Go WebSocket 如何處理並發連線?
Go WebSocket 處理並發連線的方法:為每個連線使用一個 Goroutine。透過 Channel 進行連線通訊。使用第三方函式庫(如 [gowebsocket](https://github.com/gobwas/ws)、[gorilla/websocket](https://github.com/gorilla/websocket))來簡化處理。
Go WebSocket 如何處理並發連接
WebSocket 是一種全雙工通訊協議,允許客戶端和伺服器進行即時雙向通訊。 Go 語言中處理 WebSocket 並發連線的常用方法如下:
1. Goroutine
一個簡單的解決方案是為每個連線使用一個 Goroutine。 Goroutine 是 Go 中的一種輕量級線程,可以並行執行。當一個新的WebSocket 連線建立時,我們可以建立一個新的Goroutine 來處理它:
package main import ( "fmt" "net/http" "os" "github.com/gorilla/websocket" ) var upgrader = websocket.Upgrader{} func main() { // WebSocket 服务器端口号 port := os.Getenv("PORT") if port == "" { port = "8080" } http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { // 对请求进行 WebSocket 升级 conn, err := upgrader.Upgrade(w, r, nil) if err != nil { fmt.Println(err) return } // 创建一个 Goroutine 处理连接 go handleConnection(conn) }) http.ListenAndServe(":"+port, nil) } // handleConnection 处理一个 WebSocket 连接 func handleConnection(conn *websocket.Conn) { for { msgType, msg, err := conn.ReadMessage() if err != nil { fmt.Println(err) break } if msgType == websocket.TextMessage { // 处理文本消息 fmt.Println("Received text message:", string(msg)) if err := conn.WriteMessage(msgType, msg); err != nil { fmt.Println(err) } } else { // 处理其他类型的消息 } } conn.Close() }
2. Channel
Channel 是Go 中用於通訊的並發通道。我們可以透過一個 Channel 來處理多個連接:
package main import ( "fmt" "net/http" "os" "sync" "time" "github.com/gorilla/websocket" ) var ( upgrader = websocket.Upgrader{} connections = make(chan *websocket.Conn, 100) wg sync.WaitGroup ) func main() { // WebSocket 服务器端口号 port := os.Getenv("PORT") if port == "" { port = "8080" } http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { // 对请求进行 WebSocket 升级 conn, err := upgrader.Upgrade(w, r, nil) if err != nil { fmt.Println(err) return } // 将连接添加到 Channel connections <- conn }) // 启动一个 Goroutine 处理 Channel 中的连接 go handleConnections() http.ListenAndServe(":"+port, nil) } // handleConnections 处理 Channel 中的 WebSocket 连接 func handleConnections() { for { conn := <-connections wg.Add(1) go func() { defer wg.Done() for { msgType, msg, err := conn.ReadMessage() if err != nil { fmt.Println(err) break } if msgType == websocket.TextMessage { // 处理文本消息 fmt.Println("Received text message:", string(msg)) // 向所有连接的客户端广播消息 for c := range connections { if c != conn { if err := c.WriteMessage(msgType, msg); err != nil { fmt.Println(err) } } } } else { // 处理其他类型的消息 } } conn.Close() }() } }
