PHP物件導向程式設計的深入理解:物件導向程式設計的擴展與客製化
PHP 物件導向程式設計可透過擴充和客製化類別實現擴充。擴充類別透過繼承父類別屬性和方法,並可新增屬性和方法;自訂類別則透過實作介面的方法來實作特定功能。在實戰案例中,透過擴展抽象類別 Shape,創建了 Circle 和 Rectangle 等具體形狀,可動態計算面積。
PHP 物件導向程式設計:擴充與客製化
物件導向程式設計(OOP) 可讓您建立可重複使用、可維護的代碼。在 PHP 中,OOP 可以透過擴展和自訂現有類別來進一步擴展。
擴充類別
使用 extends
關鍵字可以擴充一個類別。擴展後的類別繼承父類別的所有屬性和方法,並可以新增屬性和方法。
class BaseClass { protected $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } class ExtendedClass extends BaseClass { private $age; public function __construct($name, $age) { parent::__construct($name); $this->age = $age; } public function getAge() { return $this->age; } }
自訂類別
使用 implements
關鍵字可以自訂一個類,讓它實作一個或多個介面。介面定義了一組方法,該類別必須實作這些方法。
interface MyInterface { public function doSomething(); } class MyClass implements MyInterface { public function doSomething() { // 具体实现 } }
實戰案例
考慮一個抽象類別 Shape
,它定義了一個 getArea()
方法。我們擴展此類以創建具體形狀,例如 Circle
和 Rectangle
。
abstract class Shape { protected $color; public function __construct($color) { $this->color = $color; } abstract public function getArea(); } class Circle extends Shape { private $radius; public function __construct($color, $radius) { parent::__construct($color); $this->radius = $radius; } public function getArea() { return pi() * $this->radius ** 2; } } class Rectangle extends Shape { private $width; private $height; public function __construct($color, $width, $height) { parent::__construct($color); $this->width = $width; $this->height = $height; } public function getArea() { return $this->width * $this->height; } }
我們可以建立 Circle
和 Rectangle
物件並存取它們各自的 getArea()
方法,從而動態地計算面積。
以上是PHP物件導向程式設計的深入理解:物件導向程式設計的擴展與客製化的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
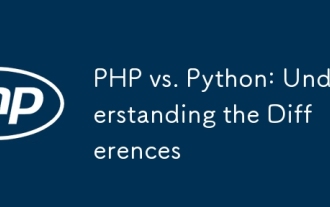
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
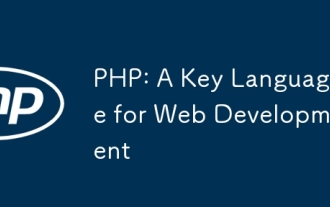
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
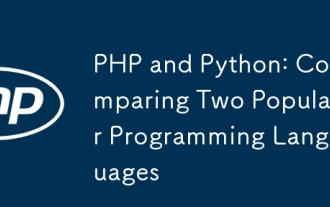
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
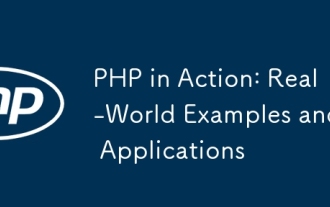
PHP在電子商務、內容管理系統和API開發中廣泛應用。 1)電子商務:用於購物車功能和支付處理。 2)內容管理系統:用於動態內容生成和用戶管理。 3)API開發:用於RESTfulAPI開發和API安全性。通過性能優化和最佳實踐,PHP應用的效率和可維護性得以提升。
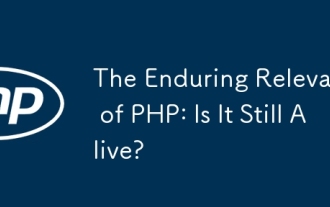
PHP仍然具有活力,其在現代編程領域中依然佔據重要地位。 1)PHP的簡單易學和強大社區支持使其在Web開發中廣泛應用;2)其靈活性和穩定性使其在處理Web表單、數據庫操作和文件處理等方面表現出色;3)PHP不斷進化和優化,適用於初學者和經驗豐富的開發者。
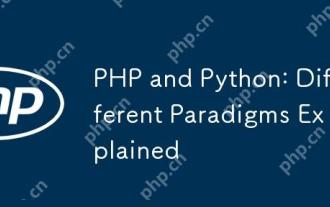
PHP主要是過程式編程,但也支持面向對象編程(OOP);Python支持多種範式,包括OOP、函數式和過程式編程。 PHP適合web開發,Python適用於多種應用,如數據分析和機器學習。
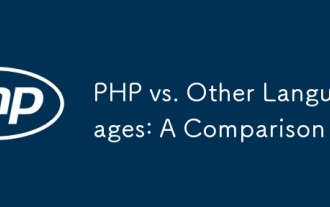
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
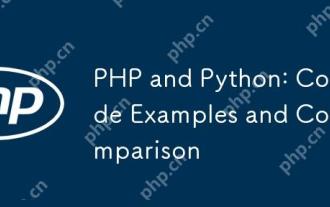
PHP和Python各有優劣,選擇取決於項目需求和個人偏好。 1.PHP適合快速開發和維護大型Web應用。 2.Python在數據科學和機器學習領域佔據主導地位。
