Laravel 中程式碼優化和效能改進的技巧
Laravel 是一個健壯且優雅的框架,但隨著應用程式的成長,優化其效能變得至關重要。這是一份包含提示和範例的綜合指南,可協助您提高效能並優化 Laravel 應用程式。
1. 預加載與延遲加載
問題:預設情況下,Laravel 使用延遲載入,這可能會導致“N 1 查詢問題”,即不必要地觸發多個資料庫查詢。
最佳化:使用預先載入在一個查詢中載入相關數據,顯著提高處理關係時的效能。
之前(延遲載入):
// This runs multiple queries (N+1 Problem) $users = User::all(); foreach ($users as $user) { $posts = $user->posts; }
之後(預先載入):
// This loads users and their posts in just two queries $users = User::with('posts')->get();
關鍵要點:當您知道需要相關模型時,請務必使用預先載入。
2. 對昂貴的查詢使用快取
問題:頻繁取得相同的資料(如使用者清單、設定或產品目錄)可能會造成效能瓶頸。
最佳化:快取昂貴的查詢和計算的結果,以減少載入時間和資料庫查詢。
之前(無緩存):
// Querying the database every time $users = User::all();
之後(使用緩存):
// Caching the user data for 60 minutes $users = Cache::remember('users', 60, function () { return User::all(); });
重點:使用 Laravel 的快取系統(Redis、Memcached)來減少不必要的資料庫查詢。
3.優化資料庫查詢
問題:低效查詢和缺乏適當的索引會大幅降低效能。
最佳化:始終將索引新增至經常查詢的列,並僅使用所需的資料。
前:
// Fetching all columns (bad practice) $orders = Order::all();
後:
// Only fetching necessary columns and applying conditions $orders = Order::select('id', 'status', 'created_at') ->where('status', 'shipped') ->get();
關鍵要點:始終指定您需要的列,並確保您的資料庫在經常查詢的欄位上有正確的索引。
4. 盡量減少中間件的使用
問題:將中間件全域套用到每條路由會增加不必要的開銷。
最佳化:僅在需要的地方選擇性地應用中間件。
之前(全域中間件使用):
// Applying middleware to all routes Route::middleware('logRouteAccess')->group(function () { Route::get('/profile', 'UserProfileController@show'); Route::get('/settings', 'UserSettingsController@index'); });
之後(選擇性中間件使用):
// Apply middleware only to specific routes Route::get('/profile', 'UserProfileController@show')->middleware('logRouteAccess');
關鍵要點:中間件應僅在必要時應用,以避免效能下降。
5. 優化大型資料集的分頁
問題:一次取得和顯示大型資料集可能會導致記憶體使用率高且反應慢。
最佳化:使用分頁限制每個請求所取得的記錄數量。
之前(取得所有記錄):
// Fetching all users (potentially too much data) $users = User::all();
之後(使用分頁):
// Fetching users in chunks of 10 records per page $users = User::paginate(10);
關鍵要點:對大型資料集進行分頁以避免資料庫不堪重負並減少記憶體使用。
6. 對長時間運行的任務進行排隊
問題:發送電子郵件或產生報告等長時間運行的任務會減慢請求回應時間。
最佳化:使用佇列卸載任務並在後台非同步處理它們。
之前(同步任務):
// Sending email directly (slows down response) Mail::to($user->email)->send(new OrderShipped($order));
之後(排隊任務):
// Queuing the email for background processing Mail::to($user->email)->queue(new OrderShipped($order));
關鍵要點:對時間不敏感的任務使用佇列來縮短反應時間。
7. 使用路由、設定和視圖快取
問題:不快取路由、配置或視圖可能會導致效能下降,尤其是在生產環境中。
最佳化:快取路由、設定檔和視圖,以提高生產效能。
命令範例:
# Cache routes php artisan route:cache # Cache configuration files php artisan config:cache # Cache compiled views php artisan view:cache
關鍵要點:始終在生產環境中快取您的配置、路由和視圖,以提高應用程式效能。
8. 使用compact()來清理程式碼
問題:手動將多個變數傳遞給視圖可能會導致程式碼冗長且難以管理。
最佳化:使用compact()簡化將多個變數傳遞到視圖的過程。
前:
return view('profile', [ 'user' => $user, 'posts' => $posts, 'comments' => $comments, ]);
後:
return view('profile', compact('user', 'posts', 'comments'));
重點:使用compact()可以讓你的程式碼更簡潔,更容易維護。
9. Use Redis or Memcached for Session and Cache Storage
Problem: Storing sessions and cache data in the file system slows down your application in high-traffic environments.
Optimization: Use fast in-memory storage solutions like Redis or Memcached for better performance.
Example Config for Redis:
// In config/cache.php 'default' => env('CACHE_DRIVER', 'redis'), // In config/session.php 'driver' => env('SESSION_DRIVER', 'redis'),
Key Takeaway: Avoid using the file driver for sessions and caching in production, especially in high-traffic applications.
10. Avoid Using Raw Queries Unless Necessary
Problem: Using raw SQL queries can make your code less readable and harder to maintain.
Optimization: Use Laravel’s Eloquent ORM or Query Builder whenever possible, but if raw queries are necessary, ensure they are optimized.
Before (Raw Query):
// Using raw query directly $users = DB::select('SELECT * FROM users WHERE status = ?', ['active']);
After (Using Eloquent or Query Builder):
// Using Eloquent ORM for better readability and maintainability $users = User::where('status', 'active')->get();
Key Takeaway: Prefer Eloquent ORM over raw queries unless absolutely necessary.
