C++技術中的機器學習:使用C++訓練機器學習模型的最佳實踐
在 C++ 中訓練機器學習模型的最佳實踐包括:使用高效的資料結構。優化記憶體管理。利用多線程。整合流行的機器學習庫。專注於程式碼簡潔性。
C++ 技術中的機器學習:訓練機器學習模型的最佳實踐
##引言
C++ 是機器學習領域中一種強大且廣泛使用的程式語言。它提供了出色的性能、記憶體管理和對機器學習庫的存取。本文介紹了在 C++ 中訓練機器學習模型的最佳實踐,包括實戰案例。最佳實務
- 使用高效率的資料結構: 對於大型資料集,使用高效率的資料結構(如Eigen 或Armadillo)對於最佳性能至關重要。
- 優化記憶體管理: C++ 中的手動記憶體管理可以透過消除記憶體洩漏並提高效能來提高效率。
- 利用多線程: C++ 支援多線程,可透過並行運算任務來提升訓練速度。
- 整合流行的機器學習函式庫: TensorFlow、PyTorch 等函式庫提供了豐富的機器學習功能,可以輕鬆整合到 C++ 程式碼中。
- 專注於程式碼簡潔性: 保持程式碼簡潔易讀,以便於維護和協作。
實戰案例:使用 TensorFlow 訓練線性迴歸模型
#以下程式碼片段示範了使用 TensorFlow 在 C++ 中訓練線性迴歸模型:#include <tensorflow/core/framework/tensor.h> #include <tensorflow/core/framework/tensor_shape.h> #include <tensorflow/core/lib/io/path.h> #include <tensorflow/core/public/session.h> using namespace tensorflow; int main() { // 创建会话 Session* session = NewSession(SessionOptions()); // 准备训练数据 float training_data[6][2] = { {1, 1}, {2, 2}, {3, 3}, {4, 4}, {5, 5}, {6, 6} }; float training_labels[6] = {2.0f, 4.0f, 6.0f, 8.0f, 10.0f, 12.0f}; Tensor training_x(DT_FLOAT, TensorShape({6, 2})); Tensor training_y(DT_FLOAT, TensorShape({6})); memcpy(training_x.flat<float>().data(), training_data, sizeof(training_data)); memcpy(training_y.flat<float>().data(), training_labels, sizeof(training_labels)); // 构建模型 GraphDef graph_def; auto status = ReadBinaryProto(Env::Default(), "model.pb", &graph_def); if (!status.ok()) throw std::runtime_error(status.message()); status = session->Create(graph_def); if (!status.ok()) throw std::runtime_error(status.message()); // 训练模型 std::vector<std::pair<string, Tensor>> inputs = { {"x", training_x}, {"y", training_y} }; std::vector<string> outputs = {"loss"}; std::vector<Tensor> out; while (true) { session->Run(inputs, outputs, {}, &out); if (out[0].scalar<float>()() < 0.01) break; } // 保存模型 string output_path = io::JoinPath("saved_model", "export"); if (!io::gfile::Exists(output_path)) io::gfile::MakeDirectories(output_path); status = session->Run({}, {}, {"model"}, &out); if (!status.ok()) throw std::runtime_error(status.message()); const Tensor& saved_model = out[0]; io::gfile::DeleteRecursively(output_path, io::gfile::Recurse::kRecurse); string path = SavedModelUtil::WriteSavedModel(saved_model, output_path); if (!path.empty()) { std::cout << "模型已保存至 " << path << std::endl; } // 清理 session->Close(); delete session; return 0; }
以上是C++技術中的機器學習:使用C++訓練機器學習模型的最佳實踐的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
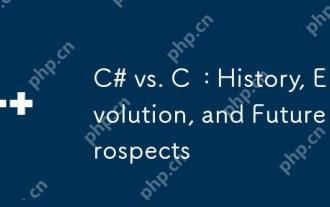
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
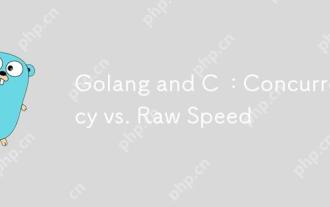
Golang在並發性上優於C ,而C 在原始速度上優於Golang。 1)Golang通過goroutine和channel實現高效並發,適合處理大量並發任務。 2)C 通過編譯器優化和標準庫,提供接近硬件的高性能,適合需要極致優化的應用。
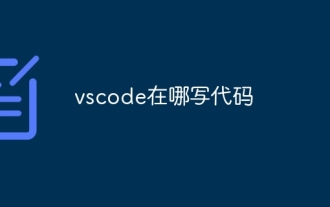
在 Visual Studio Code(VSCode)中編寫代碼簡單易行,只需安裝 VSCode、創建項目、選擇語言、創建文件、編寫代碼、保存並運行即可。 VSCode 的優點包括跨平台、免費開源、強大功能、擴展豐富,以及輕量快速。
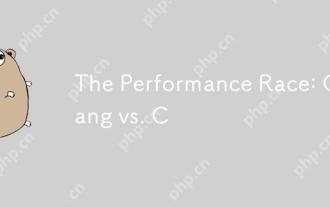
Golang和C 在性能競賽中的表現各有優勢:1)Golang適合高並發和快速開發,2)C 提供更高性能和細粒度控制。選擇應基於項目需求和團隊技術棧。
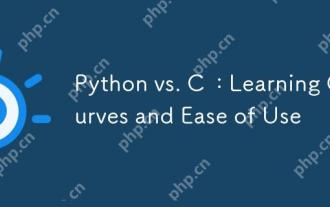
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
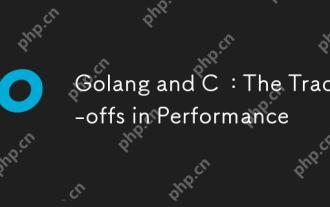
Golang和C 在性能上的差異主要體現在內存管理、編譯優化和運行時效率等方面。 1)Golang的垃圾回收機制方便但可能影響性能,2)C 的手動內存管理和編譯器優化在遞歸計算中表現更為高效。
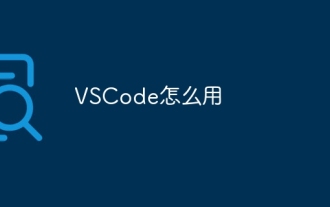
Visual Studio Code (VSCode) 是一款跨平台、開源且免費的代碼編輯器,由微軟開發。它以輕量、可擴展性和對眾多編程語言的支持而著稱。要安裝 VSCode,請訪問官方網站下載並運行安裝程序。使用 VSCode 時,可以創建新項目、編輯代碼、調試代碼、導航項目、擴展 VSCode 和管理設置。 VSCode 適用於 Windows、macOS 和 Linux,支持多種編程語言,並通過 Marketplace 提供各種擴展。它的優勢包括輕量、可擴展性、廣泛的語言支持、豐富的功能和版
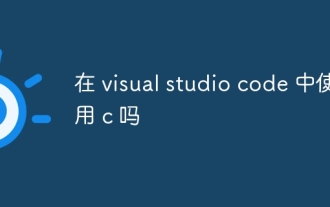
在 VS Code 中編寫 C 語言不僅可行,而且高效優雅。關鍵在於安裝優秀的 C/C 擴展,它提供代碼補全、語法高亮和調試等功能。 VS Code 的調試功能可幫助你快速定位 bug,而 printf 輸出是老式但有效的調試方法。此外,動態內存分配時應檢查返回值並釋放內存以防止內存洩漏,調試這些問題在 VS Code 中很方便。雖然 VS Code 無法直接幫助進行性能優化,但它提供了一個良好的開發環境,便於分析代碼性能。良好的編程習慣、可讀性和可維護性也至關重要。總之,VS Code 是一
