課程 使用 API 和 Web 抓取實現 HR 自動化
歡迎回到我們的Python從0到英雄系列!到目前為止,我們已經學習如何操作資料並使用強大的外部函式庫來執行與工資和人力資源系統相關的任務。但是,如果您需要取得即時數據或與外部服務互動怎麼辦?這就是 API 和 網頁抓取 發揮作用的地方。
在本課中,我們將介紹:
- API是什麼以及為何它們有用。
- 如何使用 Python 的 requests 函式庫與 REST API 互動。
- 如何應用網頁抓取技術從網站擷取資料。
- 實際範例,例如取得薪資的即時稅率或從網站抓取員工福利資料。
在本課程結束時,您將能夠自動化外部資料檢索,使您的 HR 系統更加動態和資料驅動。
1.什麼是API?
API(應用程式介面)是一組允許不同軟體應用程式相互通訊的規則。簡而言之,它允許您直接從程式碼與另一個服務或資料庫互動。
例如:
- 您可以使用 API 取得即時稅率用於薪資計算。
- 您可以與人力資源軟體 API 集成,將員工資料直接提取到您的系統中。
- 或您可以使用天氣 API 來了解何時根據極端天氣條件為員工提供特殊福利。
大多數 API 使用名為 REST(表述性狀態傳輸)的標準,它允許您發送 HTTP 請求(如 GET 或 POST)來存取或更新資料。
2. 使用Requests庫與API交互
Python 的 requests 函式庫可以輕鬆使用 API。您可以透過運行來安裝它:
pip install requests
發出基本 API 請求
讓我們從一個簡單的範例開始,了解如何使用 GET 要求從 API 取得資料。
import requests # Example API to get public data url = "https://jsonplaceholder.typicode.com/users" response = requests.get(url) # Check if the request was successful (status code 200) if response.status_code == 200: data = response.json() # Parse the response as JSON print(data) else: print(f"Failed to retrieve data. Status code: {response.status_code}")
在此範例中:
- 我們使用 requests.get() 函數從 API 取得資料。
- 如果請求成功,資料會被解析為JSON,我們就可以處理它了。
HR應用範例:取得即時稅務數據
假設您想要取得即時稅率以用於薪資核算。許多國家提供了稅率的公共 API。
在此範例中,我們將模擬從稅務 API 取得資料。使用實際 API 時的邏輯是類似的。
import requests # Simulated API for tax rates api_url = "https://api.example.com/tax-rates" response = requests.get(api_url) if response.status_code == 200: tax_data = response.json() federal_tax = tax_data['federal_tax'] state_tax = tax_data['state_tax'] print(f"Federal Tax Rate: {federal_tax}%") print(f"State Tax Rate: {state_tax}%") # Use the tax rates to calculate total tax for an employee's salary salary = 5000 total_tax = salary * (federal_tax + state_tax) / 100 print(f"Total tax for a salary of ${salary}: ${total_tax:.2f}") else: print(f"Failed to retrieve tax rates. Status code: {response.status_code}")
此腳本可以修改為與實際稅率 API 搭配使用,以協助您將薪資系統保持在最新的稅率。
3. 網頁抓取來收集數據
雖然 API 是獲取資料的首選方法,但並非所有網站都提供它們。在這些情況下,網頁抓取可用於從網頁中提取資料。
Python 的 BeautifulSoup 函式庫以及要求讓網頁抓取變得簡單。您可以透過運行來安裝它:
pip install beautifulsoup4
範例:從網站上抓取員工福利數據
想像一下您想要從公司的人力資源網站上抓取有關員工福利的資料。這是一個基本範例:
import requests from bs4 import BeautifulSoup # URL of the webpage you want to scrape url = "https://example.com/employee-benefits" response = requests.get(url) # Parse the page content with BeautifulSoup soup = BeautifulSoup(response.content, 'html.parser') # Find and extract the data you need (e.g., benefits list) benefits = soup.find_all("div", class_="benefit-item") # Loop through and print out the benefits for benefit in benefits: title = benefit.find("h3").get_text() description = benefit.find("p").get_text() print(f"Benefit: {title}") print(f"Description: {description}\n")
在此範例中:
- 我們使用requests.get()請求網頁的內容。
- BeautifulSoup 物件解析 HTML 內容。
- 然後,我們使用 find_all() 來提取我們感興趣的特定元素(例如,福利標題和描述)。
此技術對於從網路收集與人力資源相關的資料(例如福利、職位發布或薪資基準)非常有用。
4. 在 HR 應用程式中結合 API 和 Web 抓取
讓我們將所有內容放在一起,創建一個結合 API 使用和 Web 抓取的迷你應用程序,用於真實的 HR 場景:計算員工的總成本。
我們會:
- Use an API to get real-time tax rates.
- Scrape a webpage for additional employee benefit costs.
