Java中的File類別在保存應用程式的路徑和以目錄格式管理各種檔案方面起著非常重要的作用。此外,此方法用於依過於抽象的順序或層次結構管理和版本化路徑。使用任何字串命名任何檔案不足以提及應用程式的路徑。在不同平台上執行應用程式並嘗試使用一個或多個屬性來綁定和編譯自身。 File 類別由各種方法組成,這使 java 可以靈活地建立文件、重新命名文件和刪除文件。
開始您的免費軟體開發課程
網頁開發、程式語言、軟體測試及其他
語法與參數:
File abslt = new File("https://cdn.educba.com/usr/local/bin/at.txt");
登入後複製
語法流程的工作方式如下:
-
檔案: 表示執行操作時考慮該檔案。
-
abslt: 它表示檔案名,可以是與檔案相關的任何內容,即包含一些內容或值。
-
new File(): 建立與文件相關的對象,或者可以說是文件對象,它可以包含有關文件值的相關資訊並稍後檢索值。
-
(“/usr/local/bin/at.txt”): 傳遞的參數建議為絕對路徑。將相對路徑作為參數傳遞並不是非常推薦的方法。
檔案類別在 Java 中如何運作?
Java 中的檔案類別的工作方式是類別中的檔案將包含一些稍後需要的內容。稍後可以藉助文件物件來檢索和修改它們。由於這些檔案以不同名稱和路徑名的層次結構進行維護,因此這是不夠的,建議僅使用字串作為名稱。它是所維護的檔案和目錄路徑名的抽象表示。
路徑名可以是絕對路徑名或相對路徑名,取決於使用者規格。如果是絕對路徑,那麼這是一個非常推薦的方法,因為它有助於將來檢索時獲取絕對路徑中提到的指定文件。因此,實際工作首先從建立檔案類別開始,然後建立相應的對象,並使用該物件傳遞檔案名稱和目錄名稱。檔案系統有時會禁止可訪問性或在物件可訪問性時提供限制,這是由於實際檔案系統物件上已經存在的約束而引起的。這給讀、寫和執行等操作造成了障礙。由於文件類別創建的實例是不可變的,因此一旦創建,它們就無法更改路徑名,並且文件物件的表示也不會更改。
建構子
Java 的 File 類別傳遞並經常使用一些建構子:
-
File(File Parent, String child): 檔案父參數負責從建立的檔案物件呼叫抽象路徑名,字串子參數負責從檔案中呼叫子路徑名字串作為檔案的新實例所建立的物件。
-
檔案(URI uri):當給定檔案的 URI 轉換為抽象路徑名時,將建立檔案的新實例。
-
檔案(字串父級,字串子級):從父路徑名建立檔案的新實例,並將子路徑名字串作為參數傳遞給檔案。
-
檔案(字串路徑名):一旦路徑名字串轉換為抽象路徑名,就會建立檔案的新實例。
方法
方法如下:
-
boolean createNewFile(): The abstract pathname passed as an argument to the method is responsible for creating a new empty file.
-
int compareTo(File pathname): When any two pathnames are arranged in lexical order, this method is used to compare both the files and then create a new instance of the file.
-
boolean canExecute(): The abstract pathname parameter passed as an argument is responsible for testing whether the application can execute and function properly.
-
boolean canRead(): The abstract pathname parameter passed as an argument is responsible for testing whether the application can read the passed file.
-
boolean canWrite(): The abstract pathname parameter passed as an argument is responsible for testing whether the application can write some content on the passed file.
-
String getAbsolutePath(): The abstract pathname passed as a parameter for creating an instance returns the abstract pathname string.
-
boolean exists(): This method is responsible for verifying whether the abstract pathname exists.
-
boolean delete(): This method deletes the file or the directory having the contents which is pointed with this abstract pathname.
-
static File createTempFile(String prefix, String suffix): This method is used for creating an empty temporary file or directory by default.
-
boolean equals(Object obj): The file object created as part of the abstract pathname is responsible for verifying the equality with the given object.
-
String[] list(): Files present in the directory or file system gets returned in the form of an array as strings.
-
long length(): The abstract pathname denoted by the file object is responsible for getting the length of the file.
-
boolean isHidden(): This method is responsible for testing whether the abstract pathname names the file is a hidden file.
