注意:最初發佈在我的子堆疊中:https://open.substack.com/pub/andresalvareziglesias/p/speeding-up-python-code-with-c-and
Python 不是速度的典範,我們都知道這一點。但在我們的好老朋友 C 的幫助下,我們可以加快應用程式的一些關鍵部分的速度。
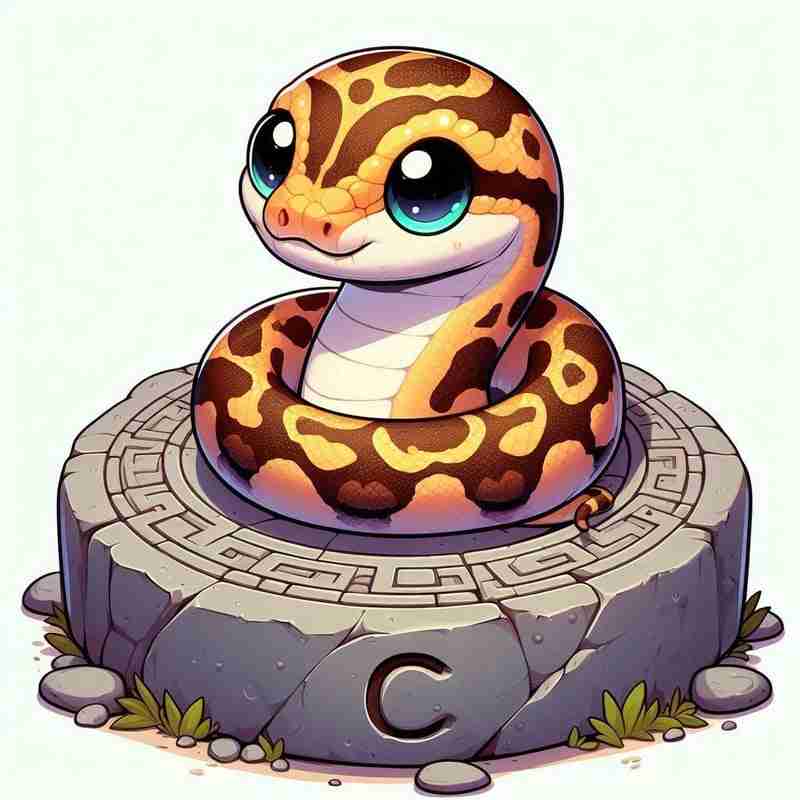
簡單 Python 中的斐波那契數列
斐波那契數列是用來教導軟體開發的經典範例。是以 0 和 1 開頭的一系列數字。後續的每個數字都是前兩個數字的總和。所以,序列是這樣的:0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ...
我們可以這樣用Python開發斐波那契:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | import time
# Configure iterations
iterations = 30
# Define fibonacci in native python
def fibonacci(n):
if n <= 1:
return n
else :
return fibonacci(n-1) + fibonacci(n-2)
# Calculate in pure python
start_time = time.perf_counter()
print (f "Calculating {iterations} iterations of fibonacci..." )
print (fibonacci(iterations))
end_time = time.perf_counter()
execution_time_ms = (end_time - start_time) * 1000
print (f "Execution time: {execution_time_ms:.2f} milliseconds" )
print ()
|
登入後複製
如果我們執行這個純 Python(在 Google IDX 虛擬機器中)版本的斐波那契,我們會得到:
- 10 次迭代:5.77 毫秒
- 30 次迭代:984.36 毫秒
- 50 次迭代:(我必須取消該過程,太多時間)
C語言中的斐波那契數列
我們可以用純 C 語言發展相同的序列:
1 2 3 4 5 6 7 8 9 | # include <stdio.h>
int fibonacci(int n) {
if (n <= 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
|
登入後複製
使用 GCC 編譯函式庫:
1 | gcc -o fibonacci.so -shared -fPIC -O2 fibonacci.c
|
登入後複製
現在,我們有了一個本地二進位函式庫,裡面有斐波那契序列函數。我們可以使用 ctypes(Python C 類型庫,因為 Python 本身是用 C 開發的)將此庫嵌入到 Python 應用程式中:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | import time
from ctypes import c_double, c_int, CDLL
# Configure iterations
iterations = 30
# Import the C library
library = CDLL( './fibonacci.so' )
fibonacciAsLibrary = library.fibonacci
fibonacciAsLibrary.restype = c_int
# Calculate as C library
start_time = time.perf_counter()
print (f "Calculating {iterations} iterations of fibonacci as C library..." )
print (fibonacciAsLibrary(iterations))
end_time = time.perf_counter()
execution_time_ms = (end_time - start_time) * 1000
print (f "Execution time: {execution_time_ms:.2f} milliseconds" )
print ()
|
登入後複製
現在,如果我們在斐波那契上執行這個版本,我們會得到:
- 10 次迭代:0.54 毫秒
- 30 次迭代:6.92 毫秒
- 50 次迭代:82324.90 毫秒
更好,不是嗎?
Python 和 C 整合的用例
我們可以在許多應用程式和場景中使用這種集成,例如:
- 加快 Django 應用程式中的序列化器和反序列化器
- 加快工作流程中的關鍵部分
- 與作業系統的低階互動
- 等等
你呢?您將如何在您的專案中使用這個小技巧?我很想聽聽您的意見!
關於名單
在 Python 和 Docker 貼文中,我也會撰寫其他相關主題(總是技術和程式主題,我保證...祈禱),例如:
如果您發現了一些有趣的技術、程式語言或其他什麼,請告訴我!我總是樂於學習新事物!
關於作者
我是 Andrés,一位來自帕爾馬的全端軟體開發人員,我正在踏上提高編碼技能的個人旅程。我也是一位自行出版的奇幻作家,以我的名字出版了四本小說。有什麼問題都可以問我!
以上是使用 C 加速 Python 程式碼(無需額外的函式庫)的詳細內容。更多資訊請關注PHP中文網其他相關文章!