掌握 JavaScript 的數學物件:內建數學函數和屬性的綜合指南
JavaScript 數學物件:概述
JavaScript Math 物件是內建對象,提供數學函數和常數的集合。它不是建構函數,因此您無法建立它的實例;相反,它是透過其靜態方法和屬性直接使用的。
1.常數
Math 物件包含幾個對數學計算有用的常數:
- Math.E:自然對數的底數,約等於 2.718。
- Math.LN2:2的自然對數,約等於0.693。
- Math.LN10:10 的自然對數,約等於 2.303。
- Math.LOG2E:E 的以 2 為底的對數,約等於 1.442。
- Math.LOG10E:E 以 10 為底的對數,約等於 0.434。
- Math.PI:圓的周長與其直徑的比值,約等於 3.14159。
- Math.SQRT1_2:1/2 的平方根,約等於 0.707。
- Math.SQRT2:2 的平方根,約等於 1.414。
2.方法
Math 物件提供了多種執行數學運算的方法:
- Math.abs(x):傳回x的絕對值。
Math.abs(-5); // 5
- Math.ceil(x):將 x 向上捨入到最接近的整數。
Math.ceil(4.2); // 5
- Math.floor(x):將 x 向下捨去到最接近的整數。
Math.floor(4.7); // 4
- Math.round(x):將 x 四捨五入到最接近的整數。
Math.round(4.5); // 5
- Math.max(...values):傳回零個或多個數字中的最大值。
Math.max(1, 5, 3); // 5
- Math.min(...values):傳回零個或多個數字中的最小值。
Math.min(1, 5, 3); // 1
- Math.random():傳回 0(含)和 1(不含)之間的偽隨機數。
Math.random(); // e.g., 0.237
- Math.pow(base, exponent):傳回底數的指數次方。
Math.pow(2, 3); // 8
- Math.sqrt(x):傳回 x 的平方根。
Math.sqrt(9); // 3
- Math.trunc(x):傳回 x 的整數部分,刪除任何小數位。
Math.trunc(4.9); // 4
3.用法範例
以下是一些如何使用 Math 物件的實際範例:
- 產生隨機整數
function getRandomInt(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; } console.log(getRandomInt(1, 10)); // e.g., 7
- 計算斜邊
function calculateHypotenuse(a, b) { return Math.sqrt(Math.pow(a, 2) + Math.pow(b, 2)); } console.log(calculateHypotenuse(3, 4)); // 5
4.限制與注意事項
- 精確度問題:浮點運算可能會導致精確度問題。例如,由於舍入誤差,Math.sqrt(2) * Math.sqrt(2) 可能不完全等於 2。
- 不是建構子:Math 物件沒有建構子函數。所有屬性和方法都是靜態的。
數學物件方法與屬性
1. Math.abs(x)
傳回 x 的絕對值。
console.log(Math.abs(-10)); // 10 console.log(Math.abs(5.5)); // 5.5
2. Math.acos(x)
傳回 x 的反餘弦(反餘弦),以弧度表示。
console.log(Math.acos(1)); // 0 console.log(Math.acos(0)); // 1.5707963267948966 (π/2)
3. Math.acosh(x)
傳回 x 的雙曲反餘弦。
console.log(Math.acosh(1)); // 0 console.log(Math.acosh(2)); // 1.3169578969248166
4. Math.asin(x)
傳回 x 的反正弦(反正弦),以弧度表示。
console.log(Math.asin(0)); // 0 console.log(Math.asin(1)); // 1.5707963267948966 (π/2)
5. Math.asinh(x)
傳回 x 的雙曲反正弦。
console.log(Math.asinh(0)); // 0 console.log(Math.asinh(1)); // 0.881373587019543
6. Math.atan(x)
傳回 x 的反正切(反正切),以弧度表示。
console.log(Math.atan(1)); // 0.7853981633974483 (π/4) console.log(Math.atan(0)); // 0
7. Math.atan2(y, x)
傳回其參數商的反正切值,以弧度表示。
console.log(Math.atan2(1, 1)); // 0.7853981633974483 (π/4) console.log(Math.atan2(-1, -1)); // -2.356194490192345 (-3π/4)
8. Math.atanh(x)
傳回 x 的雙曲反正切值。
console.log(Math.atanh(0)); // 0 console.log(Math.atanh(0.5)); // 0.5493061443340549
9. Math.cbrt(x)
回傳 x 的立方根。
console.log(Math.cbrt(27)); // 3 console.log(Math.cbrt(-8)); // -2
10。 Math.ceil(x)
將 x 向上捨入到最接近的整數。
console.log(Math.ceil(4.2)); // 5 console.log(Math.ceil(-4.7)); // -4
11。 Math.clz32(x)
傳回 x 的 32 位元二進位表示形式中前導零的數量。
console.log(Math.clz32(1)); // 31 console.log(Math.clz32(0x80000000)); // 0
12。數學.cos(x)
傳回 x 的餘弦(其中 x 的單位是弧度)。
console.log(Math.cos(0)); // 1 console.log(Math.cos(Math.PI)); // -1
13. Math.cosh(x)
Returns the hyperbolic cosine of x.