-
boolean isFile(): This method is responsible for testing whether the file returned is a normal file or is denoted by the abstract pathname object.
-
boolean isDirectory(): This method is responsible for testing whether the file denoted by this abstract pathname is a directory or not.
-
boolean mkdir(): This method is responsible for creating the directory named by passing the abstract pathname.
-
File[] listFiles(): All the files present in the directory is responsible for returning an array of abstract pathnames as denoted by the object.
-
boolean setReadable(boolean readable): It is used for setting the owner’s or user’s permission for reading the content present in the file.
-
boolean renameTo(File dest): The file represented by the abstract pathname is responsible for the renaming of the file.
-
boolean setExecutable(boolean executable): This method is used for setting the owner’s execution permission.
-
boolean setReadable(boolean readable, boolean ownerOnly): This method is responsible for setting up the constraint owner’s readability permission.
-
URI toURI(): Representation of this abstract pathname is done by constructing the URI file.
-
String toString(): This method is responsible for returning the string pathname of the abstract pathname.
-
boolean setReadOnly(): This method is used only to perform the read operations exclusively.
-
boolean setWritable(boolean writable): This method is used for setting up the write permission for the owner even at the time of execution.
Examples
Now we will learn about the Java File and its various operations with the help of examples.
Example #1
This program is used for demonstrating the creation of a new file and return the file is already present if it exists already.
Code:
import java.io.File;
import java.io.IOException;
public class FilePropTest {
public static void main(String[] args) {
try {
File file = new File("java_File.txt");
if (file.createNewFile()) {
System.out.println("Created a new file");
} else {
System.out.println("File is already present ");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
登入後複製
Output:
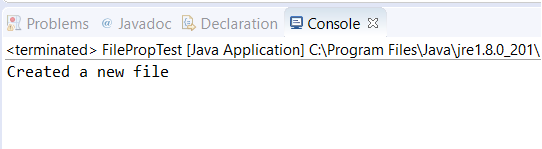
Example #2
This program is used to demonstrate the filenames being passed as an absolute pathname and retrieving all the filenames within the directory of the drive.
Code:
import java.io.File;
public class FilePropTest2 {
public static void main(String[] args) {
File fil_nm=new File("C:/Users/adutta/documents");
String all_file_names[]=fil_nm.list();
for(String enlist_filename:all_file_names){
System.out.println(enlist_filename);
}
}
}
登入後複製
Output:
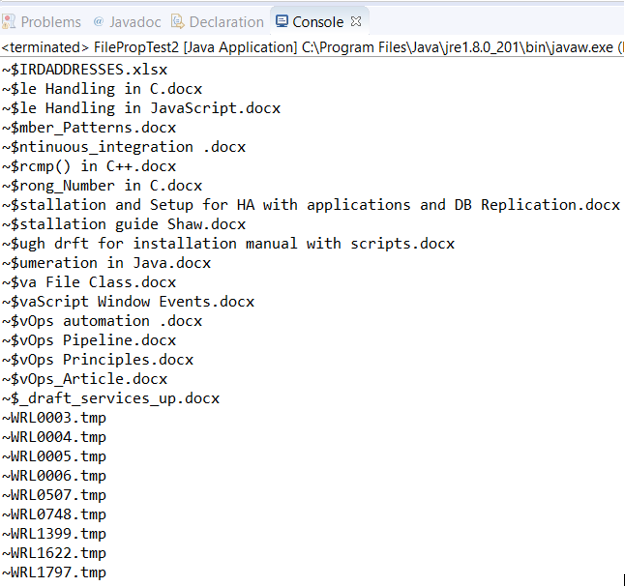
注意:所有解釋和定義的方法都可以透過這種方式演示,並且只需呼叫 Java 檔案類別中已有的方法名稱即可執行。
結論 – Java 檔案類別
Java 中的文件類是一個非常有用的類,因為它不包含任何外部規範,但包含作為文件包的一部分存在的方法,並且可以無縫地用於執行任何與文件相關的活動,包括取得檔案名稱並分別檢索文件。因此,File 類別為方法和所有其他文件提供了很大的靈活性。
以上是Java檔案類的詳細內容。更多資訊請關注PHP中文網其他相關文章!