像 Typescript 一樣寫 Python 程式碼
我想想讀這篇文章的人都知道什麼是 typescript。 Javascript 開發人員創建了 Typescript 來讓 javascript 更安全。類型安全性使程式碼更具可讀性,並且無需編寫任何測試即可減少錯誤。 python 可以實現型別安全嗎? .
為什麼我們需要類型安全?
想像一下這個看似無辜的函數
def send_email(sender, receiver, message): ...
我故意隱藏了它的程式碼實作。您能猜出函數的用途是什麼以及我們需要什麼參數才能僅透過函數名稱和參數來使用該函數嗎?從它的函數名稱我們知道它的功能是發送電子郵件。它的參數怎麼樣,我們該輸入什麼才能使用這個函數?
首先猜測寄件者是電子郵件的 str,收件者是電子郵件的 str,訊息是電子郵件正文的 str。
send_email(sender="john@mail.com", receiver="doe@mail.com", message="Hello doe! How are you?")
最簡單的猜測。但這不是唯一的猜測。
第二個猜測傳送者是資料庫上 user_id 的 int,接收者是資料庫上 user_id 的 int,訊息是電子郵件正文的 str。
john_user_id = 1 doe_user_id = 2 send_email(sender=1, receiver=2, message="Hello doe! How are you?")
想像一下在應用程式上工作。大多數應用程式使用一些資料庫。使用者通常用它的 id 來表示。
第三個猜測發送者是字典,接收者是字典,訊息是字典。
john = { "id": 1, "username": "john", "email": "john@mail.com" } doe = { "id": 2, "username": "doe", "email": "doe@mail.com" } message = { "title": "Greeting my friend doe", "body": "Hello doe! How are you?" } send_email(sender=john, receiver=doe, message=message)
也許 send_email 需要的不只是電子郵件和使用者 ID。為了在每個參數上添加更多數據,它使用了一些字典結構。您注意到該訊息不僅僅是字串,也許還需要標題和正文。
第四次猜測發送者是 User 類,接收者是 User 類,訊息是字典。
class User(): def __init__(self, id, username, email): self.id = id self.username = username self.email = email john = User(id=1, username="john", email="john@mail.com") doe = User(id=2, username="doe", email="doe@mail.com") message = { "title": "Greeting my friend doe", "body": "Hello doe! How are you?" } send_email(sender=john, receiver=doe, message=message)
也許send_email 與一些資料庫orm 集成,如Django ORM 或Sqlalchemy。為了方便最終用戶使用,直接使用ORM類別。
那麼哪一個才是正確答案呢?其中之一可能是正確答案。也許正確答案可以是兩個猜測的結合。就像發送者和接收者一樣,它是 User 類別(第四次猜測),但訊息是 str(第一次猜測)。除非我們閱讀它的程式碼實現,否則我們無法確定。如果您是最終用戶,這就是浪費時間。作為使用此函數的最終用戶,我們只需要函數做什麼、需要什麼參數以及函數輸出是什麼。
解決方案
文件字串
Python 使用文件字串內建了功能文件。這裡是文檔字串範例。
def add(x, y): """Add two number Parameter:\n x -- int\n y -- int\n Return: int """ return x + y def send_email(sender, receiver, message): """Send email from sender to receiver Parameter:\n sender -- email sender, class User\n receiver -- email receiver, class User\n message -- body of the email, dictionary (ex: {"title": "some title", "body": "email body"}\n Return: None """ ...
文件字串的優點是它與編輯器相容。在 vscode 中,當您將滑鼠懸停在函數上時,它將顯示文件字串。 Python 中的大多數函式庫都使用文件字串來記錄其功能。
文檔字串的問題是文檔同步。如何確保文件字串始終與程式碼實作同步。你無法正確地測試它。我從網路上隨機的人那裡聽說「擁有過時的文件比沒有文件更糟糕」。
文檔測試
順便說一句,您可以使用 doctest 來測試文件字串。 Doctest 透過在文件字串上執行範例來測試您的文件字串。 Doctest 已預先安裝在 python 中,因此您不需要外部相依性。讓我們來看看這個範例,建立一個名為 my_math.py 的新文件,然後放入此程式碼。
# my_math.py def add(x, y): """Add two integer Parameter:\n x -- int\n y -- int\n Return: int >>> add(1, 2) 3 """ return x + y if __name__ == "__main__": import doctest doctest.testmod()
它的程式碼與 docstring example 相同,但我在程式碼的最後一行加入了 example 和 doctest 。為了測試文件字串,只需運行文件 python my_math.py。如果沒有輸出,則表示您的範例通過了測試。如果您想查看輸出,請在詳細模式下執行 python my_math.py -v,您將看到此輸出。
Trying: add(1, 2) Expecting: 3 ok 1 items had no tests: __main__ 1 items passed all tests: 1 tests in __main__.add 1 tests in 2 items. 1 passed and 0 failed. Test passed
如果您在程式碼範例中犯錯,它將傳回錯誤。
# my_math.py def add(x, y): """Add two integer Parameter:\n x -- int\n y -- int\n Return: int >>> add(2, 2) # <-- I change it here 3 """ return x + y if __name__ == "__main__": import doctest doctest.testmod()
輸出:
********************************************************************** File "~/typescript-in-python/my_math.py", line 12, in __main__.add Failed example: add(2, 2) # <-- I change it here Expected: 3 Got: 4 ********************************************************************** 1 items had failures: 1 of 1 in __main__.add ***Test Failed*** 1 failures.
