使用 React 建立主題切換的 Todo 應用程式
介紹
在本教程中,我們將使用 React 建立一個 待辦事項清單 Web 應用程式。該項目有助於理解狀態管理、事件處理以及在 React 中使用清單。對於想要增強 React 開發技能的初學者來說,它是完美的選擇。
項目概況
待辦事項清單應用程式允許使用者新增、標記為已完成和刪除任務。它提供了一個乾淨的介面來管理日常任務。該專案展示瞭如何使用 React 來管理簡單而動態的應用程式的狀態。
特徵
- 新增任務:使用者可以將任務新增到清單中。
- 標記為已完成:使用者可以將任務標記為已完成。
- 刪除任務:使用者可以從清單中刪除任務。
- 本地儲存:使用 localStorage 在頁面重新載入時保留任務。
使用的技術
- React:用於建立使用者介面和管理元件狀態。
- CSS:用於設計應用程式的樣式。
- JavaScript:核心邏輯與功能。
專案結構
專案結構遵循典型的 React 專案佈局:
├── public ├── src │ ├── components │ │ ├── TodoList.jsx │ │ ├── TodoItem.jsx │ ├── App.jsx │ ├── App.css │ ├── index.js │ └── index.css ├── package.json └── README.md
關鍵零件
- TodoList.jsx:處理待辦事項清單的顯示與管理。
- TodoItem.jsx:管理單一待辦事項,包括將它們標記為已完成或刪除它們。
程式碼說明
待辦事項清單組件
此元件處理整個待辦事項清單的狀態,包括新增任務和渲染清單。
import { useState, useEffect } from "react"; import TodoItem from "./TodoItem"; const TodoList = () => { const [task, setTask] = useState(""); const [tasks, setTasks] = useState([]); useEffect(() => { const savedTasks = JSON.parse(localStorage.getItem("tasks")) || []; setTasks(savedTasks); }, []); useEffect(() => { localStorage.setItem("tasks", JSON.stringify(tasks)); }, [tasks]); const addTask = () => { if (task.trim()) { setTasks([...tasks, { text: task, completed: false }]); setTask(""); } }; const toggleCompletion = (index) => { const newTasks = tasks.map((t, i) => i === index ? { ...t, completed: !t.completed } : t ); setTasks(newTasks); }; const deleteTask = (index) => { const newTasks = tasks.filter((_, i) => i !== index); setTasks(newTasks); }; return ( <div classname="todo-list"> <h1 id="Todo-List">Todo List</h1> <input type="text" value="{task}" onchange="{(e)"> setTask(e.target.value)} placeholder="Add a new task" /> <button onclick="{addTask}">Add Task</button> <ul> {tasks.map((t, index) => ( <todoitem key="{index}" task="{t}" index="{index}" togglecompletion="{toggleCompletion}" deletetask="{deleteTask}"></todoitem> ))} </ul> </div> ); }; export default TodoList;
TodoItem 元件
TodoItem 元件管理每個任務的顯示,以及將其標記為已完成或刪除的選項。
const TodoItem = ({ task, index, toggleCompletion, deleteTask }) => { return (
在此元件中,我們從父 TodoList 接收 props 並處理諸如切換任務完成或刪除任務之類的操作。
應用程式元件
App.jsx 作為應用程式的根,渲染 TodoList 元件。
import { useState } from "react"; import "./App.css"; import TodoList from './components/TodoList'; import sun from "./assets/images/icon-sun.svg"; import moon from "./assets/images/icon-moon.svg"; const App = () => { const [isLightTheme, setIsLightTheme] = useState(false); const toggleTheme = () => { setIsLightTheme(!isLightTheme); }; return ( <div classname="{isLightTheme" :> <div classname="app"> <div classname="header"> <div classname="title"> <h1 id="TODO">TODO</h1> </div> <div classname="mode" onclick="{toggleTheme}"> <img src="%7BisLightTheme" moon : sun alt="使用 React 建立主題切換的 Todo 應用程式"> </div> </div> <todo></todo> <div classname="footer"> <p>Made with ❤️ by Abhishek Gurjar</p> </div> </div> </div> ); }; export default App;
CSS 樣式
CSS 確保待辦事項清單應用程式用戶友好且響應迅速。
