使用 JavaScript 釋放大型語言模型的力量:實際應用程式
近年來,大型語言模型 (LLM) 徹底改變了我們與技術互動的方式,使機器能夠理解和產生類似人類的文本。 JavaScript 是一種用於 Web 開發的多功能語言,將 LLM 整合到您的應用程式中可以打開一個充滿可能性的世界。在這篇部落格中,我們將探索一些使用 JavaScript 的法學碩士令人興奮的實際用例,並提供範例以幫助您入門。
1. 透過智慧聊天機器人增強客戶支持
想像一下,有一個虛擬助理可以 24/7 處理客戶查詢,提供即時、準確的回應。法學碩士可用於建立能夠有效理解並回應客戶問題的聊天機器人。
例:客戶支援聊天機器人
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function getSupportResponse(query) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Customer query: "${query}". How should I respond?`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error generating response:', error); return 'Sorry, I am unable to help with that request.'; } } // Example usage const customerQuery = 'How do I reset my password?'; getSupportResponse(customerQuery).then(response => { console.log('Support Response:', response); });
透過此範例,您可以建立一個聊天機器人,為常見的客戶查詢提供有用的回應,從而改善使用者體驗並減少人工支援代理的工作量。
2. 透過自動化部落格大綱促進內容創建
創造引人入勝的內容可能是一個耗時的過程。法學碩士可以協助產生部落格文章大綱,使內容創建更加有效率。
範例:部落格文章大綱產生器
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function generateBlogOutline(topic) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Create a detailed blog post outline for the topic: "${topic}".`, max_tokens: 150, temperature: 0.7 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error generating outline:', error); return 'Unable to generate the blog outline.'; } } // Example usage const topic = 'The Future of Artificial Intelligence'; generateBlogOutline(topic).then(response => { console.log('Blog Outline:', response); });
此腳本可協助您快速為下一篇部落格文章產生結構化大綱,為您提供堅實的起點並節省內容建立流程的時間。
3.透過即時翻譯打破語言障礙
語言翻譯是法學碩士擅長的另一個領域。您可以利用法學碩士為使用不同語言的使用者提供即時翻譯。
範例:文字翻譯
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function translateText(text, targetLanguage) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Translate the following English text to ${targetLanguage}: "${text}"`, max_tokens: 60, temperature: 0.3 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error translating text:', error); return 'Translation error.'; } } // Example usage const text = 'Hello, how are you?'; translateText(text, 'French').then(response => { console.log('Translated Text:', response); });
透過此範例,您可以將翻譯功能整合到您的應用程式中,使其可供全球受眾使用。
4. 總結複雜的文本以便於理解
閱讀和理解冗長的文章可能具有挑戰性。法學碩士可以幫助總結這些文本,使它們更容易理解。
範例:文字摘要
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function summarizeText(text) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Summarize the following text: "${text}"`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error summarizing text:', error); return 'Unable to summarize the text.'; } } // Example usage const article = 'The quick brown fox jumps over the lazy dog. This sentence contains every letter of the English alphabet at least once.'; summarizeText(article).then(response => { console.log('Summary:', response); });
此程式碼片段可協助您建立長文章或文件的摘要,這對於內容管理和資訊傳播非常有用。
5. 協助開發人員產生程式碼
開發人員可以使用 LLM 產生程式碼片段,為編碼任務提供協助並減少編寫樣板程式碼所花費的時間。
範例:程式碼生成
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function generateCodeSnippet(description) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Write a JavaScript function that ${description}.`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error generating code:', error); return 'Unable to generate the code.'; } } // Example usage const description = 'calculates the factorial of a number'; generateCodeSnippet(description).then(response => { console.log('Generated Code:', response); });
透過此範例,您可以根據描述產生程式碼片段,使開發任務更有效率。
6. 提供個人化推薦
法學碩士可以幫助根據使用者興趣提供個人化推薦,增強使用者在各種應用中的體驗。
例:書籍推薦
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function recommendBook(interest) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Recommend a book for someone interested in ${interest}.`, max_tokens: 60, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error recommending book:', error); return 'Unable to recommend a book.'; } } // Example usage const interest = 'science fiction'; recommendBook(interest).then(response => { console.log('Book Recommendation:', response); });
此腳本根據使用者興趣提供個人化的書籍推薦,這對於創建量身定制的內容建議非常有用。
7. 透過概念解釋支持教育
法學碩士可以透過提供複雜概念的詳細解釋來協助教育,使學習更容易。
