以非連續方式儲存元素的線性資料結構稱為 LinkedList,其中指標用於將鍊錶中的元素相互鏈接,System.Collections.Generic 命名空間由 LinkedList C# 中的類,可以以非常快速的方式從中刪除或插入元素,實現經典鍊錶,並且每個對象的分配在鍊錶中是單獨的,並且無需複製整個集合來執行某些操作在鏈接列表上。
文法:
C#中LinkedList類別的語法如下:
LinkedList<Type> linkedlist_name = new LinkedList <Type>();
登入後複製
其中Type代表鍊錶的型別。
C# 中 LinkedList 類別的工作
- 鍊錶中存在節點,每個節點由兩個部分組成,即資料欄位和指向鍊錶中下一個節點的連結。
- 鍊錶中每個節點的型別都是LinkedListNode;型別。
- 節點可以從鍊錶中刪除,也可以插入回同一個鍊錶,也可以插入到另一個鍊錶,因此堆上沒有額外的分配。
- 向鍊錶中插入元素、從鍊錶中刪除元素、取得按讚清單維護的內部屬性count屬性都是O(1)操作。
- 鍊錶類別支援枚舉器,因為它是通用鍊錶。
- 鍊錶不支援任何使鍊錶不一致的東西。
- 如果鍊錶是雙向鍊錶,那麼每個節點都有兩個指針,一個指向鍊錶中的前一個節點,另一個指向鍊錶中的下一個節點。
LinkedList 類別的建構子
C#中的LinkedList類別中有幾個建構子。他們是:
-
LinkedList(): 鍊錶類別的新實例被初始化,該實例為空。
-
LinkedList(IEnumerable):初始化鍊錶類別的新實例,該實例取自 IEnumerable 的指定實現,其容量足以累積所有複製的元素。
-
LinkedList(SerializationInfo, StreamingContext): 鍊錶類別的新實例被初始化,可以使用指定為參數的serializationInfo和StreamingContext進行序列化。
C#中LinkedList類別的方法
C#中的LinkedList類別中有幾個方法。他們是:
-
AddAfter: A value or new node is added after an already present node in the linked list using the AddAfter method.
-
AddFirst: A value or new node is added at the beginning of the linked list using the AddFirst method.
-
AddBefore: A value or new node is added before an already present node in the linked list using the AddBefore method.
-
AddLast: A value or new node is added at the end of the linked list using the AddLast method.
-
Remove(LinkedListNode): A node specified as a parameter will be removed from the linked list using Remove(LinkedListNode) method.
-
RemoveFirst(): A node at the beginning of the linked list will be removed from the linked list using RemoveFirst() method.
-
Remove(T): The first occurrence of the value specified as a parameter in the linked list will be removed from the linked list using the Remove(T) method.
-
RemoveLast(): A node at the end of the linked list will be removed from the linked list using the RemoveLast() method.
-
Clear(): All the nodes from the linked list will be removed using the Clear() method.
-
Find(T): The value specified as the parameter present in the very first node will be identified by using the Find(T) method.
-
Contains(T): We can use the Contains(T) method to find out if a value is present in the linked list or not.
-
ToString(): A string representing the current object is returned by using the ToString() method.
-
CopyTo(T[], Int32): The whole linked list is copied to an array which is one dimensional and is compatible with the linked list and the linked list begins at the index specified in the array to be copied to using CopyTo(T[], Int32) method.
-
OnDeserialization(Object): After the completion of deserialization, an event of deserialization is raised and the ISerializable interface is implemented using OnDeserialization(Object) method.
-
Equals(Object): If the object specified as the parameter is equal to the current object or not is identified using Equals(Object) method.
-
FindLast(T): The value specified as the parameter present in the last node will be identified by using FindLast(T) method.
-
MemberwiseClone(): A shallow copy of the current object is created using MemeberwiseClone() method.