Example: Total Employee Cost Calculator
import requests from bs4 import BeautifulSoup # Step 1: Get tax rates from API def get_tax_rates(): api_url = "https://api.example.com/tax-rates" response = requests.get(api_url) if response.status_code == 200: tax_data = response.json() federal_tax = tax_data['federal_tax'] state_tax = tax_data['state_tax'] return federal_tax, state_tax else: print("Error fetching tax rates.") return None, None # Step 2: Scrape employee benefit costs from a website def get_benefit_costs(): url = "https://example.com/employee-benefits" response = requests.get(url) if response.status_code == 200: soup = BeautifulSoup(response.content, 'html.parser') # Let's assume the page lists the monthly benefit cost benefit_costs = soup.find("div", class_="benefit-total").get_text() return float(benefit_costs.strip("$")) else: print("Error fetching benefit costs.") return 0.0 # Step 3: Calculate total employee cost def calculate_total_employee_cost(salary): federal_tax, state_tax = get_tax_rates() benefits_cost = get_benefit_costs() if federal_tax is not None and state_tax is not None: # Total tax deduction total_tax = salary * (federal_tax + state_tax) / 100 # Total cost = salary + benefits + tax total_cost = salary + benefits_cost + total_tax return total_cost else: return None # Example usage employee_salary = 5000 total_cost = calculate_total_employee_cost(employee_salary) if total_cost: print(f"Total cost for the employee: ${total_cost:.2f}") else: print("Could not calculate employee cost.")
How It Works:
- The get_tax_rates() function retrieves tax rates from an API.
- The get_benefit_costs() function scrapes a webpage for the employee benefits cost.
- The calculate_total_employee_cost() function calculates the total cost by combining salary, taxes, and benefits.
This is a simplified example but demonstrates how you can combine data from different sources (APIs and web scraping) to create more dynamic and useful HR applications.
Best Practices for Web Scraping
While web scraping is powerful, there are some important best practices to follow:
- Respect the website’s robots.txt: Some websites don’t allow scraping, and you should check their robots.txt file before scraping.
- Use appropriate intervals between requests: Avoid overloading the server by adding delays between requests using the time.sleep() function.
- Avoid scraping sensitive or copyrighted data: Always make sure you’re not violating any legal or ethical rules when scraping data.
Conclusion
In this lesson, we explored how to interact with external services using APIs and how to extract data from websites through web scraping. These techniques open up endless possibilities for integrating external data into your Python applications, especially in an HR context.
以上是課程 使用 API 和 Web 抓取實現 HR 自動化的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
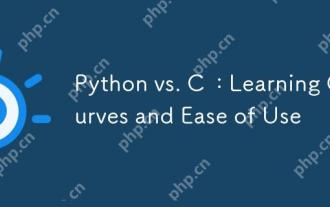
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
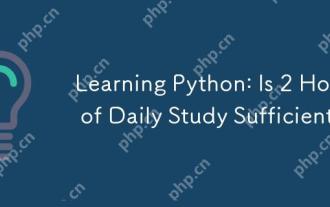
每天學習Python兩個小時是否足夠?這取決於你的目標和學習方法。 1)制定清晰的學習計劃,2)選擇合適的學習資源和方法,3)動手實踐和復習鞏固,可以在這段時間內逐步掌握Python的基本知識和高級功能。
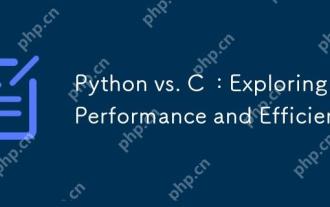
Python在開發效率上優於C ,但C 在執行性能上更高。 1.Python的簡潔語法和豐富庫提高開發效率。 2.C 的編譯型特性和硬件控制提升執行性能。選擇時需根據項目需求權衡開發速度與執行效率。
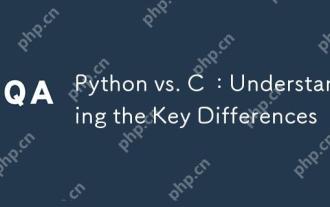
Python和C 各有優勢,選擇應基於項目需求。 1)Python適合快速開發和數據處理,因其簡潔語法和動態類型。 2)C 適用於高性能和系統編程,因其靜態類型和手動內存管理。
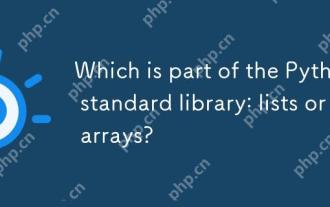
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
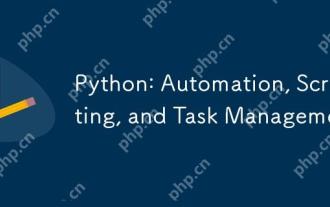
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
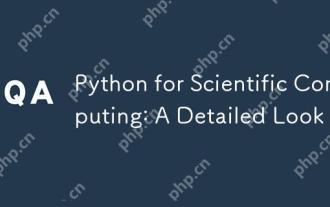
Python在科學計算中的應用包括數據分析、機器學習、數值模擬和可視化。 1.Numpy提供高效的多維數組和數學函數。 2.SciPy擴展Numpy功能,提供優化和線性代數工具。 3.Pandas用於數據處理和分析。 4.Matplotlib用於生成各種圖表和可視化結果。
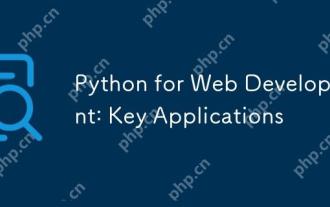
Python在Web開發中的關鍵應用包括使用Django和Flask框架、API開發、數據分析與可視化、機器學習與AI、以及性能優化。 1.Django和Flask框架:Django適合快速開發複雜應用,Flask適用於小型或高度自定義項目。 2.API開發:使用Flask或DjangoRESTFramework構建RESTfulAPI。 3.數據分析與可視化:利用Python處理數據並通過Web界面展示。 4.機器學習與AI:Python用於構建智能Web應用。 5.性能優化:通過異步編程、緩存和代碼優
