如何在 Golang 中進行非同步資料庫操作?
在 Golang 中非同步資料庫操作有兩種主要方式:使用協程:在後台執行緒中非同步執行查詢。若查詢取消,程式會列印 "查詢已取消" 資訊。使用 goroutine pool:對於處理大量並行查詢的應用程序,goroutine pool 可以提高效能,因為它可以重用協程來執行任務。
在 Golang 中進行非同步資料庫操作
非同步資料庫操作允許應用程式在等待查詢結果時繼續執行其他任務。這可以大大提高應用程式的效能,特別是對於涉及大量資料庫 I/O 的應用程式。
使用協程
在 Golang 中進行非同步資料庫操作的常用方法是使用協程(goroutine
)。協程是並發執行的輕量級線程,可以與 main 線程同時運行。
以下程式碼範例示範如何使用協程非同步執行資料庫查詢:
package main import ( "context" "database/sql" "fmt" "time" ) func main() { // 打开数据库连接 db, err := sql.Open("postgres", "user=postgres password=my-password database=database host=localhost port=5432") if err != nil { panic(err) } defer db.Close() // 创建用于取消查询的上下文 ctx := context.Background() // 创建协程 go func() { // 使用 ctx.Done() 检查是否已取消查询 for { select { case <-ctx.Done(): fmt.Println("查询已取消") return default: // 执行查询 rows, err := db.QueryContext(ctx, "SELECT name FROM people") if err != nil { fmt.Println(err) continue } // 迭代查询结果 for rows.Next() { var name string if err := rows.Scan(&name); err != nil { fmt.Println(err) continue } fmt.Println(name) } rows.Close() } } }() // 等待一段时间,然后取消查询 time.Sleep(time.Second * 2) ctx.Cancel() }
這段程式碼會在後台協程中非同步執行一個資料庫查詢。如果在查詢完成之前取消該查詢,則程式碼會列印 "查詢已取消" 資訊。
使用 goroutine pool
對於需要處理大量並行資料庫查詢的應用程序,使用 goroutine pool 可以提高效能。 goroutine pool 是一組管理的協程,可以重複使用以執行任務。
以下程式碼範例示範如何使用 goroutine pool 進行非同步資料庫操作:
package main import ( "context" "database/sql" "fmt" "sync" "time" ) func main() { // 创建 goroutine pool pool := sync.Pool{ New: func() interface{} { return &sql.DB{} }, } // 打开数据库连接 db := pool.Get().(*sql.DB) defer pool.Put(db) // 创建用于取消查询的上下文 ctx := context.Background() // 创建 goroutine go func() { // 使用 ctx.Done() 检查是否已取消查询 for { select { case <-ctx.Done(): fmt.Println("查询已取消") return default: // 执行查询 rows, err := db.QueryContext(ctx, "SELECT name FROM people") if err != nil { fmt.Println(err) continue } // 迭代查询结果 for rows.Next() { var name string if err := rows.Scan(&name); err != nil { fmt.Println(err) continue } fmt.Println(name) } rows.Close() } } }() // 等待一段时间,然后取消查询 time.Sleep(time.Second * 2) ctx.Cancel() }
