在 Next.js 中上傳圖像(檔案上傳、Filestack)
1. Introduction
Uploading images in Next.js is a common task when developing web applications. In this tutorial, we will explore two different approaches:
- Uploading files directly to your backend (with Next.js).
- Using Filestack, a service that simplifies file management in the cloud.
Prerequisites:
- Have the latest version of Next.js installed.
- Basic knowledge of React and Node.js.
- For the Filestack section, you will need a Filestack account.
2. Uploading Images Directly (File Uploads)
First, let's see how you can handle file uploads directly in Next.js without using external services.
Step 1: Create the upload form in Next.js
Create a form in your desired component to select and upload an image. Here, we use useState to store the file and fetch to send it to the backend.
import { useState } from 'react'; export default function UploadForm() { const [selectedFile, setSelectedFile] = useState(null); const handleFileChange = (event) => { setSelectedFile(event.target.files[0]); }; const handleSubmit = async (event) => { event.preventDefault(); const formData = new FormData(); formData.append('file', selectedFile); const response = await fetch('/api/upload', { method: 'POST', body: formData, }); if (response.ok) { console.log('File uploaded successfully'); } else { console.error('Error uploading file'); } }; return ( <form onSubmit={handleSubmit}> <input type="file" onChange={handleFileChange} /> <button type="submit">Upload</button> </form> ); }
Step 2: Create the API to handle the upload
Now, create an endpoint in Next.js to process the image in the backend. We will use Next.js' API routes to handle server-side operations.
Create a file in pages/api/upload.js:
import fs from 'fs'; import path from 'path'; export const config = { api: { bodyParser: false, // Disable bodyParser to handle large files }, }; export default async function handler(req, res) { if (req.method === 'POST') { const chunks = []; req.on('data', (chunk) => { chunks.push(chunk); }); req.on('end', () => { const buffer = Buffer.concat(chunks); const filePath = path.resolve('.', 'uploads', 'image.png'); // Saves to the `uploads` folder fs.writeFileSync(filePath, buffer); res.status(200).json({ message: 'File uploaded successfully' }); }); } else { res.status(405).json({ message: 'Method not allowed' }); } }
Step 3: Create the upload folder
Make sure you have a folder called uploads in the root of your project. You can create it manually:
mkdir uploads
With this, you can now select an image and upload it directly to your server.
3. Uploading Images with Filestack
Now, if you prefer using an external service like Filestack, which offers file hosting, CDN, and efficient image handling, here's how you can do it.
Step 1: Install Filestack
First, we need to install the Filestack package in your Next.js project.
npm install filestack-js
Step 2: Set up Filestack in the frontend
Next, let's set up the Filestack widget in a component so that users can select and upload images. This is straightforward, as Filestack provides a ready-to-use widget.
Here’s an example implementation:
import { useState } from 'react'; import * as filestack from 'filestack-js'; const client = filestack.init('YOUR_API_KEY'); // Replace with your API Key export default function FilestackUpload() { const [imageUrl, setImageUrl] = useState(''); const handleUpload = async () => { const result = await client.picker({ onUploadDone: (res) => { setImageUrl(res.filesUploaded[0].url); console.log('File uploaded: ', res.filesUploaded[0].url); }, }).open(); }; return ( <div> <button onClick={handleUpload}>Upload Image with Filestack</button> {imageUrl && <img src={imageUrl} alt="Uploaded Image" />} </div> ); }
Step 3: Get your Filestack API Key
For Filestack to work, you need to create an account on Filestack and generate your API Key. Replace YOUR_API_KEY in the code above with the one provided to you.
Step 4: Display the uploaded image
Once the user uploads an image, you can display it using the URL generated by Filestack:
{imageUrl && <img src={imageUrl} alt="Uploaded Image" />}
4. Conclusion
And that’s it! You now have two approaches to handle image uploads in a Next.js application:
- Direct upload to your backend.
- Upload using Filestack, which gives you a more advanced and scalable solution if you don't want to manage file storage yourself.
以上是在 Next.js 中上傳圖像(檔案上傳、Filestack)的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
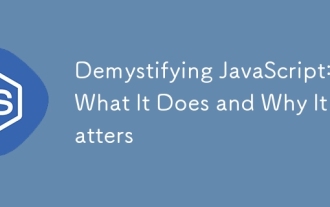
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
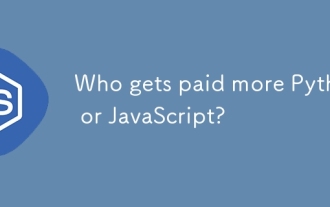
Python和JavaScript開發者的薪資沒有絕對的高低,具體取決於技能和行業需求。 1.Python在數據科學和機器學習領域可能薪資更高。 2.JavaScript在前端和全棧開發中需求大,薪資也可觀。 3.影響因素包括經驗、地理位置、公司規模和特定技能。
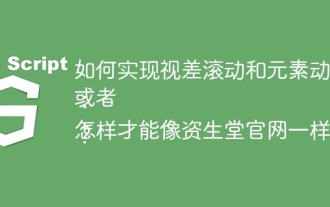
實現視差滾動和元素動畫效果的探討本文將探討如何實現類似資生堂官網(https://www.shiseido.co.jp/sb/wonderland/)中�...
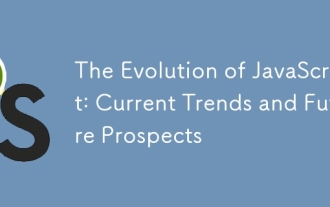
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
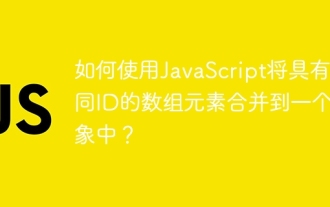
如何在JavaScript中將具有相同ID的數組元素合併到一個對像中?在處理數據時,我們常常會遇到需要將具有相同ID�...
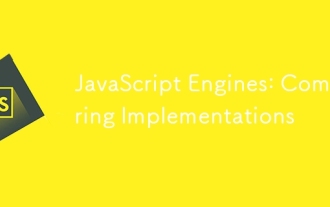
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
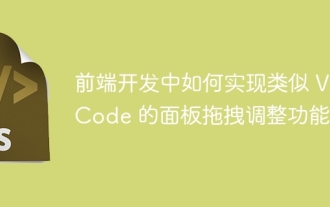
探索前端中類似VSCode的面板拖拽調整功能的實現在前端開發中,如何實現類似於VSCode...