例:概念解釋
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function explainConcept(concept) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Explain the concept of ${concept} in detail.`, max_tokens: 150, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error explaining concept:', error); return 'Unable to explain the concept.'; } } // Example usage const concept = 'quantum computing'; explainConcept(concept).then(response => { console.log('Concept Explanation:', response); });
此範例有助於產生複雜概念的詳細解釋,有助於教育環境。
8. 起草個人化電子郵件回复
製作個人化回覆可能非常耗時。法學碩士可以幫助根據上下文和用戶輸入生成量身定制的電子郵件回复。
範例:電子郵件回覆起草
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function draftEmailResponse(emailContent) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Draft a response to the following email: "${emailContent}"`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error drafting email response:', error); return 'Unable to draft the email response.'; } } // Example usage const emailContent = 'I am interested in your product and would like more information.'; draftEmailResponse(emailContent).then(response => { console.log('Drafted Email Response:', response); });
此腳本會自動執行起草電子郵件回覆的過程,節省時間並確保一致的溝通。
9. 法律文件匯總
法律文件可能很密集且難以解析。法學碩士可以幫助總結這些文檔,使它們更易於訪問。
範例:法律文件摘要
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function summarizeLegalDocument(document) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Summarize the following legal document: "${document}"`, max_tokens: 150, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error summarizing document:', error); return 'Unable to summarize the document.'; } } // Example usage const document = 'This agreement governs the terms under which the parties agree to collaborate...'; summarizeLegalDocument(document).then(response => { console.log('Document Summary:', response); });
這個範例示範如何總結複雜的法律文檔,使它們更容易理解。
10. 解釋醫療狀況
醫療資訊可能很複雜且難以掌握。法學碩士可以對醫療狀況提供清晰簡潔的解釋。
範例:醫療狀況說明
const axios = require('axios'); // Replace with your OpenAI API key const apiKey = 'YOUR_OPENAI_API_KEY'; const apiUrl = 'https://api.openai.com/v1/completions'; async function explainMedicalCondition(condition) { try { const response = await axios.post(apiUrl, { model: 'text-davinci-003', prompt: `Explain the medical condition ${condition} in simple terms.`, max_tokens: 100, temperature: 0.5 }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data.choices[0].text.trim(); } catch (error) { console.error('Error explaining condition:', error); return 'Unable to explain the condition.'; } } // Example usage const condition = 'Type 2 Diabetes'; explainMedicalCondition(condition).then(response => { console.log('Condition Explanation:', response); });
該腳本提供了醫療狀況的簡化解釋,有助於患者教育和理解。
將 LLM 納入您的 JavaScript 應用程式可以顯著增強功能和使用者體驗。無論您是建立聊天機器人、生成內容還是協助教育,法學碩士都提供強大的功能來簡化和改進各種流程。透過將這些範例整合到您的專案中,您可以利用人工智慧的力量來創建更聰明、響應更靈敏的應用程式。
您可以根據您的特定需求和用例隨意調整和擴展這些範例。快樂編碼!
以上是使用 JavaScript 釋放大型語言模型的力量:實際應用程式的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
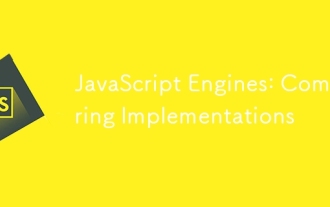
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
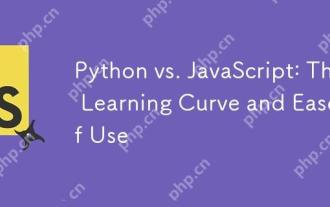
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
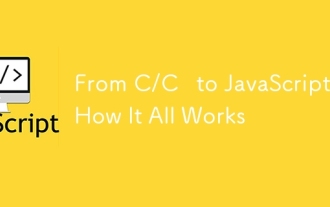
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
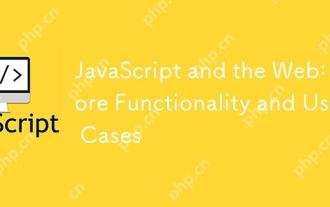
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。
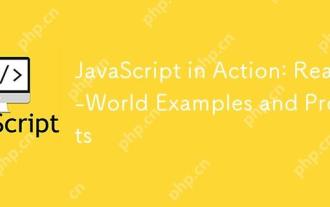
JavaScript在現實世界中的應用包括前端和後端開發。 1)通過構建TODO列表應用展示前端應用,涉及DOM操作和事件處理。 2)通過Node.js和Express構建RESTfulAPI展示後端應用。
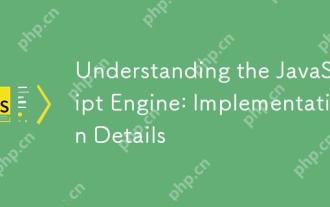
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。
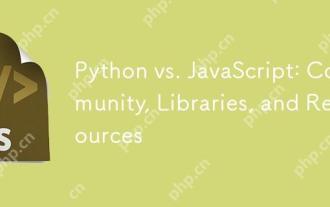
Python和JavaScript在社區、庫和資源方面的對比各有優劣。 1)Python社區友好,適合初學者,但前端開發資源不如JavaScript豐富。 2)Python在數據科學和機器學習庫方面強大,JavaScript則在前端開發庫和框架上更勝一籌。 3)兩者的學習資源都豐富,但Python適合從官方文檔開始,JavaScript則以MDNWebDocs為佳。選擇應基於項目需求和個人興趣。
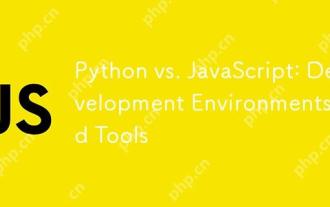
Python和JavaScript在開發環境上的選擇都很重要。 1)Python的開發環境包括PyCharm、JupyterNotebook和Anaconda,適合數據科學和快速原型開發。 2)JavaScript的開發環境包括Node.js、VSCode和Webpack,適用於前端和後端開發。根據項目需求選擇合適的工具可以提高開發效率和項目成功率。
