SharpAPI Laravel 整合指南
歡迎來到SharpAPI Laravel 整合指南!該儲存庫提供了有關如何將 SharpAPI 整合到下一個 Laravel AI 應用程式中的全面的逐步教學。無論您是想透過**人工智慧支援的功能**還是自動化工作流程來增強您的應用程序,本指南都將引導您完成從身份驗證到進行API 呼叫和處理響應的整個過程。
文章也作為 Github 儲存庫發佈在 https://github.com/sharpapi/laravel-ai-integration-guide。
目錄
- 先決條件
- 設定 Laravel 項目
- 安裝 SharpAPI PHP 用戶端
-
配置
- 環境變數
- 使用 SharpAPI 進行驗證
-
進行 API 呼叫
- 範例:產生職位描述
- 處理回應
- 錯誤處理
- 測試整合
-
進階用法
- 非同步請求
- 快取回應
- 結論
- 支持
- 許可證
先決條件
開始之前,請確保您已滿足以下要求:
- PHP:>= 8.1
- Composer:PHP 的依賴管理器
- Laravel:版本 9 或更高版本
- SharpAPI 帳號:從 SharpAPI.com 取得 API 金鑰
- Laravel基礎知識:熟悉Laravel框架與MVC架構
設定 Laravel 項目
如果你已經有Laravel項目,可以跳過這一步。否則,請按照以下說明建立一個新的 Laravel 專案。
- 透過 Composer 安裝 Laravel
composer create-project --prefer-dist laravel/laravel laravel-ai-integration-guide
- 導覽至專案目錄
cd laravel-ai-integration-guide
- 送達申請
php artisan serve
可以透過 http://localhost:8000 存取該應用程式。
安裝 SharpAPI PHP 用戶端
要與 SharpAPI 交互,您需要安裝 SharpAPI PHP 用戶端程式庫。
需要透過 Composer 取得 SharpAPI 套件
composer require sharpapi/sharpapi-laravel-client php artisan vendor:publish --tag=sharpapi-laravel-client
配置
環境變數
在環境變數中儲存 API 金鑰等敏感資訊是最佳實務。 Laravel 使用 .env 檔案進行特定於環境的配置。
- 開啟 .env 檔案
位於 Laravel 專案的根目錄中。
- 新增您的 SharpAPI API 金鑰
SHARP_API_KEY=your_actual_sharpapi_api_key_here
注意:將此處的 your_actual_sharpapi_api_key_key_替換為您實際的 SharpAPI API 金鑰。
- 在程式碼中存取環境變數
Laravel 提供了 env 輔助函數來存取環境變數。
$apiKey = env('SHARP_API_KEY');
使用 SharpAPI 進行身份驗證
需要進行身份驗證才能安全地與 SharpAPI 端點互動。
- 初始化 SharpAPI 用戶端
建立服務或直接在控制器中使用它。
<?php namespace App\Services; use SharpAPI\SharpApiService; class SharpApiClient { protected $client; public function __construct() { $this->client = new SharpApiService(env('SHARP_API_KEY')); } public function getClient() { return $this->client; } }
- 將服務綁定到服務提供者(可選)
這允許您在需要的地方注入服務。
<?php namespace App\Providers; use Illuminate\Support\ServiceProvider; use App\Services\SharpApiClient; class AppServiceProvider extends ServiceProvider { public function register() { $this->app->singleton(SharpApiClient::class, function ($app) { return new SharpApiClient(); }); } public function boot() { // } }
- 在控制器中使用服務
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Services\SharpApiClient; class SharpApiController extends Controller { protected $sharpApi; public function __construct(SharpApiClient $sharpApi) { $this->sharpApi = $sharpApi->getClient(); } public function ping() { $response = $this->sharpApi->ping(); return response()->json($response); } }
- 定義路線
將路由加入routes/web.php或routes/api.php:
use App\Http\Controllers\SharpApiController; Route::get('/sharpapi/ping', [SharpApiController::class, 'ping']);
進行 API 呼叫
經過驗證後,您可以開始對各種 SharpAPI 端點進行 API 呼叫。以下是如何與不同端點互動的範例。
範例:產生職位描述
- 建立作業描述參數 DTO
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Services\SharpApiClient; use SharpAPI\Dto\JobDescriptionParameters; class SharpApiController extends Controller { protected $sharpApi; public function __construct(SharpApiClient $sharpApi) { $this->sharpApi = $sharpApi->getClient(); } public function generateJobDescription() { $jobDescriptionParams = new JobDescriptionParameters( "Software Engineer", "Tech Corp", "5 years", "Bachelor's Degree in Computer Science", "Full-time", [ "Develop software applications", "Collaborate with cross-functional teams", "Participate in agile development processes" ], [ "Proficiency in PHP and Laravel", "Experience with RESTful APIs", "Strong problem-solving skills" ], "USA", true, // isRemote true, // hasBenefits "Enthusiastic", "Category C driving license", "English" ); $statusUrl = $this->sharpApi->generateJobDescription($jobDescriptionParams); $resultJob = $this->sharpApi->fetchResults($statusUrl); return response()->json($resultJob->getResultJson()); } }
