保護 Node.js API:身份驗證簡單指南
我建立了一個 Node.js API 並想要保護它,所以我檢查了必須選擇的幾個選項。因此,我將引導您了解三種常見的驗證方法:基本驗證、JWT(JSON Web 令牌) 和 API 金鑰。
1. 基本認證
它是什麼?
基本驗證非常簡單。客戶端在 Authorization 標頭中隨每個請求發送使用者名稱和密碼。雖然它很容易實現,但它並不是最安全的,除非您使用 HTTPS,因為憑證僅採用 Base64 編碼(未加密)。
如何實施
要使用 Express 將基本驗證新增至您的 API,您需要:
- 安裝 basic-auth 套件:
npm install basic-auth
- 新增身份驗證中間件:
const express = require('express'); const basicAuth = require('basic-auth'); const app = express(); function auth(req, res, next) { const user = basicAuth(req); const validUser = user && user.name === 'your-username' && user.pass === 'your-password'; if (!validUser) { res.set('WWW-Authenticate', 'Basic realm="example"'); return res.status(401).send('Authentication required.'); } next(); } app.use(auth); app.get('/', (req, res) => { res.send('Hello, authenticated user!'); }); const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
測試它
使用curl測試您的基本驗證:
curl -u your-username:your-password http://localhost:3000/
提示:始終使用 HTTPS 上的基本驗證以確保憑證受到保護。
2.JWT(JSON網路令牌)
它是什麼?
JWT 是一種更安全且可擴展的使用者驗證方式。伺服器不會在每個請求中發送憑證,而是在登入時產生令牌。用戶端將此令牌包含在後續請求的 Authorization 標頭中。
如何實施
首先,安裝所需的軟體包:
npm install jsonwebtoken express-jwt
以下是如何設定 JWT 驗證的範例:
const express = require('express'); const jwt = require('jsonwebtoken'); const expressJwt = require('express-jwt'); const app = express(); const secret = 'your-secret-key'; // Middleware to protect routes const jwtMiddleware = expressJwt({ secret, algorithms: ['HS256'] }); app.use(express.json()); // Parse JSON bodies // Login route to generate JWT token app.post('/login', (req, res) => { const { username, password } = req.body; if (username === 'user' && password === 'password') { const token = jwt.sign({ username }, secret, { expiresIn: '1h' }); return res.json({ token }); } return res.status(401).json({ message: 'Invalid credentials' }); }); // Protected route app.get('/protected', jwtMiddleware, (req, res) => { res.send('This is a protected route. You are authenticated!'); }); const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
測試它
首先,登入取得令牌:
curl -X POST http://localhost:3000/login -d '{"username":"user","password":"password"}' -H "Content-Type: application/json"
然後,使用令牌存取受保護的路由:
curl -H "Authorization: Bearer <your-token>" http://localhost:3000/protected
JWT 很棒,因為令牌有過期時間,並且不必在每個請求中發送憑證。
3.API金鑰認證
它是什麼?
API 金鑰驗證很簡單:您為每個用戶端提供一個唯一的金鑰,並將其包含在請求中。它很容易實現,但不如 JWT 安全或靈活,因為相同的金鑰會一遍又一遍地重複使用。最終是一個強大的解決方案,可以輕鬆地用於限制 api 調用的數量,並且許多網站都在使用它。作為額外的安全措施,可以將請求限製到特定的 IP。
如何實施
您不需要任何特殊的軟體包,但使用 dotenv 來管理您的 API 金鑰是個好主意。首先,安裝dotenv:
npm install dotenv
然後,使用 API 金鑰驗證建立您的 API:
require('dotenv').config(); const express = require('express'); const app = express(); const API_KEY = process.env.API_KEY || 'your-api-key'; function checkApiKey(req, res, next) { const apiKey = req.query.api_key || req.headers['x-api-key']; if (apiKey === API_KEY) { next(); } else { res.status(403).send('Forbidden: Invalid API Key'); } } app.use(checkApiKey); app.get('/', (req, res) => { res.send('Hello, authenticated user with a valid API key!'); }); const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
測試它
您可以使用以下方法測試您的 API 金鑰驗證:
curl http://localhost:3000/?api_key=your-api-key
或使用自訂標頭:
curl -H "x-api-key: your-api-key" http://localhost:3000/
認證方法總結
-
基本驗證:
- 優點:易於設定。
- 缺點:憑證會隨每個請求一起傳送,因此應透過 HTTPS 使用。
- 用例:使用者數量較少的簡單 API。
-
JWT 驗證:
- 優點:安全、無狀態且可擴充性良好。
- 缺點:比基本驗證更複雜。
- 用例:需要強大安全性的可擴充 API。
-
API 金鑰驗證:
- 優點:簡單且使用廣泛。
- 缺點:與 JWT 相比,API 金鑰安全性較低,且較難管理。
- 使用案例:您想要在沒有使用者管理的情況下對客戶端進行身份驗證的簡單 API。
結論
如果您正在尋找快速簡單的方法,基本驗證 可以使用,但請記住使用 HTTPS。如果您想要更強大、可擴充的安全性,請選擇 JWT。對於輕量級或內部 API,API 金鑰 驗證可能就足夠了。
您打算使用哪種身份驗證方法或您有其他解決方案嗎?請在評論中告訴我!
以上是保護 Node.js API:身份驗證簡單指南的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
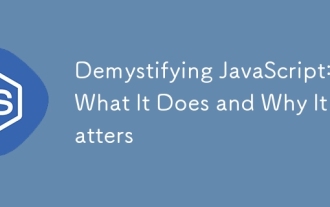
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
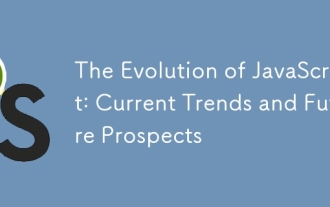
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
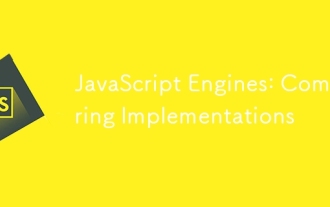
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
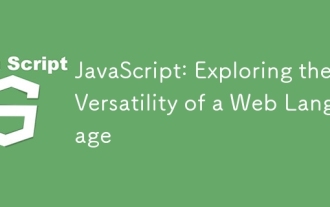
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
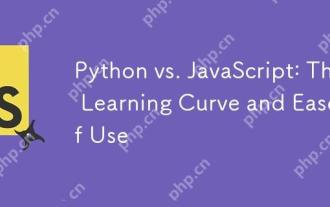
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
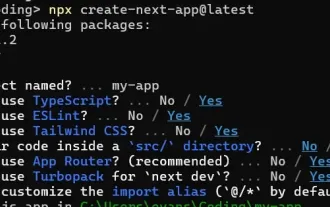
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
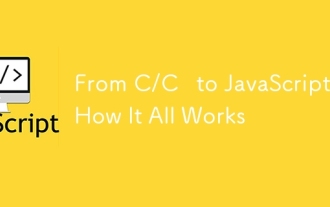
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
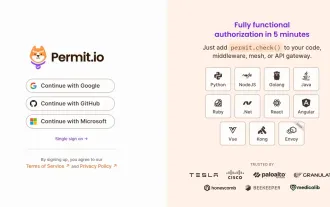
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
