使用 Completable Future 處理 Java 中的多執行緒
1. 理解完整的未來
CompletableFuture 是java.util.concurrent 套件的一部分,提供了一種以更具可讀性和可維護性的方式編寫非同步、非阻塞程式碼的方法。它代表非同步計算的未來結果。
1.1 建立一個簡單的CompletableFuture
從 CompletableFuture 開始,您可以建立一個簡單的非同步任務。這是一個例子:
import java.util.concurrent.CompletableFuture; public class CompletableFutureExample { public static void main(String[] args) { CompletableFuture<Void> future = CompletableFuture.runAsync(() -> { System.out.println("Running asynchronously..."); // Simulate a long-running task try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } }); future.join(); // Wait for the task to complete System.out.println("Task completed."); } }
- CompletableFuture.runAsync() 非同步執行任務。
- future.join() 阻塞主線程,直到任務完成。
示範結果:
Running asynchronously... Task completed.
1.2 將 CompletableFuture 與結果結合使用
您也可以使用CompletableFuture傳回非同步任務的結果:
import java.util.concurrent.CompletableFuture; public class CompletableFutureWithResult { public static void main(String[] args) { CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> { // Simulate a computation return 5 * 5; }); future.thenAccept(result -> { System.out.println("The result is: " + result); }).join(); } }
- CompletableFuture.supplyAsync() 用於傳回結果的任務。
- thenAccept() 計算完成後處理結果。
示範結果:
The result is: 25
2. 組合多個CompletableFuture
處理多個非同步任務是一個常見的用例。 CompletableFuture 提供了多種組合 future 的方法。
2.1 將 Future 與 thenCombine 結合起來
您可以組合多個 CompletableFutures 的結果:
import java.util.concurrent.CompletableFuture; public class CombiningFutures { public static void main(String[] args) { CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> 5); CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> 10); CompletableFuture<Integer> combinedFuture = future1.thenCombine(future2, (result1, result2) -> result1 + result2); combinedFuture.thenAccept(result -> { System.out.println("Combined result: " + result); }).join(); } }
- thenCombine () 組合兩個 future 的結果。
- 使用 thenAccept () 處理組合結果。
示範結果:
Combined result: 15
2.2 使用 allOf 處理多個 Future
當需要等待多個 future 完成時,使用 CompletableFuture.allOf():
import java.util.concurrent.CompletableFuture; public class AllOfExample { public static void main(String[] args) { CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> { // Simulate task try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } }); CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> { // Simulate another task try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } }); CompletableFuture<Void> allOfFuture = CompletableFuture.allOf(future1, future2); allOfFuture.join(); System.out.println("All tasks completed."); } }
- CompletableFuture.allOf() 等待所有給定的 future 完成。
- join() 確保主執行緒等待,直到所有任務完成。
示範結果:
All tasks completed.
3. CompletableFuture 的錯誤處理
處理錯誤在非同步程式設計中至關重要。 CompletableFuture 提供了管理異常的方法。
3.1 使用異常處理異常
使用異常()處理非同步任務中的異常:
import java.util.concurrent.CompletableFuture; public class ExceptionHandlingExample { public static void main(String[] args) { CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> { throw new RuntimeException("Something went wrong!"); }).exceptionally(ex -> { System.out.println("Exception occurred: " + ex.getMessage()); return null; }); future.join(); } }
- 異常 () 捕捉並處理異常。
- 它允許您提供後備結果或處理錯誤。
示範結果:
Exception occurred: Something went wrong!
4.CompletableFuture的優缺點
4.1 優點
- 非同步執行:高效處理並發運行的任務,而不阻塞主執行緒。
- 提高了可讀性:與傳統的回呼方法相比,提供了一種更具可讀性和可維護性的方式來處理非同步程式碼。
- 豐富的 API :提供多種方法來組合、處理和組合多個 future。
4.2 缺點
- 複雜性:CompletableFuture 雖然功能強大,但會在管理和偵錯非同步程式碼時引入複雜性。
- 異常處理:處理異常有時可能很棘手,尤其是在具有多個階段的複雜場景中。
5. 結論
在本指南中,我們探討如何使用 CompletableFuture 處理 Java 中的並發請求。從建立簡單的非同步任務到組合多個 future 和處理錯誤,CompletableFuture 提供了一種健壯且靈活的非同步程式設計方法。
如果您有任何疑問或需要進一步協助,請隨時在下面發表評論。我很樂意提供協助!
閱讀更多文章:使用 Completable Future 處理 Java 中的多執行緒
以上是使用 Completable Future 處理 Java 中的多執行緒的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
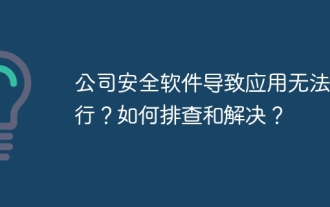
公司安全軟件導致部分應用無法正常運行的排查與解決方法許多公司為了保障內部網絡安全,會部署安全軟件。 ...
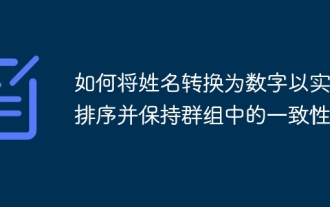
將姓名轉換為數字以實現排序的解決方案在許多應用場景中,用戶可能需要在群組中進行排序,尤其是在一個用...
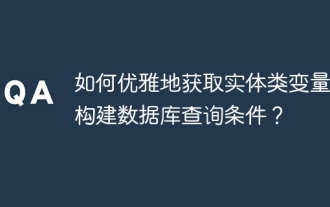
在使用MyBatis-Plus或其他ORM框架進行數據庫操作時,經常需要根據實體類的屬性名構造查詢條件。如果每次都手動...
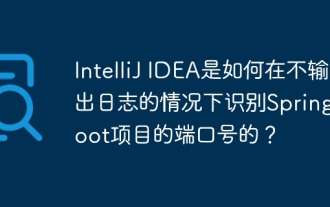
在使用IntelliJIDEAUltimate版本啟動Spring...
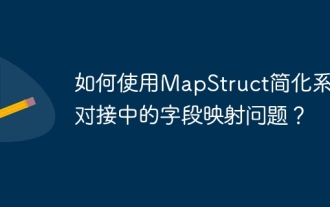
系統對接中的字段映射處理在進行系統對接時,常常會遇到一個棘手的問題:如何將A系統的接口字段有效地映�...
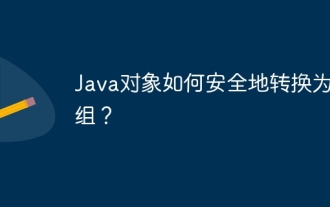
Java對象與數組的轉換:深入探討強制類型轉換的風險與正確方法很多Java初學者會遇到將一個對象轉換成數組的�...
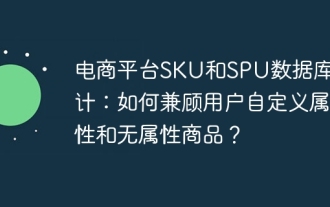
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
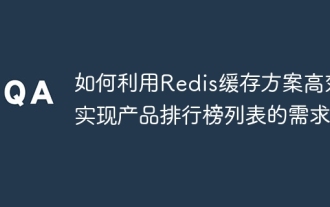
Redis緩存方案如何實現產品排行榜列表的需求?在開發過程中,我們常常需要處理排行榜的需求,例如展示一個�...
