字串前綴分數之和
2416。字串前綴分數總和
難度:難
主題:陣列、字串、Trie、計數
給你一個大小為 n 的單字數組,由 非空 字串組成。
我們將字串單字的分數定義為字串單字[i]的數量,這樣單字就是單字[i]的前綴。
- 例如,如果words = ["a", "ab", "abc", "cab"],那麼"ab"的分數是2,因為"ab"是"ab"和"ab"的前綴“ abc」。
傳回大小為n的陣列答案,其中answer[i]是單字[i]的每個非空前綴分數的總和。
注意字串被視為其自身的前綴。
範例1:
- 輸入:words = ["abc","ab","bc","b"]
- 輸出: [5,4,3,2]
-
解釋: 每個字串的答案如下:
- 「abc」有 3 個前綴:「a」、「ab」和「abc」。
- 有 2 個帶有前綴「a」的字串,2 個帶有前綴「ab」的字串,1 個帶有前綴「abc」的字串。
- 總數為答案[0] = 2 + 2 + 1 = 5。
- 「ab」有 2 個前綴:「a」和「ab」。
- 有 2 個帶有前綴「a」的字串,以及 2 個帶有前綴「ab」的字串。
- 總數為答案[1] = 2 + 2 = 4。
- 「bc」有 2 個前綴:「b」和「bc」。
- 有 2 個帶有前綴「b」的字串,1 個帶有前綴「bc」的字串。
- 總數為答案[2] = 2 + 1 = 3。
- 「b」有 1 個前綴:「b」。
- 有 2 個字串帶有前綴「b」。
- 總數為答案[3] = 2。
範例2:
- 輸入:words = ["abcd"]
- 輸出: [4]
-
說明:
- 「abcd」有 4 個前綴:「a」、「ab」、「abc」和「abcd」。
- 每個字首的得分為 1,因此總數為 answer[0] = 1 + 1 + 1 + 1 = 4。
約束:
- 1
- 1
- words[i] 由小寫英文字母組成。
提示:
- 什麼樣的資料結構可以讓您有效地追蹤每個前綴的分數?
- 使用特里樹。將所有單字插入其中,並在每個節點保留一個計數器,該計數器會告訴您我們訪問每個前綴的次數。
解:
我們可以使用 Trie 資料結構,它對於處理前綴特別有效。 Trie 中的每個節點都代表單字的一個字母,我們將在每個節點維護一個計數器來儲存遇到該前綴的次數。這使我們能夠透過計算有多少個單字以該前綴開頭來有效地計算每個前綴的分數。
方法:
-
將單字插入 Trie:
- 我們將逐個字元地將每個單字插入 Trie 中。
- 在每個節點(代表一個字元),我們維護一個計數器來追蹤有多少單字通過該前綴。
-
計算前綴分數:
- 對於每個單詞,當我們遍歷 Trie 中的前綴時,我們將對每個節點存儲的計數器求和,以計算每個前綴的分數。
-
建立答案陣列:
- 對於每個單詞,我們將計算其所有前綴的分數總和並將其儲存在結果數組中。
讓我們用 PHP 實作這個解:2416。字串前綴分數總和
<?php class TrieNode { /** * @var array */ public $children; /** * @var int */ public $count; public function __construct() { $this->children = []; $this->count = 0; } } class Trie { /** * @var TrieNode */ private $root; public function __construct() { $this->root = new TrieNode(); } /** * Insert a word into the Trie and update the prefix counts * * @param $word * @return void */ public function insert($word) { ... ... ... /** * go to ./solution.php */ } /** * Get the sum of prefix scores for a given word * * @param $word * @return int */ public function getPrefixScores($word) { ... ... ... /** * go to ./solution.php */ } } /** * @param String[] $words * @return Integer[] */ function sumOfPrefixScores($words) { ... ... ... /** * go to ./solution.php */ } // Example usage: $words1 = ["abc", "ab", "bc", "b"]; $words2 = ["abcd"]; print_r(sumOfPrefixScores($words1)); // Output: [5, 4, 3, 2] print_r(sumOfPrefixScores($words2)); // Output: [4] ?>
解釋:
-
TrieNode 類別:
- 每個節點都有一個子節點數組(代表單字中的下一個字元)和一個計數,用於追蹤有多少單字共享此前綴。
-
特里類:
- insert 方法在 Trie 中加入一個單字。當我們插入每個字元時,我們會增加每個節點的計數,表示有多少個單字具有此前綴。
- getPrefixScores 方法計算給定單字的所有前綴的分數總和。它遍歷 Trie,將單字中某個字元對應的每個節點的計數相加。
-
主要函數(sumOfPrefixScores):
- First, we insert all words into the Trie.
- Then, for each word, we calculate the sum of scores for its prefixes by querying the Trie and store the result in the result array.
Example:
For words = ["abc", "ab", "bc", "b"], the output will be:
Array ( [0] => 5 [1] => 4 [2] => 3 [3] => 2 )
- "abc" has 3 prefixes: "a" (2 words), "ab" (2 words), "abc" (1 word) -> total = 5.