透過管道傳遞連接可以在所有 Goroutine 之間共享連接,並避免創建過多線程帶來的開銷。
3. 第三方函式庫
還有許多第三方函式庫可以簡化WebSocket 並發處理,例如:
- [gowebsocket]( https://github.com/gobwas/ws)
- [gorilla/websocket](https://github.com/gorilla/websocket)
- [fasthttp/websocket](https: //github.com/valyala/fasthttp/blob/master/websocket/websocket.go)
這些函式庫提供了高層次的API 來處理並發連接,並簡化了WebSocket 的使用。
以上是Go WebSocket 如何處理並發連線?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
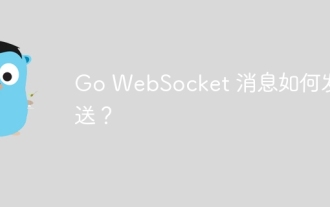
在Go中,可以使用gorilla/websocket包發送WebSocket訊息。具體步驟:建立WebSocket連線。傳送文字訊息:呼叫WriteMessage(websocket.TextMessage,[]byte("訊息"))。發送二進位訊息:呼叫WriteMessage(websocket.BinaryMessage,[]byte{1,2,3})。
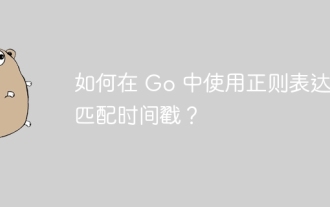
在Go中,可以使用正規表示式比對時間戳記:編譯正規表示式字串,例如用於匹配ISO8601時間戳記的表達式:^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ 。使用regexp.MatchString函數檢查字串是否與正規表示式相符。
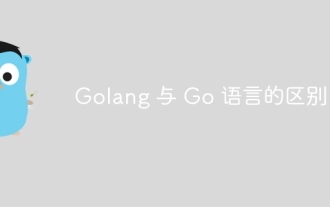
Go和Go語言是不同的實體,具有不同的特性。 Go(又稱Golang)以其並發性、編譯速度快、記憶體管理和跨平台優點而聞名。 Go語言的缺點包括生態系統不如其他語言豐富、文法更嚴格、缺乏動態類型。
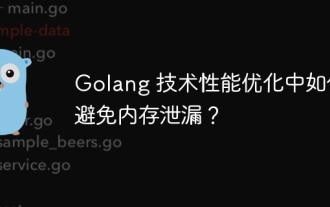
記憶體洩漏會導致Go程式記憶體不斷增加,可通過:關閉不再使用的資源,如檔案、網路連線和資料庫連線。使用弱引用防止記憶體洩漏,當物件不再被強引用時將其作為垃圾回收目標。利用go協程,協程棧記憶體會在退出時自動釋放,避免記憶體洩漏。
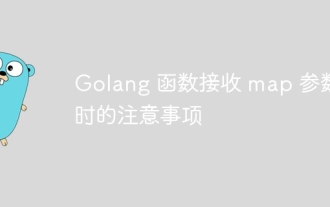
在Go中傳遞map給函數時,預設會建立副本,對副本的修改不影響原map。如果需要修改原始map,可透過指標傳遞。空map需小心處理,因為技術上是nil指針,傳遞空map給期望非空map的函數會發生錯誤。
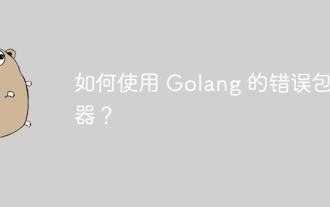
在Golang中,錯誤包裝器允許你在原始錯誤上追加上下文訊息,從而創建新錯誤。這可用於統一不同程式庫或元件拋出的錯誤類型,簡化偵錯和錯誤處理。步驟如下:使用errors.Wrap函數將原有錯誤包裝成新錯誤。新錯誤包含原始錯誤的上下文資訊。使用fmt.Printf輸出包裝後的錯誤,提供更多上下文和可操作性。在處理不同類型的錯誤時,使用errors.Wrap函數統一錯誤類型。
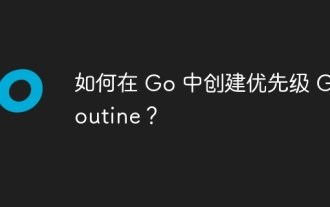
在Go語言中建立優先權Goroutine有兩步驟:註冊自訂Goroutine建立函數(步驟1)並指定優先權值(步驟2)。這樣,您可以建立不同優先順序的Goroutine,優化資源分配並提高執行效率。
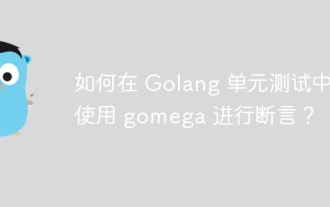
如何在Golang單元測試中使用Gomega進行斷言在Golang單元測試中,Gomega是一個流行且功能強大的斷言庫,它提供了豐富的斷言方法,使開發人員可以輕鬆驗證測試結果。安裝Gomegagoget-ugithub.com/onsi/gomega使用Gomega進行斷言以下是使用Gomega進行斷言的一些常用範例:1.相等斷言import"github.com/onsi/gomega"funcTest_MyFunction(t*testing.T){