console.log(Math.cosh(0)); // 1 console.log(Math.cosh(1)); // 1.5430806348152437
14. Math.E
Returns Euler's number, approximately 2.718.
console.log(Math.E); // 2.718281828459045
15. Math.exp(x)
Returns the value of e raised to the power of x.
console.log(Math.exp(1)); // 2.718281828459045 console.log(Math.exp(0)); // 1
16. Math.expm1(x)
Returns the value of e raised to the power of x, minus 1.
console.log(Math.expm1(1)); // 1.718281828459045 console.log(Math.expm1(0)); // 0
17. Math.floor(x)
Rounds x downwards to the nearest integer.
console.log(Math.floor(4.7)); // 4 console.log(Math.floor(-4.2)); // -5
18. Math.fround(x)
Returns the nearest (32-bit single precision) float representation of x.
console.log(Math.fround(1.337)); // 1.336914 console.log(Math.fround(1.5)); // 1.5
19. Math.LN2
Returns the natural logarithm of 2, approximately 0.693.
console.log(Math.LN2); // 0.6931471805599453
20. Math.LN10
Returns the natural logarithm of 10, approximately 2.302.
console.log(Math.LN10); // 2.302585092994046
21. Math.log(x)
Returns the natural logarithm (base e) of x.
console.log(Math.log(Math.E)); // 1 console.log(Math.log(10)); // 2.302585092994046
22. Math.log10(x)
Returns the base-10 logarithm of x.
console.log(Math.log10(10)); // 1 console.log(Math.log10(100)); // 2
23. Math.LOG10E
Returns the base-10 logarithm of e, approximately 0.434.
console.log(Math.LOG10E); // 0.4342944819032518
24. Math.log1p(x)
Returns the natural logarithm of 1 + x.
console.log(Math.log1p(1)); // 0.6931471805599453 console.log(Math.log1p(0)); // 0
25. Math.log2(x)
Returns the base-2 logarithm of x.
console.log(Math.log2(2)); // 1 console.log(Math.log2(8)); // 3
26. Math.LOG2E
Returns the base-2 logarithm of e, approximately 1.442.
console.log(Math.LOG2E); // 1.4426950408889634
27. Math.max(...values)
Returns the largest of zero or more numbers.
console.log(Math.max(1, 5, 3)); // 5 console.log(Math.max(-1, -5, -3)); // -1
28. Math.min(...values)
Returns the smallest of zero or more numbers.
console.log(Math.min(1, 5, 3)); // 1 console.log(Math.min(-1, -5, -3)); // -5
29. Math.PI
Returns the value of π, approximately 3.14159.
console.log(Math.PI); // 3.141592653589793
30. Math.pow(base, exponent)
Returns the value of base raised to the power of exponent.
console.log(Math.pow(2, 3)); // 8 console.log(Math.pow(5, 0)); // 1
31. Math.random()
Returns a pseudo-random number between 0 (inclusive) and 1 (exclusive).
console.log(Math.random()); // e.g., 0.237
32. Math.round(x)
Rounds x to the nearest integer.
