Python 多處理模組快速指南及範例
介紹
Python 中的多處理模組可讓您建立和管理進程,使您能夠充分利用機器上的多個處理器。它透過為每個進程使用單獨的記憶體空間來幫助您實現並行執行,這與線程共享相同記憶體空間的線程不同。以下是多處理模組中常用的類別和方法的列表,並附有簡短的範例。
1. 流程
Process 類別是多處理模組的核心,可讓您建立和運行新進程。
from multiprocessing import Process def print_numbers(): for i in range(5): print(i) p = Process(target=print_numbers) p.start() # Starts a new process p.join() # Waits for the process to finish
2. 開始()
啟動進程的活動。
p = Process(target=print_numbers) p.start() # Runs the target function in a separate process
3. 加入([超時])
阻塞呼叫程序,直到呼叫 join() 方法的程序終止。您可以選擇指定逾時。
p = Process(target=print_numbers) p.start() p.join(2) # Waits up to 2 seconds for the process to finish
4.is_alive()
如果進程仍在運行,則傳回 True。
p = Process(target=print_numbers) p.start() print(p.is_alive()) # True if the process is still running
5. 當前進程()
傳回表示呼叫程序的目前 Process 物件。
from multiprocessing import current_process def print_current_process(): print(current_process()) p = Process(target=print_current_process) p.start() # Prints the current process info
6.active_children()
傳回目前活動的所有 Process 物件的清單。
p1 = Process(target=print_numbers) p2 = Process(target=print_numbers) p1.start() p2.start() print(Process.active_children()) # Lists all active child processes
7. cpu_count()
傳回機器上可用的 CPU 數量。
from multiprocessing import cpu_count print(cpu_count()) # Returns the number of CPUs on the machine
8. 泳池
Pool 物件提供了一種跨多個輸入值並行執行函數的便捷方法。它管理一個工作進程池。
from multiprocessing import Pool def square(n): return n * n with Pool(4) as pool: # Pool with 4 worker processes result = pool.map(square, [1, 2, 3, 4, 5]) print(result) # [1, 4, 9, 16, 25]
9. 隊列
佇列是一種共享資料結構,允許多個進程透過在它們之間傳遞資料來進行通訊。
from multiprocessing import Process, Queue def put_data(q): q.put([1, 2, 3]) def get_data(q): data = q.get() print(data) q = Queue() p1 = Process(target=put_data, args=(q,)) p2 = Process(target=get_data, args=(q,)) p1.start() p2.start() p1.join() p2.join()
10. 鎖
鎖定確保一次只有一個行程可以存取共享資源。
from multiprocessing import Process, Lock lock = Lock() def print_numbers(): with lock: for i in range(5): print(i) p1 = Process(target=print_numbers) p2 = Process(target=print_numbers) p1.start() p2.start() p1.join() p2.join()
11. 值和數組
Value 和 Array 物件允許在進程之間共享簡單的資料類型和陣列。
from multiprocessing import Process, Value def increment(val): with val.get_lock(): val.value += 1 shared_val = Value('i', 0) processes = [Process(target=increment, args=(shared_val,)) for _ in range(10)] for p in processes: p.start() for p in processes: p.join() print(shared_val.value) # Output will be 10
12. 管道
管道提供兩個進程之間的雙向通訊通道。
from multiprocessing import Process, Pipe def send_message(conn): conn.send("Hello from child") conn.close() parent_conn, child_conn = Pipe() p = Process(target=send_message, args=(child_conn,)) p.start() print(parent_conn.recv()) # Receives data from the child process p.join()
13. 經理
管理器可讓您建立多個程序可以同時修改的共用對象,例如清單和字典。
from multiprocessing import Process, Manager def modify_list(shared_list): shared_list.append("New item") with Manager() as manager: shared_list = manager.list([1, 2, 3]) p = Process(target=modify_list, args=(shared_list,)) p.start() p.join() print(shared_list) # [1, 2, 3, "New item"]
14. 信號量
信號量允許您控制對資源的訪問,一次只允許一定數量的進程訪問它。
from multiprocessing import Process, Semaphore import time sem = Semaphore(2) # Only 2 processes can access the resource def limited_access(): with sem: print("Accessing resource") time.sleep(2) processes = [Process(target=limited_access) for _ in range(5)] for p in processes: p.start() for p in processes: p.join()
結論
Python 中的多處理模組旨在充分利用機器上的多個處理器。從使用 Process 建立和管理進程,到使用 Lock 和 Semaphore 控制共享資源,以及透過 Queue 和 Pipe 促進通信,多處理模組對於 Python 應用程式中的平行任務至關重要。
以上是Python 多處理模組快速指南及範例的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
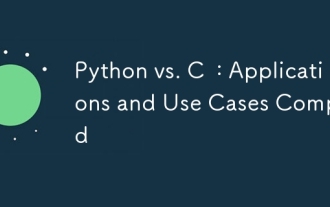
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
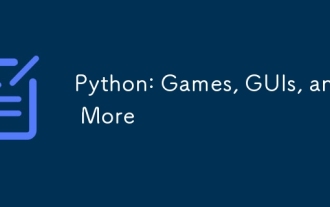
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
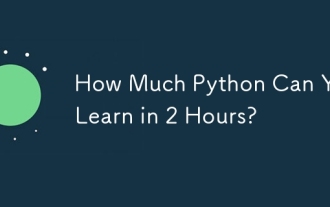
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
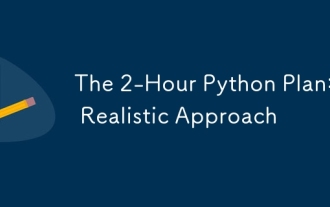
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
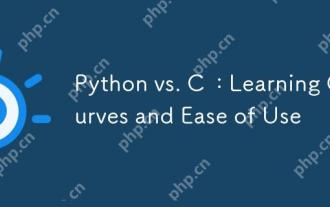
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
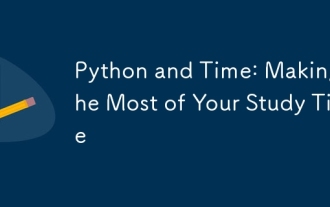
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
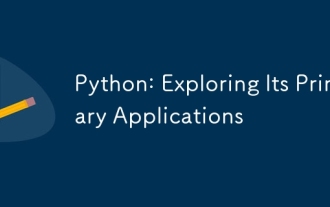
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
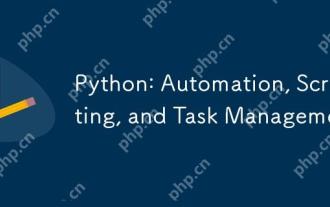
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