- 定義路線
Route::get('/sharpapi/generate-job-description', [SharpApiController::class, 'generateJobDescription']);
- 存取端點
造訪 http://localhost:8000/sharpapi/generate-job-description 查看產生的職位說明。
處理回應
SharpAPI 回應通常封裝在作業物件中。要有效處理這些回應:
- 理解反應結構
{ "id": "uuid", "type": "JobType", "status": "Completed", "result": { // Result data } }
- 訪問結果
使用提供的方法存取結果資料。
$resultJob = $this->sharpApi->fetchResults($statusUrl); $resultData = $resultJob->getResultObject(); // As a PHP object // or $resultJson = $resultJob->getResultJson(); // As a JSON string
- Example Usage in Controller
public function generateJobDescription() { // ... (initialize and make API call) if ($resultJob->getStatus() === 'Completed') { $resultData = $resultJob->getResultObject(); // Process the result data as needed return response()->json($resultData); } else { return response()->json(['message' => 'Job not completed yet.'], 202); } }
Error Handling
Proper error handling ensures that your application can gracefully handle issues that arise during API interactions.
- Catching Exceptions
Wrap your API calls in try-catch blocks to handle exceptions.
public function generateJobDescription() { try { // ... (initialize and make API call) $resultJob = $this->sharpApi->fetchResults($statusUrl); return response()->json($resultJob->getResultJson()); } catch (\Exception $e) { return response()->json([ 'error' => 'An error occurred while generating the job description.', 'message' => $e->getMessage() ], 500); } }
- Handling API Errors
Check the status of the job and handle different statuses accordingly.
if ($resultJob->getStatus() === 'Completed') { // Handle success } elseif ($resultJob->getStatus() === 'Failed') { // Handle failure $error = $resultJob->getResultObject()->error; return response()->json(['error' => $error], 400); } else { // Handle other statuses (e.g., Pending, In Progress) return response()->json(['message' => 'Job is still in progress.'], 202); }
Testing the Integration
Testing is crucial to ensure that your integration with SharpAPI works as expected.
- Writing Unit Tests
Use Laravel's built-in testing tools to write unit tests for your SharpAPI integration.
<?php namespace Tests\Feature; use Tests\TestCase; use App\Services\SharpApiClient; use SharpAPI\Dto\JobDescriptionParameters; class SharpApiTest extends TestCase { protected $sharpApi; protected function setUp(): void { parent::setUp(); $this->sharpApi = new SharpApiClient(); } public function testPing() { $response = $this->sharpApi->ping(); $this->assertEquals('OK', $response['status']); } public function testGenerateJobDescription() { $jobDescriptionParams = new JobDescriptionParameters( "Backend Developer", "InnovateTech", "3 years", "Bachelor's Degree in Computer Science", "Full-time", ["Develop APIs", "Optimize database queries"], ["Proficiency in PHP and Laravel", "Experience with RESTful APIs"], "USA", true, true, "Professional", "Category B driving license", "English" ); $statusUrl = $this->sharpApi->generateJobDescription($jobDescriptionParams); $resultJob = $this->sharpApi->fetchResults($statusUrl); $this->assertEquals('Completed', $resultJob->getStatus()); $this->assertNotEmpty($resultJob->getResultObject()); } // Add more tests for other methods... }
- Running Tests
Execute your tests using PHPUnit.