太棒了!現在我可以測試我的文檔字串了。但注意事項是:
- doctest 只檢查範例它不檢查註解函數參數和回傳
- doctest需要執行程式碼像其他測試工具一樣,以檢查它是否正確 doctest需要執行程式碼範例。如果您的程式碼需要一些外部工具,例如資料庫或 smtp 伺服器(例如傳送電子郵件),則很難使用 doctest 進行測試。
Python 打字
有時你不需要執行程式碼來檢查程式碼是否正確。您只需要輸入類型和輸出類型。如何?考慮這個例子。
def add(x, y): """Add two integer Parameter:\n x -- int\n y -- int\n Return: int """ return x + y def sub(x, y): """Substract two integer Parameter:\n x -- int\n y -- int\n Return: int """ return x - y a = add(2, 1) b = add(1, 1) c = sub(a, b)
函數add傳回一個int,函數sub需要兩個int作為輸入參數。如果我使用添加函數的兩個返回值,然後將其放在子參數上,如上面的範例,它會出錯嗎?當然不是因為 sub 函數需要 int 而你也輸入了 int。
從 python 3.5 開始,python 內建了稱為打字的類型。透過輸入,您可以在函數上新增類型,如下例所示。
def add(x: int, y: int) -> int: """Add two integer""" return x + y a = add(1, 2)
Instead put it on your docstring you put it on the function. Typing is supported on many editor. If you use vscode you can hover on variable and it will shown it's type.
Nice now our code will have a type safety. eeehhhh not realy. If I intentionally use function incorrectlly like this.
def add(x: int, y: int) -> int: """Add two integer""" return x + y res = add(1, []) print(res)
It will show error
Traceback (most recent call last): File "~/typescript-in-python/main.py", line 5, in <module> res = add(1, []) ^^^^^^^^^^ File "~/typescript-in-python/main.py", line 3, in add return x + y ~~^~~ TypeError: unsupported operand type(s) for +: 'int' and 'list'
But it doesn't show that you put incorrect type. Even worse if you use it like this.
def add(x: int, y: int) -> int: """Add two integer""" return x + y res = add("hello", "world") print(res)
It will succeed. It must be error because you put incorrect type.
helloworld
Why python typing doesn't have type checker by default??. Based on pep-3107 it said
Before launching into a discussion of the precise ins and outs of Python 3.0’s function annotations, let’s first talk broadly about what annotations are and are not:
- Function annotations, both for parameters and return values, are completely optional.
- Function annotations are nothing more than a way of associating arbitrary Python expressions with various parts of a function at compile-time. By itself, Python does not attach any particular meaning or significance to annotations. Left to its own, Python simply makes these expressions available as described in Accessing Function Annotations below.
The only way that annotations take on meaning is when they are interpreted by third-party libraries. ...
So in python typing is like a decorator in typescript or java it doesn't mean anything. You need third party libraries todo type checking. Let's see some library for typechecking.
Python typing + type checker
Here are libraries for typechecking in python. For example we will typecheck this wrong.py file
def add(x: int, y: int) -> int: """Add two integer""" return x + y res = add("hello", "world") print(res)
1.mypy
The "OG" of python type checker. To install it just using pip pip install mypy. Now let's use mypy to typecheck this file. Run mypy wrong.py. It will shown type error which is nice.
wrong.py:5: error: Argument 1 to "add" has incompatible type "str"; expected "int" [arg-type] wrong.py:5: error: Argument 2 to "add" has incompatible type "str"; expected "int" [arg-type] Found 2 errors in 1 file (checked 1 source file)
btw you can run mypy on entire project by using mypy ..
