled = false;<br /> this._submitting = false;<br /> // variable changes to true if the button was clicked<br /> // 3 seconds ago (requred to fix safari on mac error)<br /> this._justclicked = false;<br /> this._parentdialog = d.body;<br /> <br /> if (window.jquery && jquery.ui && jquery.ui.dialog){<br /> var parentdialog = jquery(this._button).parents('.ui-dialog');<br /> if (parentdialog.length){<br /> this._parentdialog = parentdialog[0];<br /> }<br /> } <br /> <br /> this._settings = {<br /> // location of the server-side upload script<br /> action: 'upload.php', <br /> // file upload name<br /> name: 'userfile',<br /> // additional data to send<br /> data: {},<br /> // submit file as soon as it's selected<br /> autosubmit: true,<br /> // the type of data that you're expecting back from the server.<br /> // html and xml are detected automatically.<br /> // only useful when you are using json data as a response.<br /> // set to "json" in that case. <br /> responsetype: false,<br /> // location of the server-side script that fixes safari <br /> // hanging problem returning "connection: close" header<br /> closeconnection: '',<br /> // class applied to button when mouse is hovered<br /> hoverclass: 'hover', <br /> // when user selects a file, useful with autosubmit disabled <br /> onchange: function(file, extension){}, <br /> // callback to fire before file is uploaded<br /> // you can return false to cancel upload<br /> onsubmit: function(file, extension){},<br /> // fired when file upload is completed<br /> // warning! do not use "false" string as a response!<br /> oncomplete: function(file, response) {}<br /> }; <p> // merge the users options with our defaults<br /> for (var i in options) {<br /> this._settings[i] = options[i];<br /> }<br /> <br /> this._createinput();<br /> this._rerouteclicks();<br /> }<br /> <br /> // assigning methods to our class<br /> ajaxupload.prototype = {<br /> setdata : function(data){<br /> this._settings.data = data;<br /> },<br /> disable : function(){<br /> this._disabled = true;<br /> },<br /> enable : function(){<br /> this._disabled = false;<br /> },<br /> // removes instance<br /> destroy : function(){<br /> if(this._input){<br /> if(this._input.parentnode){<br /> this._input.parentnode.removechild(this._input);<br /> }<br /> this._input = null;<br /> }<br /> }, <br /> /**<br /> * creates invisible file input above the button <br /> */<br /> _createinput : function(){<br /> var self = this;<br /> var input = d.createelement("input");<br /> input.setattribute('type', 'file');<br /> input.setattribute('name', this._settings.name);<br /> var styles = {<br /> 'position' : 'absolute'<br /> ,'margin': '-5px 0 0 -175px'<br /> ,'padding': 0<br /> ,'width': '220px'<br /> ,'height': '30px'<br /> ,'fontsize': '14px' <br /> ,'opacity': 0<br /> ,'cursor': 'pointer'<br /> ,'display' : 'none'<br /> ,'zindex' : 2147483583 //max zindex supported by opera 9.0-9.2x <br /> // strange, i expected 2147483647<br /> // doesn't work in ie :(<br /> //,'direction' : 'ltr' <br /> };<br /> for (var i in styles){<br /> input.style[i] = styles[i];<br /> }<br /> <br /> // make sure that element opacity exists<br /> // (ie uses filter instead)<br /> if ( ! (input.style.opacity === "0")){<br /> input.style.filter = "alpha(opacity=0)";<br /> }<br /> <br /> this._parentdialog.appendchild(input); <p> addevent(input, 'change', function(){<br /> // get filename from input<br /> var file = filefrompath(this.value); <br /> if(self._settings.onchange.call(self, file, getext(file)) == false ){<br /> return; <br /> } <br /> // submit form when value is changed<br /> if (self._settings.autosubmit){<br /> self.submit(); <br /> } <br /> });<br /> <br /> // fixing problem with safari<br /> // the problem is that if you leave input before the file select dialog opens<br /> // it does not upload the file.<br /> // as dialog opens slowly (it is a sheet dialog which takes some time to open)<br /> // there is some time while you can leave the button.