在 Golang 中安全使用 Map:宣告和初始化的差異
介紹
本週,我正在為 golang 開發一個 API 包裝器包,它處理發送帶有 URL 編碼值的 post 請求、設定 cookie 以及所有有趣的東西。但是,當我建立主體時,我使用 url.Value 類型來建立主體,並使用它來新增和設定鍵值對。然而,我在某些部分遇到了有線零指標引用錯誤,我認為這是因為我手動設定的一些變數造成的。然而,透過仔細調試,我發現了一個常見的陷阱或不好的做法,即僅聲明類型但初始化它,從而導致零引用錯誤。
在這篇文章中,我將介紹什麼是映射、如何建立映射,特別是如何正確宣告和初始化它們。在 golang 中的對應或任何類似資料類型的宣告和初始化之間建立適當的區別。
Golang 中的地圖是什麼?
golang中的map或hashmap是一種基本資料類型,允許我們儲存鍵值對。在底層,它是一個類似標頭映射的資料結構,用於保存儲存桶,這些儲存桶基本上是指向儲存桶數組(連續記憶體)的指標。它具有儲存實際鍵值對的雜湊碼,以及在當前鍵數量溢出時指向新儲存桶的指標。這是一個非常聰明的資料結構,提供幾乎恆定的時間存取。
如何在 Golang 中建立地圖
要在 golang 中建立一個簡單的映射,您可以使用字串和整數的映射作為字母頻率計數器的範例。地圖會將字母儲存為鍵,將它們的頻率儲存為值。
因此,透過將地圖初始化為map[string]int{},您將得到一個空地圖。然後,這可以用於填充鍵和值,我們迭代字串,對於每個字元(符文),我們將該字元位元組轉換為字串並遞增值,int 的零值為0,因此預設情況下如果密鑰不存在,則其值為零,但這是一把雙刃劍,我們永遠不知道地圖中存在值為0 的密鑰,或者密鑰不存在但預設值為0。為此,您需要檢查該密鑰是否存在於地圖中。
更多詳情,您可以查看我的 Golang Maps 貼文詳細內容。
聲明和初始化之間的區別
在程式語言中宣告和初始化任何變數都是不同的,並且必須在底層類型的實作上做更多的事情。對於 int、string、float 等主要資料類型,有預設值/零值,因此這與變數的宣告和初始化相同。然而,在映射和切片的情況下,聲明只是確保變數可用於程式的範圍,但是對於初始化來說,是將其設定為其預設值/零值或應分配的實際值。
因此,宣告只是使變數在程式範圍內可用。對於映射和切片,聲明變數而不初始化會將其設為 nil,這意味著它指向沒有分配的內存,並且不能直接使用。
而初始化會分配記憶體並將變數設為可用狀態。對於映射和切片,您需要使用 myMap = make(map[keyType]valueType) 或 slice = []type{} 等語法明確初始化它們。如果沒有此初始化,嘗試使用映射或切片將導致運行時錯誤,例如在存取或修改 nil 映射或切片時出現恐慌。
讓我們看看宣告/初始化/未初始化時映射的值。
假設您正在建立一個從地圖讀取設定的組態管理員。地圖將在全域聲明,但僅在載入配置時初始化。
- 已宣告但未初始化
下面的程式碼演示了未初始化的地圖訪問。
- 同時宣告與初始化
下面的程式碼示範了同時初始化的地圖存取。
- 宣告並稍後初始化
下面的程式碼示範了稍後初始化的地圖訪問。
package main import ( "fmt" "log" ) // Global map to store configuration settings var configSettings map[string]string // declared but not initialized func main() { // Initialize configuration settings initializeConfigSettings() // if the function is not called, the map will be nil // Get a configuration setting safely serverPort := getConfigSetting("server_port") fmt.Printf("Server port: %s\n", serverPort) } func initializeConfigSettings() { if configSettings == nil { configSettings = make(map[string]string) // Properly initialize the map configSettings["server_port"] = "8080" configSettings["database_url"] = "localhost:5432" fmt.Println("Configuration settings initialized") } } func getConfigSetting(key string) string { if configSettings == nil { log.Fatal("Configuration settings map is not initialized") } value, exists := configSettings[key] if !exists { return "Setting not found" } return value }
$ go run main.go Configuration settings initialized Server port: 8080
In the above code, we declared the global map configSettings but didn't initialize it at that point, until we wanted to access the map. We initialize the map in the main function, this main function could be other specific parts of the code, and the global variable configSettings a map from another part of the code, by initializing it in the required scope, we prevent it from causing nil pointer access errors. We only initialize the map if it is nil i.e. it has not been initialized elsewhere in the code. This prevents overriding the map/flushing out the config set from other parts of the scope.
Pitfalls in access of un-initialized maps
But since it deals with pointers, it comes with its own pitfalls like nil pointers access when the map is not initialized.
Let's take a look at an example, a real case where this might happen.
package main import ( "fmt" "net/url" ) func main() { var vals url.Values vals.Add("foo", "bar") fmt.Println(vals) }
This will result in a runtime panic.
