用 Go 建構一個簡單的負載平衡器
負載平衡器在現代軟體開發中至關重要。如果您曾經想知道如何在多個伺服器之間分配請求,或者為什麼某些網站即使在流量大的情況下也感覺更快,答案通常在於高效的負載平衡。
在這篇文章中,我們將使用 Go 中的 循環演算法 建立一個簡單的應用程式負載平衡器。這篇文章的目的是逐步了解負載平衡器的底層工作原理。
什麼是負載平衡器?
負載平衡器是一個在多個伺服器之間分配傳入網路流量的系統。它確保沒有任何一台伺服器承受過多的負載,防止瓶頸並改善整體使用者體驗。負載平衡方法還確保如果一台伺服器發生故障,則流量可以自動重新路由到另一台可用的伺服器,從而減少故障的影響並提高可用性。
我們為什麼要使用負載平衡器?
- 高可用性:透過分配流量,負載平衡器確保即使一台伺服器發生故障,流量也可以路由到其他健康的伺服器,從而使應用程式更具彈性。
- 可擴展性:負載平衡器可讓您透過隨著流量的增加而添加更多伺服器來水平擴展系統。
- 效率:它透過確保所有伺服器平等地分擔工作負載來最大化資源利用率。
負載平衡演算法
有不同的演算法和策略來分配流量:
- 循環法:最簡單的方法之一。它在可用伺服器之間按順序分配請求。一旦到達最後一個伺服器,它就會從頭開始。
- 加權輪循:與輪循演算法類似,只是每個伺服器都被分配了一些固定的數位權重。這個給定的權重用於確定路由流量的伺服器。
- 最少連接:將流量路由到活動連接最少的伺服器。
- IP 雜湊:根據客戶端的 IP 位址選擇伺服器。
在這篇文章中,我們將專注於實作循環負載平衡器。
什麼是循環演算法?
循環演算法以循環方式將每個傳入請求傳送到下一個可用伺服器。如果伺服器 A 處理第一個請求,伺服器 B 將處理第二個請求,伺服器 C 將處理第三個請求。一旦所有伺服器都收到請求,它就會從伺服器 A 重新開始。
現在,讓我們進入程式碼並建立我們的負載平衡器!
第 1 步:定義負載平衡器和伺服器
type LoadBalancer struct { Current int Mutex sync.Mutex }
我們先定義一個簡單的 LoadBalancer 結構,其中包含一個 Current 欄位來追蹤哪個伺服器應該處理下一個請求。互斥體確保我們的程式碼可以安全地同時使用。
我們負載平衡的每個伺服器都是由 Server 結構體定義的:
type Server struct { URL *url.URL IsHealthy bool Mutex sync.Mutex }
這裡,每個伺服器都有一個 URL 和一個 IsHealthy 標誌,該標誌指示伺服器是否可以處理請求。
第 2 步:循環演算法
我們的負載平衡器的核心是循環演算法。其工作原理如下:
func (lb *LoadBalancer) getNextServer(servers []*Server) *Server { lb.Mutex.Lock() defer lb.Mutex.Unlock() for i := 0; i < len(servers); i++ { idx := lb.Current % len(servers) nextServer := servers[idx] lb.Current++ nextServer.Mutex.Lock() isHealthy := nextServer.IsHealthy nextServer.Mutex.Unlock() if isHealthy { return nextServer } } return nil }
- 此方法以循環方式循環遍歷伺服器清單。如果所選伺服器運作狀況良好,則會傳回該伺服器來處理傳入請求。
- 我們使用 Mutex 來確保一次只有一個 Goroutine 可以存取和修改負載平衡器的 Current 欄位。這確保了循環演算法在同時處理多個請求時正確運行。
- 每個伺服器也有自己的互斥鎖。當我們檢查 IsHealthy 欄位時,我們會鎖定伺服器的 Mutex 以防止多個 goroutine 並發存取。
- 如果沒有互斥鎖,另一個 goroutine 可能會更改值,導致讀取不正確或不一致的資料。
- 更新 Current 欄位或讀取 IsHealthy 欄位值後,我們會立即解鎖互斥體,以保持臨界區盡可能小。透過這種方式,我們使用互斥來避免任何競爭條件。
步驟 3:配置負載平衡器
我們的設定儲存在 config.json 檔案中,其中包含伺服器 URL 和執行狀況檢查間隔(更多資訊請參閱下一節)。
type Config struct { Port string `json:"port"` HealthCheckInterval string `json:"healthCheckInterval"` Servers []string `json:"servers"` }
設定檔可能如下所示:
{ "port": ":8080", "healthCheckInterval": "2s", "servers": [ "http://localhost:5001", "http://localhost:5002", "http://localhost:5003", "http://localhost:5004", "http://localhost:5005" ] }
Step 4: Health Checks
We want to make sure that the servers are healthy before routing any incoming traffic to them. This is done by sending periodic health checks to each server:
func healthCheck(s *Server, healthCheckInterval time.Duration) { for range time.Tick(healthCheckInterval) { res, err := http.Head(s.URL.String()) s.Mutex.Lock() if err != nil || res.StatusCode != http.StatusOK { fmt.Printf("%s is down\n", s.URL) s.IsHealthy = false } else { s.IsHealthy = true } s.Mutex.Unlock() } }
Every few seconds (as specified in the config), the load balancer sends a HEAD request to each server to check if it is healthy. If a server is down, the IsHealthy flag is set to false, preventing future traffic from being routed to it.