<br /> // so we should not change display to none immediately<br /> addevent(input, 'click', function(){<br /> self.justclicked = true;<br /> settimeout(function(){<br /> // we will wait 3 seconds for dialog to open<br /> self.justclicked = false;<br /> }, 2500); <br /> }); <br /> <br /> this._input = input;<br /> },<br /> _rerouteclicks : function (){<br /> var self = this;<br /> <br /> // ie displays 'access denied' error when using this method<br /> // other browsers just ignore click()<br /> // addevent(this._button, 'click', function(e){<br /> // self._input.click();<br /> // });<br /> <br /> var box, dialogoffset = {top:0, left:0}, over = false;<br /> <br /> addevent(self._button, 'mouseo教程ver', function(e){<br /> if (!self._input || over) return;<br /> <br /> over = true;<br /> box = getbox(self._button);<br /> <br /> if (self._parentdialog != d.body){<br /> dialogoffset = getoffset(self._parentdialog);<br /> } <br /> });<br /> <br /> <br /> // we can't use mouseout on the button,<br /> // because invisible input is over it<br /> addevent(document, 'mousemove', function(e){<br /> var input = self._input; <br /> if (!input || !over) return;<br /> <br /> if (self._disabled){<br /> removeclass(self._button, self._settings.hoverclass);<br /> input.style.display = 'none';<br /> return;<br /> } <br /> <br /> var c = getmousecoords(e); <p> if ((c.x >= box.left) && (c.x <= box.right) && <br /> (c.y >= box.top) && (c.y <= box.bottom)){<br /> <br /> input.style.top = c.y - dialogoffset.top + 'px';<br /> input.style.left = c.x - dialogoffset.left + 'px';<br /> input.style.display = 'block';<br /> addclass(self._button, self._settings.hoverclass);<br /> <br /> } else { <br /> // mouse left the button<br /> over = false;<br /> <br /> var check = setinterval(function(){<br /> // if input was just clicked do not hide it<br /> // to prevent safari bug<br /> <br /> if (self.justclicked){<br /> return;<br /> }<br /> <br /> if ( !over ){<br /> input.style.display = 'none'; <br /> } <br /> <br /> clearinterval(check);<br /> <br /> }, 25);<br /> <p> removeclass(self._button, self._settings.hoverclass);<br /> } <br /> }); <br /> <br /> },<br /> /**<br /> * creates iframe with unique name<br /> */<br /> _createiframe : function(){<br /> // unique name<br /> // we cannot use gettime, because it sometimes return<br /> // same value in safari :(<br /> var id = getuid();<br /> <br /> // remove ie6 "this page contains both secure and nonsecure items" prompt <br /> // http://tinyurl.com/77w9wh<br /> var iframe = toelement('<iframe src="网页特效:false;" name="' + id + '" />');<br /> iframe.id = id;<br /> iframe.style.display = 'none';<br /> d.body.appendchild(iframe); <br /> return iframe; <br /> },<br /> /**<br /> * upload file without refreshing the page<br /> */<br /> submit : function(){<br /> var self = this, settings = this._settings; <br /> <br /> if (this._input.value === ''){<br /> // there is no file<br /> return;<br /> }<br /> <br /> // get filename from input<br /> var file = filefrompath(this._input.value); <p> // execute user event<br /> if (! (settings.onsubmit.call(this, file, getext(file)) == false)) {<br /> // create new iframe for this submission<br /> var iframe = this._createiframe();<br /> <br /> // do not submit if user function returns false <br /> var form = this._createform(iframe);<br /> form.appendchild(this._input); <p> // a pretty little hack to make uploads not hang in safari. just call this<br /> // immediately before the upload is submitted. this does an ajax call to<br /> // the server, which returns an empty document with the "connection: close"<br /> // header, telling safari to close the active connection.<br /> // http://blog.airbladesoftware.com/2007/8/17/note-to-self-prevent-uploads-hanging-in-safari<br /> if (settings.closeconnection && /applewebkit|msie/.test(navigator.useragent)){<br /> var xhr = getxhr();<br /> // open synhronous connection<br /> xhr.open('get', settings.closeconnection, false);<br /> xhr.send('');<br /> }<br /> <br /> form.submit();<br /> <br /> d.body.removechild(form); <br /> form = null;<br /> this._input = null;<br /> <br /> // create new input<br /> this._createinput();<br /> <br /> var todeleteflag = false;<br /> <br /> addevent(iframe, 'load', function(e){<br /> <br /> if (// for safari<br /> iframe.src == "javascript:'%3chtml%3e%3c/html%3e';" ||<br /> // for ff, ie<br /> iframe.src == "javascript:'<html>';"){ <br /> <br /> // first time around, do not delete.