console.log(Math.round(4.5)); // 5 console.log(Math.round(4.4)); // 4
33. Math.sign(x)
Returns the sign of a number, indicating whether the number is positive, negative, or zero.
console.log(Math.sign(-5)); // -1 console.log(Math.sign(0)); // 0 console.log(Math.sign(5)); // 1
34. Math.sin(x)
Returns the sine of x (where x is in radians).
console.log(Math.sin(0)); // 0 console.log(Math.sin(Math.PI / 2)); // 1
35. Math.sinh(x)
Returns the hyperbolic sine of x.
console.log(Math.sinh(0)); // 0 console.log(Math.sinh(1)); // 1.1752011936438014
36. Math.sqrt(x)
Returns the square root of x.
console.log(Math.sqrt(9)); // 3 console.log(Math.sqrt(16)); // 4
37. Math.SQRT1_2
Returns the square root of 1/2, approximately 0.707.
console.log(Math.SQRT1_2); // 0.7071067811865476
38. Math.SQRT2
Returns the square root of 2, approximately 1.414.
console.log(Math.SQRT2); // 1.4142135623730951
39. Math.tan(x)
Returns the tangent of x (where x is in radians).
console.log(Math.tan(0)); // 0 console.log(Math.tan(Math.PI / 4)); // 1
40. Math.tanh(x)
Returns the hyperbolic tangent of x.
console.log(Math.tanh(0)); // 0 console.log(Math.tanh(1)); // 0.7615941559557649
41. Math.trunc(x)
Returns the integer part of a number by removing any fractional digits.
console.log(Math.trunc(4.9)); // 4 console.log(Math.trunc(-4.9)); // -4
以上是掌握 JavaScript 的數學物件:內建數學函數和屬性的綜合指南的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
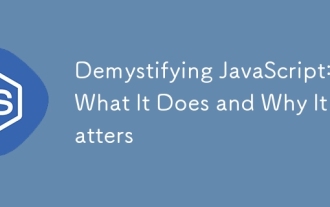
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
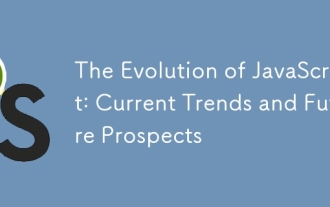
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
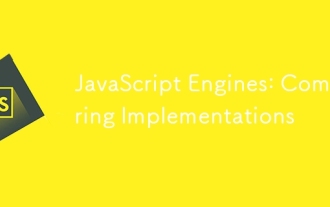
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
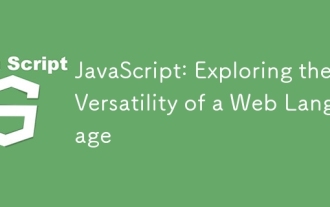
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
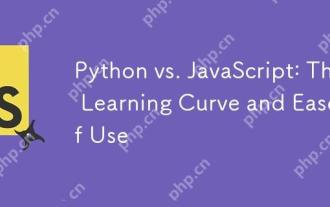
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
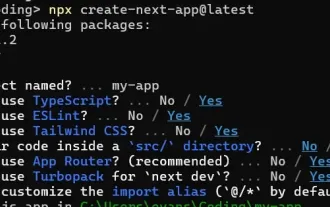
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
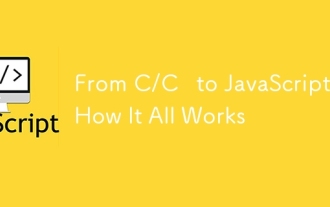
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
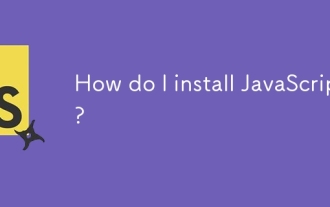
JavaScript不需要安裝,因為它已內置於現代瀏覽器中。你只需文本編輯器和瀏覽器即可開始使用。 1)在瀏覽器環境中,通過標籤嵌入HTML文件中運行。 2)在Node.js環境中,下載並安裝Node.js後,通過命令行運行JavaScript文件。