* { box-sizing: border-box; } body { margin: 0; padding: 0; font-family: Josefin Sans, sans-serif; } .app { width: 100%; height: 100vh; background-color: #161722; color: white; background-image: url(./assets//images/bg-desktop-dark.jpg); background-repeat: no-repeat; background-size: contain; background-position-x: center; background-position-y: top; display: flex; align-items: center; justify-content: flex-start; flex-direction: column; } .header { width: 350px; margin-top: 20px; display: flex; align-items: center; justify-content: space-between; } .title h1 { font-size: 30px; letter-spacing: 7px; } .mode { display: flex; align-items: center; justify-content: center; } .mode img { width: 22px; } .todo { width: 350px; flex-direction: column; display: flex; align-items: center; justify-content: flex-start; } .input-box { border-bottom: 1px solid white; display: flex; align-items: center; justify-content: center; background-color: #25273c; width: 100%; gap: 10px; padding: 8px; border-radius: 10px; } .check-circle { width: 12px; height: 12px; border-radius: 50%; border: 1px solid white; display: flex; align-items: center; justify-content: center; background-image: linear-gradient(to right,hsl(230, 50%, 20%) , hsl(280, 46%, 28%)); } .input-task { width: 90%; border: none; color: white; background-color: #25273c; } .input-task:focus { outline: none; } .todo-list { margin-top: 20px; width: 350px; background-color: #25273c; } .todo-box { margin-inline: 15px; margin-block: 10px; width: 100%; display: flex; align-items: center; justify-content: flex-start; gap: 15px; } .todo-box .cross{ width: 14px; } .details { margin-bottom: 40px; border-bottom: 1px solid white; width: 350px; display: flex; align-items: center; justify-content: space-evenly; background-color: #25273c; font-size: 12px; padding: 12px; border-bottom-right-radius: 7px; border-bottom-left-radius: 7px; } .details .clickBtn{ cursor: pointer; } .details .clickBtn:hover{ color: #3074fd; } /* //light Theme */ .light-theme .app { background-color: #fff; color: #000; background-image: url(./assets//images/bg-desktop-light.jpg); } .light-theme .header { color: white; } .light-theme .input-box{ background-color: white; color: black; border-bottom: 1px solid black; } .light-theme input{ background-color: white; color: black; } .light-theme .check-circle{ border:1px solid black; } .light-theme .todo-list{ background-color: white; color: black; } .light-theme .details{ border-bottom: 1px solid black; background-color: white; color: black; } .footer{ margin: 40px; }
這些樣式確保待辦事項清單簡單乾淨,同時允許任務管理。
安裝與使用
首先,複製儲存庫並安裝相依性:
git clone https://github.com/abhishekgurjar-in/todo_list.git cd todo-list npm install npm start
應用程式將在 http://localhost:3000 開始運作。
現場演示
在此處查看待辦事項清單的現場演示。
結論
Todo List 項目是練習在 React 中使用狀態、清單和事件處理的好方法。它演示瞭如何建立一個有用的應用程序,可以使用 localStorage 跨會話保存資料。
製作人員
- 靈感:受到對簡單有效的任務管理工具的需求的啟發。
作者
Abhishek Gurjar 是一位充滿熱情的 Web 開發人員。你可以在 GitHub 上查看他的更多專案。
以上是使用 React 建立主題切換的 Todo 應用程式的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
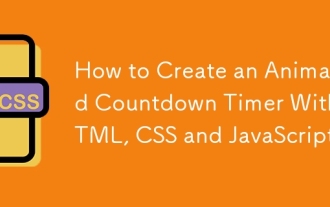
您是否曾經在項目上需要一個倒計時計時器?對於這樣的東西,可以自然訪問插件,但實際上更多
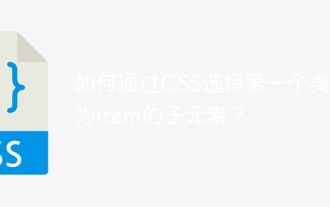
在元素個數不固定的情況下如何通過CSS選擇第一個指定類名的子元素在處理HTML結構時,常常會遇到元素個數不�...
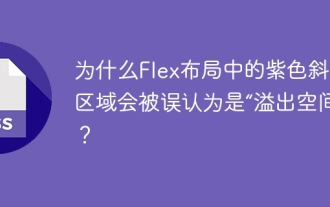
關於Flex佈局中紫色斜線區域的疑問在使用Flex佈局時,你可能會遇到一些令人困惑的現象,比如在開發者工具(d...
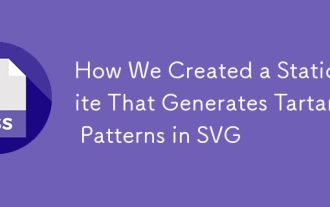
格子呢是一塊圖案布,通常與蘇格蘭有關,尤其是他們時尚的蘇格蘭語。在Tartanify.com上,我們收集了5,000多個格子呢