<br /> if( todeleteflag ){<br /> // fix busy state in ff3<br /> settimeout( function() {<br /> d.body.removechild(iframe);<br /> }, 0);<br /> }<br /> return;<br /> } <br /> <br /> var doc = iframe.contentdocument ? iframe.contentdocument : frames[iframe.id].document; <p> // fixing opera 9.26<br /> if (doc.readystate && doc.readystate != 'complete'){<br /> // opera fires load event multiple times<br /> // even when the dom is not ready yet<br /> // this fix should not affect other browsers<br /> return;<br /> }<br /> <br /> // fixing opera 9.64<br /> if (doc.body && doc.body.innerhtml == "false"){<br /> // in opera 9.64 event was fired second time<br /> // when body.innerhtml changed from false <br /> // to server response approx. after 1 sec<br /> return; <br /> }<br /> <br /> var response;<br /> <br /> if (doc.xmldocument){<br /> // response is a xml document ie property<br /> response = doc.xmldocument;<br /> } else if (doc.body){<br /> // response is html document or plain text<br /> response = doc.body.innerhtml;<br /> if (settings.responsetype && settings.responsetype.tolowercase() == 'json'){<br /> // if the document was sent as 'application/javascript' or<br /> // 'text/javascript', then the browser wraps教程 the text in a <pre class="brush:php;toolbar:false"><br /> // tag and performs html encoding on the contents. in this case,<br /> // we need to pull the original text content from the text node's<br /> // nodevalue property to retrieve the unmangled content.<br /> // note that ie6 only understands text/html<br /> if (doc.body.firstchild && doc.body.firstchild.nodename.touppercase() == 'pre'){<br /> response = doc.body.firstchild.firstchild.nodevalue;<br /> }<br /> if (response) {<br /> response = window["eval"]("(" + response + ")");<br /> } else {<br /> response = {};<br /> }<br /> }<br /> } else {<br /> // response is a xml document<br /> var response = doc;<br /> }<br /> <br /> settings.oncomplete.call(self, file, response);<br /> <br /> // reload blank page, so that reloading main page<br /> // does not re-submit the post. also, remember to<br /> // delete the frame<br /> todeleteflag = true;<br /> <br /> // fix ie mixed content issue<br /> iframe.src = "javascript:'<html>';"; <br /> });<br /> <br /> } else {<br /> // clear input to allow user to select same file<br /> // doesn't work in ie6<br /> // this._input.value = '';<br /> d.body.removechild(this._input); <br /> this._input = null;<br /> <br /> // create new input<br /> this._createinput(); <br /> }<br /> }, <br /> /**<br /> * creates form, that will be submitted to iframe<br /> */<br /> _createform : function(iframe){<br /> var settings = this._settings;<br /> <br /> // method, enctype must be specified here<br /> // because changing this attr on the fly is not allowed in ie 6/7 <br /> var form = toelement('<form method="post" enctype="multipart/form-data">');<br /> form.style.display = 'none';<br /> form.action = settings.action;<br /> form.target = iframe.name;<br /> d.body.appendchild(form);<br /> <br /> // create hidden input element for each data key<br /> for (var prop in settings.data){<br /> var el = d.createelement("input");<br /> el.type = 'hidden';<br /> el.name = prop;<br /> el.value = settings.data[prop];<br /> form.appendchild(el);<br /> } <br /> return form;<br /> } <br /> };<br /> })();<br /> </script>

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
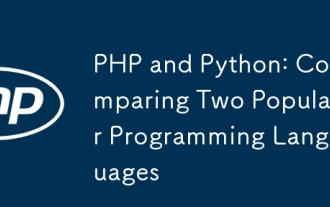
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。

WebDevelovermentReliesonHtml,CSS和JavaScript:1)HTMLStructuresContent,2)CSSStyleSIT和3)JavaScriptAddSstractivity,形成thebasisofmodernWebemodernWebExexperiences。
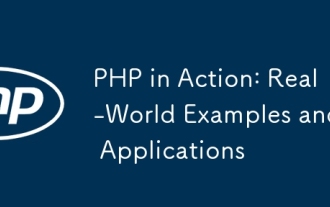
PHP在電子商務、內容管理系統和API開發中廣泛應用。 1)電子商務:用於購物車功能和支付處理。 2)內容管理系統:用於動態內容生成和用戶管理。 3)API開發:用於RESTfulAPI開發和API安全性。通過性能優化和最佳實踐,PHP應用的效率和可維護性得以提升。
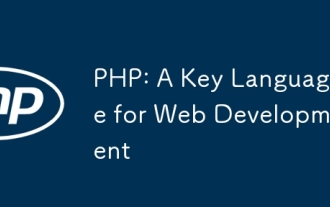
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
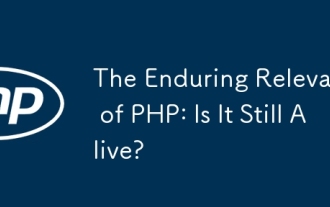
PHP仍然具有活力,其在現代編程領域中依然佔據重要地位。 1)PHP的簡單易學和強大社區支持使其在Web開發中廣泛應用;2)其靈活性和穩定性使其在處理Web表單、數據庫操作和文件處理等方面表現出色;3)PHP不斷進化和優化,適用於初學者和經驗豐富的開發者。
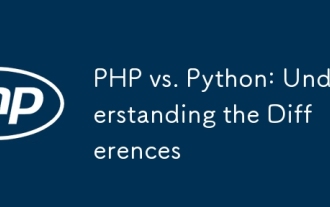
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
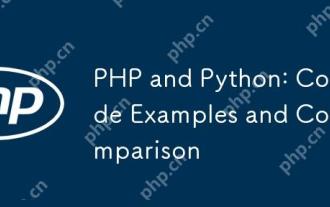
PHP和Python各有優劣,選擇取決於項目需求和個人偏好。 1.PHP適合快速開發和維護大型Web應用。 2.Python在數據科學和機器學習領域佔據主導地位。
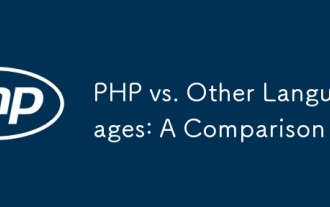
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