2.pyright
Another typechecker is pyright. It created by microsoft. It's same like mypy install through pip pip install pyright. Then run it pyright wrong.py. It will shown this error.
~/typescript-in-python/wrong.py ~/typescript-in-python/wrong.py:5:11 - error: Argument of type "Literal['hello']" cannot be assigned to parameter "x" of type "int" in function "add" "Literal['hello']" is incompatible with "int" (reportArgumentType) ~/typescript-in-python/wrong.py:5:20 - error: Argument of type "Literal['world']" cannot be assigned to parameter "y" of type "int" in function "add" "Literal['world']" is incompatible with "int" (reportArgumentType) 2 errors, 0 warnings, 0 informations
It said that it's more faster than mypy but I found that's not much diffrent. Maybe my code base it's to small. Also pyright implement more python standard than mypy you can see on https://microsoft.github.io/pyright/#/mypy-comparison. Personaly I prefer mypy than pyright because the error message were more readable.
3.pylyzer
Speaking of performance and speed another new python typechecker pylyzer. It's written in rust. You can install it through pip pip install pylyzer or through cargo (rust package manager) cargo install pylyzer --locked. Then run it pylyzer wrong.py. It will shown this error.
Start checking: wrong.py Found 2 errors: wrong.py Error[#2258]: File wrong.py, line 5, <module>.res 5 | res = add("hello", "world") : ------- : |- expected: Int : `- but found: {"hello"} TypeError: the type of add::x (the 1st argument) is mismatched Error[#2258]: File wrong.py, line 5, <module>.res 5 | res = add("hello", "world") : ------- : |- expected: Int : `- but found: {"world"} TypeError: the type of add::y (the 2nd argument) is mismatched
So far this is the most readable and beautiful error message. It's reminds me of rust compiler error. Speed, performance and most readable error message, I think I will choose to using pylyzer if the package already stable. The problem is at the time I write this blog, pylyzer still in beta. It can only typecheck your code base, it haven't support external depedencies.
Conclusion
Alright we successfully write python code like typescript (kinda). There is more way to using python typing module other than check simple type (str, int, bool etc). Maybe I will cover more advance type it in next blog. Maybe you guys have opinion about this, know better typechecker other then those 3, found other way to do typecheck in python or other. let me know on comment section below. As always Happy Coding.
以上是像 Typescript 一樣寫 Python 程式碼的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
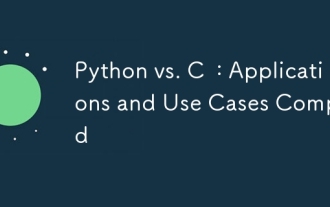
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
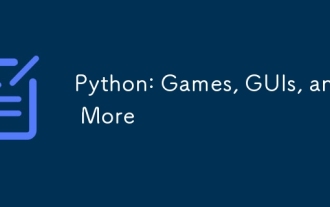
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
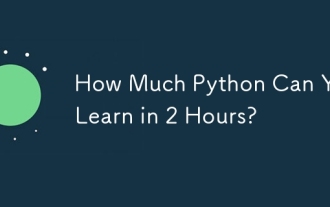
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
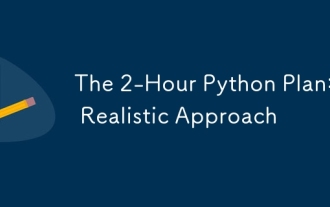
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
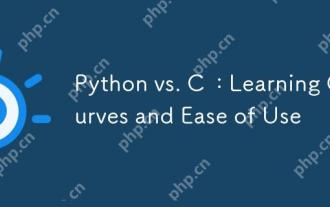
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
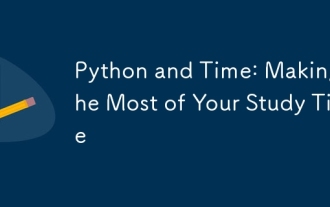
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
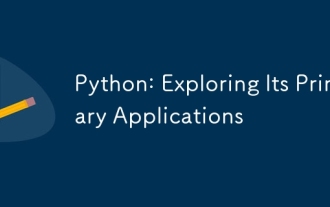
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
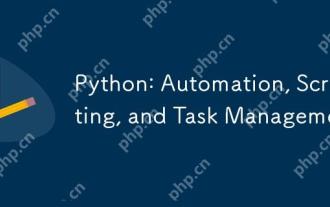
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