- "ab" has 2 prefixes: "a" (2 words), "ab" (2 words) -> total = 4.
- "bc" has 2 prefixes: "b" (2 words), "bc" (1 word) -> total = 3.
- "b" has 1 prefix: "b" (2 words) -> total = 2.
Time Complexity:
- Trie Construction: O(n * m) where n is the number of words and m is the average length of the words.
- Prefix Score Calculation: O(n * m) as we traverse each word's prefix in the Trie.
This approach ensures that we efficiently compute the prefix scores in linear time relative to the total number of characters in all words.
Contact Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
- GitHub
以上是字串前綴分數之和的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
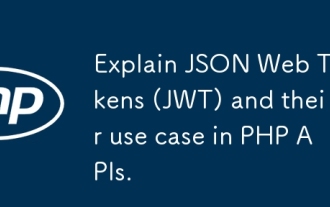
JWT是一種基於JSON的開放標準,用於在各方之間安全地傳輸信息,主要用於身份驗證和信息交換。 1.JWT由Header、Payload和Signature三部分組成。 2.JWT的工作原理包括生成JWT、驗證JWT和解析Payload三個步驟。 3.在PHP中使用JWT進行身份驗證時,可以生成和驗證JWT,並在高級用法中包含用戶角色和權限信息。 4.常見錯誤包括簽名驗證失敗、令牌過期和Payload過大,調試技巧包括使用調試工具和日誌記錄。 5.性能優化和最佳實踐包括使用合適的簽名算法、合理設置有效期、
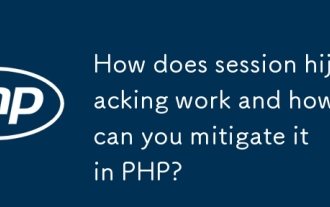
會話劫持可以通過以下步驟實現:1.獲取會話ID,2.使用會話ID,3.保持會話活躍。在PHP中防範會話劫持的方法包括:1.使用session_regenerate_id()函數重新生成會話ID,2.通過數據庫存儲會話數據,3.確保所有會話數據通過HTTPS傳輸。
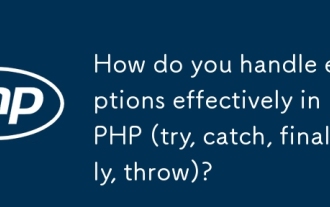
在PHP中,異常處理通過try,catch,finally,和throw關鍵字實現。 1)try塊包圍可能拋出異常的代碼;2)catch塊處理異常;3)finally塊確保代碼始終執行;4)throw用於手動拋出異常。這些機制幫助提升代碼的健壯性和可維護性。
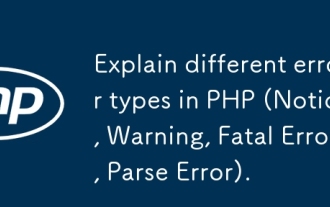
PHP中有四種主要錯誤類型:1.Notice:最輕微,不會中斷程序,如訪問未定義變量;2.Warning:比Notice嚴重,不會終止程序,如包含不存在文件;3.FatalError:最嚴重,會終止程序,如調用不存在函數;4.ParseError:語法錯誤,會阻止程序執行,如忘記添加結束標籤。
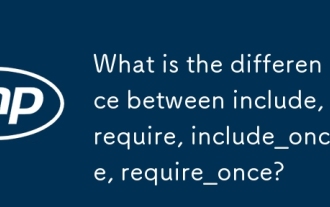
在PHP中,include,require,include_once,require_once的區別在於:1)include產生警告並繼續執行,2)require產生致命錯誤並停止執行,3)include_once和require_once防止重複包含。這些函數的選擇取決於文件的重要性和是否需要防止重複包含,合理使用可以提高代碼的可讀性和可維護性。
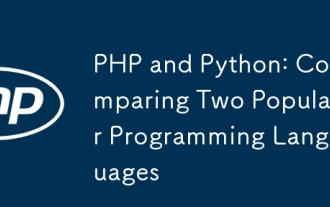
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
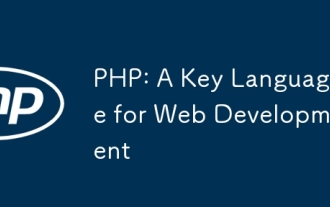
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
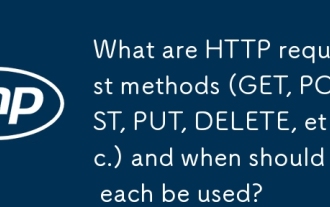
HTTP請求方法包括GET、POST、PUT和DELETE,分別用於獲取、提交、更新和刪除資源。 1.GET方法用於獲取資源,適用於讀取操作。 2.POST方法用於提交數據,常用於創建新資源。 3.PUT方法用於更新資源,適用於完整更新。 4.DELETE方法用於刪除資源,適用於刪除操作。