11. Use Efficient Logging Levels
Problem: Logging everything at all times can cause performance degradation and fill up your storage.
Optimization: Set proper log levels in production to capture only what’s necessary (e.g., errors and critical messages).
Example:
// In .env file, set log level to 'error' in production LOG_LEVEL=error
Key Takeaway: Log only what’s necessary in production to avoid unnecessary storage usage and performance hits.
Final Thoughts
Optimizing Laravel performance is crucial for scalable and efficient applications. By implementing these best practices, you can ensure that your Laravel app runs faster, handles more traffic, and offers a better user experience.
Let me know what you think, or feel free to share your own tips and tricks for optimizing Laravel applications!
Happy coding! ?
以上是Laravel 中程式碼優化和效能改進的技巧的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
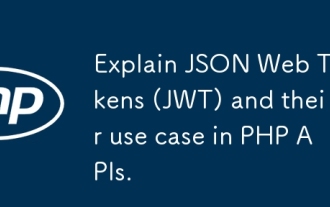
JWT是一種基於JSON的開放標準,用於在各方之間安全地傳輸信息,主要用於身份驗證和信息交換。 1.JWT由Header、Payload和Signature三部分組成。 2.JWT的工作原理包括生成JWT、驗證JWT和解析Payload三個步驟。 3.在PHP中使用JWT進行身份驗證時,可以生成和驗證JWT,並在高級用法中包含用戶角色和權限信息。 4.常見錯誤包括簽名驗證失敗、令牌過期和Payload過大,調試技巧包括使用調試工具和日誌記錄。 5.性能優化和最佳實踐包括使用合適的簽名算法、合理設置有效期、
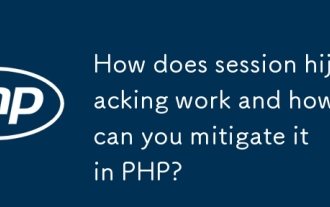
會話劫持可以通過以下步驟實現:1.獲取會話ID,2.使用會話ID,3.保持會話活躍。在PHP中防範會話劫持的方法包括:1.使用session_regenerate_id()函數重新生成會話ID,2.通過數據庫存儲會話數據,3.確保所有會話數據通過HTTPS傳輸。
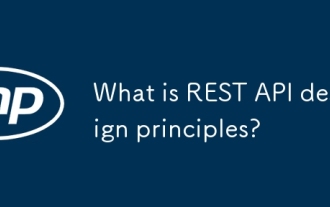
RESTAPI設計原則包括資源定義、URI設計、HTTP方法使用、狀態碼使用、版本控制和HATEOAS。 1.資源應使用名詞表示並保持層次結構。 2.HTTP方法應符合其語義,如GET用於獲取資源。 3.狀態碼應正確使用,如404表示資源不存在。 4.版本控制可通過URI或頭部實現。 5.HATEOAS通過響應中的鏈接引導客戶端操作。
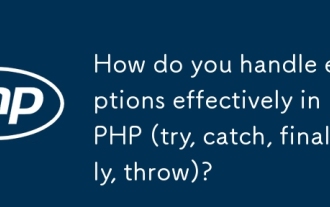
在PHP中,異常處理通過try,catch,finally,和throw關鍵字實現。 1)try塊包圍可能拋出異常的代碼;2)catch塊處理異常;3)finally塊確保代碼始終執行;4)throw用於手動拋出異常。這些機制幫助提升代碼的健壯性和可維護性。
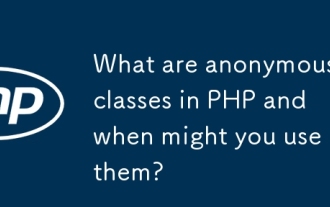
匿名類在PHP中的主要作用是創建一次性使用的對象。 1.匿名類允許在代碼中直接定義沒有名字的類,適用於臨時需求。 2.它們可以繼承類或實現接口,增加靈活性。 3.使用時需注意性能和代碼可讀性,避免重複定義相同的匿名類。
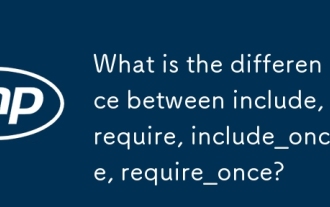
在PHP中,include,require,include_once,require_once的區別在於:1)include產生警告並繼續執行,2)require產生致命錯誤並停止執行,3)include_once和require_once防止重複包含。這些函數的選擇取決於文件的重要性和是否需要防止重複包含,合理使用可以提高代碼的可讀性和可維護性。
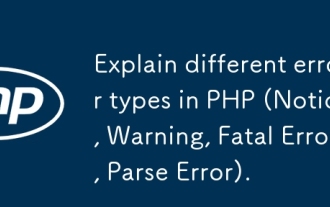
PHP中有四種主要錯誤類型:1.Notice:最輕微,不會中斷程序,如訪問未定義變量;2.Warning:比Notice嚴重,不會終止程序,如包含不存在文件;3.FatalError:最嚴重,會終止程序,如調用不存在函數;4.ParseError:語法錯誤,會阻止程序執行,如忘記添加結束標籤。
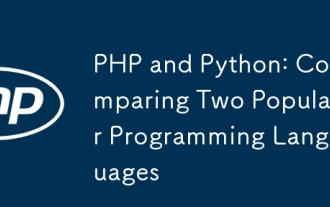
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