-
GetEnumerator(): An enumerator is returned using GetEnumerator() method and the returned enumerator loops through the linked list.
-
GetType(): The type of the current instance is returned using GetType() method.
-
GetHashCode(): The GetHashCode() method is the hash function by default.
-
GetObjectData(SerializationInfo, StreamingContext): The data which is necessary to make the linked list serializable is returned by using GetObjectData(SerializationInfo, StreamingContext) method along with implementing the ISerializable interface.
Example of LinkedList Class in C#
C# program to demonstrate AddLast() method, Remove(LinkedListNode) method, Remove(T) method, RemoveFirst() method, RemoveLast() method and Clear() method in Linked List class:
Code:
using System;
using System.Collections.Generic;
//a class called program is defined
public class program
{
// Main Method is called
static public void Main()
{
//a new linked list is created
LinkedList<String> list = new LinkedList<String>();
//AddLast() method is used to add the elements to the newly created linked list
list.AddLast("Karnataka");
list.AddLast("Mumbai");
list.AddLast("Pune");
list.AddLast("Hyderabad");
list.AddLast("Chennai");
list.AddLast("Delhi");
Console.WriteLine("The states in India are:");
//Using foreach loop to display the elements of the newly created linked list
foreach(string places in list)
{
Console.WriteLine(places);
}
Console.WriteLine("The places after using Remove(LinkedListNode) method are:");
//using Remove(LinkedListNode) method to remove a node from the linked list
list.Remove(list.First);
foreach(string place in list)
{
Console.WriteLine(place);
}
Console.WriteLine("The places after using Remove(T) method are:");
//using Remove(T) method to remove a node from the linked list
list.Remove("Chennai");
foreach(string plac in list)
{
Console.WriteLine(plac);
}
Console.WriteLine("The places after using RemoveFirst() method are:");
//using RemoveFirst() method to remove the first node from the linked list
list.RemoveFirst();
foreach(string pla in list)
{
Console.WriteLine(pla);
}
Console.WriteLine("The places after using RemoveLast() method are:");
//using RemoveLast() method to remove the last node from the linked list
list.RemoveLast();
foreach(string pl in list)
{
Console.WriteLine(pl);
}
//using Clear() method to remove all the nodes from the linked list
list.Clear();
Console.WriteLine("The count of places after using Clear() method is: {0}",
list.Count);
}
}
登入後複製
The output of the above program is as shown in the snapshot below:
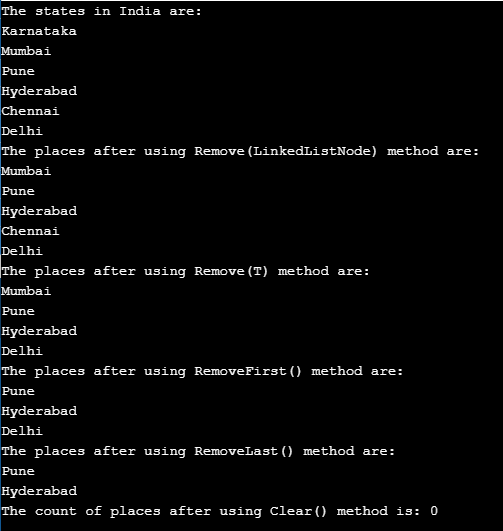
In the above program, a class called program is defined. Then the main method is called. Then a new linked list is created. Then AddLast() method is used to add the elements to the newly created linked list. Then foreach loop is used to display the elements of the newly created linked list. Then Remove(LinkedListNode) method is used to remove a node from the linked list. Then Remove(T) method is used to remove a node from the linked list. Then RemoveFirst() method is used to remove the first node from the linked list. Then RemoveLast() method is used to remove the last node from the linked list. Then Clear() method is used to remove all the nodes from the linked list. The output of the program is shown in the snapshot above.
以上是C# 鍊錶的詳細內容。更多資訊請關注PHP中文網其他相關文章!