這段程式碼與上一個範例類似,但它使用 goroutine pool 來管理協程。這可以減少創建新協程的開銷,從而提高效能。
以上是如何在 Golang 中進行非同步資料庫操作?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
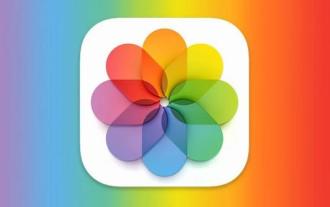
蘋果公司最新發布的iOS18、iPadOS18以及macOSSequoia系統為Photos應用程式增添了一項重要功能,旨在幫助用戶輕鬆恢復因各種原因遺失或損壞的照片和影片。這項新功能在Photos應用的"工具"部分引入了一個名為"已恢復"的相冊,當用戶設備中存在未納入其照片庫的圖片或影片時,該相冊將自動顯示。 "已恢復"相簿的出現為因資料庫損壞、相機應用未正確保存至照片庫或第三方應用管理照片庫時照片和視頻丟失提供了解決方案。使用者只需簡單幾步
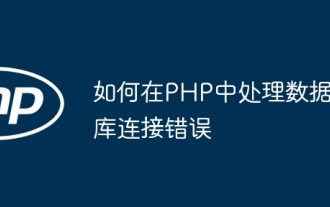
PHP處理資料庫連線報錯,可以使用下列步驟:使用mysqli_connect_errno()取得錯誤代碼。使用mysqli_connect_error()取得錯誤訊息。透過擷取並記錄這些錯誤訊息,可以輕鬆識別並解決資料庫連接問題,確保應用程式的順暢運作。
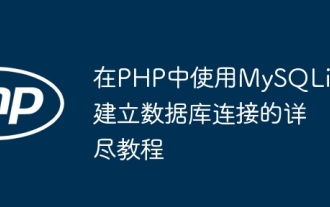
如何在PHP中使用MySQLi建立資料庫連線:包含MySQLi擴充(require_once)建立連線函數(functionconnect_to_db)呼叫連線函數($conn=connect_to_db())執行查詢($result=$conn->query())關閉連線( $conn->close())
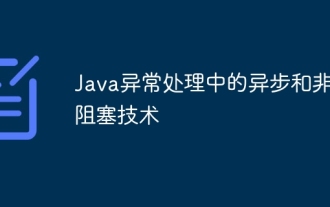
非同步和非阻塞技術可用於補充傳統異常處理,允許創建更具響應性和高效的Java應用程式:非同步異常處理:在另一個執行緒或進程中處理異常,讓主執行緒繼續執行,避免阻塞。非阻塞異常處理:涉及I/O操作出錯時事件驅動的異常處理,避免阻塞線程,由事件循環處理異常。
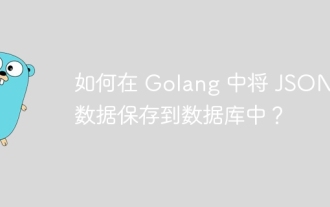
可以透過使用gjson函式庫或json.Unmarshal函數將JSON資料儲存到MySQL資料庫中。 gjson函式庫提供了方便的方法來解析JSON字段,而json.Unmarshal函數需要一個目標類型指標來解組JSON資料。這兩種方法都需要準備SQL語句和執行插入操作來將資料持久化到資料庫中。
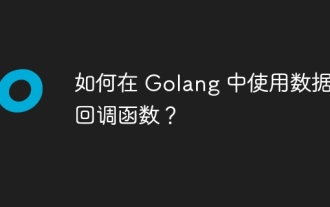
在Golang中使用資料庫回呼函數可以實現:在指定資料庫操作完成後執行自訂程式碼。透過單獨的函數新增自訂行為,無需編寫額外程式碼。回調函數可用於插入、更新、刪除和查詢操作。必須使用sql.Exec、sql.QueryRow或sql.Query函數才能使用回呼函數。
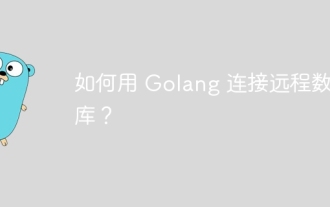
透過Go標準庫database/sql包,可以連接到MySQL、PostgreSQL或SQLite等遠端資料庫:建立包含資料庫連接資訊的連接字串。使用sql.Open()函數開啟資料庫連線。執行SQL查詢和插入操作等資料庫操作。使用defer關閉資料庫連線以釋放資源。
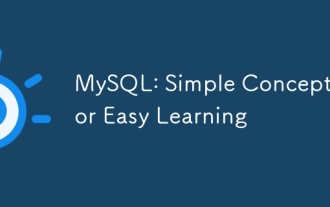
MySQL是一個開源的關係型數據庫管理系統。 1)創建數據庫和表:使用CREATEDATABASE和CREATETABLE命令。 2)基本操作:INSERT、UPDATE、DELETE和SELECT。 3)高級操作:JOIN、子查詢和事務處理。 4)調試技巧:檢查語法、數據類型和權限。 5)優化建議:使用索引、避免SELECT*和使用事務。