$ go run main.go panic: assignment to entry in nil map goroutine 1 [running]: net/url.Values.Add(...) /usr/local/go/src/net/url/url.go:902 main.main() /home/meet/code/playground/go/main.go:10 +0x2d exit status 2
This is because the url.Values is a map of string and a list of string values. Since the underlying type is a map for Values, and in the example, we only have declared the variable vals with the type url.Values, it will point to a nil reference, hence the message on adding the value to the type. So, it is a good practice to use make while declaring or initializing a map data type. If you are not sure the underlying type is map then you could use Type{} to initialize an empty value of that type.
package main import ( "fmt" "net/url" ) func main() { vals := make(url.Values) // OR // vals := url.Values{} vals.Add("foo", "bar") fmt.Println(vals) }
$ go run urlvals.go map[foo:[bar]] foo=bar
It is also recommended by the golang team to use the make function while initializing a map. So, either use make for maps, slices, and channels, or initialize the empty value variable with Type{}. Both of them work similarly, but the latter is more generally applicable to structs as well.
Conclusion
Understanding the difference between declaring and initializing maps in Golang is essential for any developer, not just in golang, but in general. As we've explored, simply declaring a map variable without initializing it can lead to runtime errors, such as panics when attempting to access or modify a nil map. Initializing a map ensures that it is properly allocated in memory and ready for use, thereby avoiding these pitfalls.
By following best practices—such as using the make function or initializing with Type{}—you can prevent common issues related to uninitialized maps. Always ensure that maps and slices are explicitly initialized before use to safeguard against unexpected nil pointer dereferences
Thank you for reading this post, If you have any questions, feedback, and suggestions, feel free to drop them in the comments.
Happy Coding :)
以上是在 Golang 中安全使用 Map:宣告和初始化的差異的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
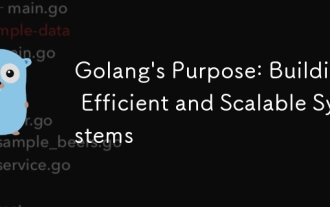
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
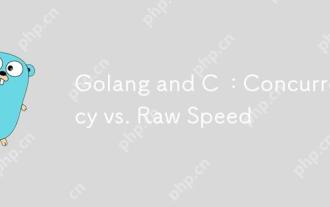
Golang在並發性上優於C ,而C 在原始速度上優於Golang。 1)Golang通過goroutine和channel實現高效並發,適合處理大量並發任務。 2)C 通過編譯器優化和標準庫,提供接近硬件的高性能,適合需要極致優化的應用。
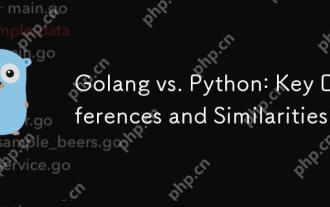
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。Golang以其并发模型和高效性能著称,Python则以简洁语法和丰富库生态系统著称。
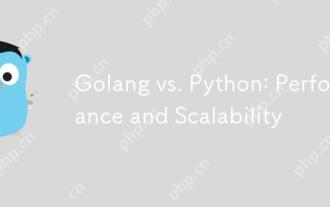
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
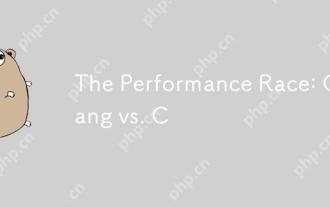
Golang和C 在性能競賽中的表現各有優勢:1)Golang適合高並發和快速開發,2)C 提供更高性能和細粒度控制。選擇應基於項目需求和團隊技術棧。
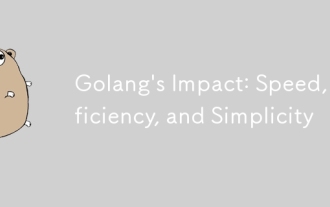
goimpactsdevelopmentpositationality throughspeed,效率和模擬性。 1)速度:gocompilesquicklyandrunseff,IdealforlargeProjects.2)效率:效率:ITScomprehenSevestAndardArdardArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增強的Depleflovelmentimency.3)簡單性。
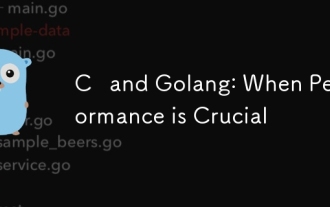
C 更適合需要直接控制硬件資源和高性能優化的場景,而Golang更適合需要快速開發和高並發處理的場景。 1.C 的優勢在於其接近硬件的特性和高度的優化能力,適合遊戲開發等高性能需求。 2.Golang的優勢在於其簡潔的語法和天然的並發支持,適合高並發服務開發。
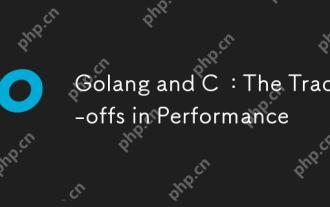
Golang和C 在性能上的差異主要體現在內存管理、編譯優化和運行時效率等方面。 1)Golang的垃圾回收機制方便但可能影響性能,2)C 的手動內存管理和編譯器優化在遞歸計算中表現更為高效。