Step 5: Reverse Proxy
When the load balancer receives a request, it forwards the request to the next available server using a reverse proxy. In Golang, the httputil package provides a built-in way to handle reverse proxying, and we will use it in our code through the ReverseProxy function:
func (s *Server) ReverseProxy() *httputil.ReverseProxy { return httputil.NewSingleHostReverseProxy(s.URL) }
What is a Reverse Proxy?
A reverse proxy is a server that sits between a client and one or more backend severs. It receives the client's request, forwards it to one of the backend servers, and then returns the server's response to the client. The client interacts with the proxy, unaware of which specific backend server is handling the request.
In our case, the load balancer acts as a reverse proxy, sitting in front of multiple servers and distributing incoming HTTP requests across them.
Step 6: Handling Requests
When a client makes a request to the load balancer, it selects the next available healthy server using the round robin algorithm implementation in getNextServer function and proxies the client request to that server. If no healthy server is available then we send service unavailable error to the client.
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { server := lb.getNextServer(servers) if server == nil { http.Error(w, "No healthy server available", http.StatusServiceUnavailable) return } w.Header().Add("X-Forwarded-Server", server.URL.String()) server.ReverseProxy().ServeHTTP(w, r) })
The ReverseProxy method proxies the request to the actual server, and we also add a custom header X-Forwarded-Server for debugging purposes (though in production, we should avoid exposing internal server details like this).
Step 7: Starting the Load Balancer
Finally, we start the load balancer on the specified port:
log.Println("Starting load balancer on port", config.Port) err = http.ListenAndServe(config.Port, nil) if err != nil { log.Fatalf("Error starting load balancer: %s\n", err.Error()) }
Working Demo
TL;DR
In this post, we built a basic load balancer from scratch in Golang using a round robin algorithm. This is a simple yet effective way to distribute traffic across multiple servers and ensure that your system can handle higher loads efficiently.
There's a lot more to explore, such as adding sophisticated health checks, implementing different load balancing algorithms, or improving fault tolerance. But this basic example can be a solid foundation to build upon.
You can find the source code in this GitHub repo.
以上是用 Go 建構一個簡單的負載平衡器的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
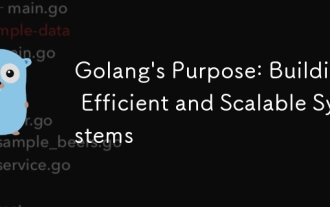
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
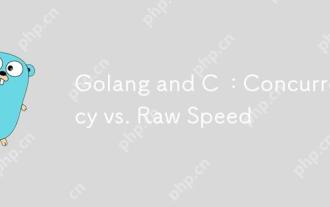
Golang在並發性上優於C ,而C 在原始速度上優於Golang。 1)Golang通過goroutine和channel實現高效並發,適合處理大量並發任務。 2)C 通過編譯器優化和標準庫,提供接近硬件的高性能,適合需要極致優化的應用。
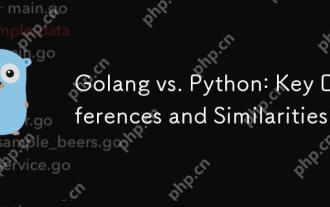
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。Golang以其并发模型和高效性能著称,Python则以简洁语法和丰富库生态系统著称。
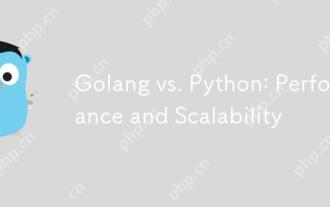
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
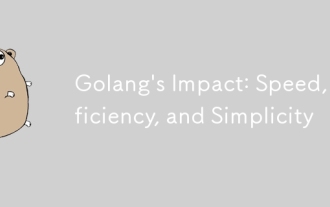
goimpactsdevelopmentpositationality throughspeed,效率和模擬性。 1)速度:gocompilesquicklyandrunseff,IdealforlargeProjects.2)效率:效率:ITScomprehenSevestAndardArdardArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增強的Depleflovelmentimency.3)簡單性。
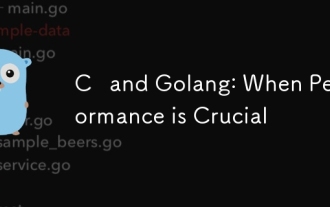
C 更適合需要直接控制硬件資源和高性能優化的場景,而Golang更適合需要快速開發和高並發處理的場景。 1.C 的優勢在於其接近硬件的特性和高度的優化能力,適合遊戲開發等高性能需求。 2.Golang的優勢在於其簡潔的語法和天然的並發支持,適合高並發服務開發。
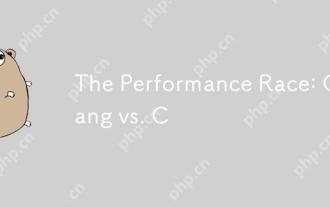
Golang和C 在性能競賽中的表現各有優勢:1)Golang適合高並發和快速開發,2)C 提供更高性能和細粒度控制。選擇應基於項目需求和團隊技術棧。
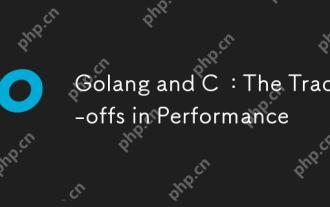
Golang和C 在性能上的差異主要體現在內存管理、編譯優化和運行時效率等方面。 1)Golang的垃圾回收機制方便但可能影響性能,2)C 的手動內存管理和編譯器優化在遞歸計算中表現更為高效。