./vendor/bin/phpunit
Advanced Usage
Asynchronous Requests
For handling multiple API requests concurrently, consider implementing asynchronous processing using Laravel Queues.
- Setting Up Queues
Configure your queue driver in the .env file.
QUEUE_CONNECTION=database
Run the necessary migrations.
php artisan queue:table php artisan migrate
- Creating a Job
php artisan make:job ProcessSharpApiRequest
<?php namespace App\Jobs; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Foundation\Bus\Dispatchable; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Queue\SerializesModels; use App\Services\SharpApiClient; use SharpAPI\Dto\JobDescriptionParameters; class ProcessSharpApiRequest implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; protected $params; public function __construct(JobDescriptionParameters $params) { $this->params = $params; } public function handle(SharpApiClient $sharpApi) { $statusUrl = $sharpApi->generateJobDescription($this->params); $resultJob = $sharpApi->fetchResults($statusUrl); // Handle the result... } }
- Dispatching the Job
use App\Jobs\ProcessSharpApiRequest; public function generateJobDescriptionAsync() { $jobDescriptionParams = new JobDescriptionParameters( // ... parameters ); ProcessSharpApiRequest::dispatch($jobDescriptionParams); return response()->json(['message' => 'Job dispatched successfully.']); }
- Running the Queue Worker
php artisan queue:work
Caching Responses
To optimize performance and reduce redundant API calls, implement caching.
- Using Laravel's Cache Facade
use Illuminate\Support\Facades\Cache; public function generateJobDescription() { $cacheKey = 'job_description_' . md5(json_encode($jobDescriptionParams)); $result = Cache::remember($cacheKey, 3600, function () use ($jobDescriptionParams) { $statusUrl = $this->sharpApi->generateJobDescription($jobDescriptionParams); $resultJob = $this->sharpApi->fetchResults($statusUrl); return $resultJob->getResultJson(); }); return response()->json(json_decode($result, true)); }
- Invalidating Cache
When the underlying data changes, ensure to invalidate the relevant cache.
Cache::forget('job_description_' . md5(json_encode($jobDescriptionParams)));
Conclusion
Integrating SharpAPI into your Laravel application unlocks a myriad of AI-powered functionalities, enhancing your application's capabilities and providing seamless workflow automation. This guide has walked you through the essential steps, from setting up authentication to making API calls and handling responses. With the examples and best practices provided, you're well-equipped to leverage SharpAPI's powerful features in your Laravel projects.
Support
If you encounter any issues or have questions regarding the integration process, feel free to open an issue on the GitHub repository or contact our support team at contact@sharpapi.com.
License
This project is licensed under the MIT License.
以上是SharpAPI Laravel 整合指南的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
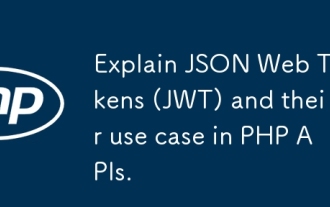
JWT是一種基於JSON的開放標準,用於在各方之間安全地傳輸信息,主要用於身份驗證和信息交換。 1.JWT由Header、Payload和Signature三部分組成。 2.JWT的工作原理包括生成JWT、驗證JWT和解析Payload三個步驟。 3.在PHP中使用JWT進行身份驗證時,可以生成和驗證JWT,並在高級用法中包含用戶角色和權限信息。 4.常見錯誤包括簽名驗證失敗、令牌過期和Payload過大,調試技巧包括使用調試工具和日誌記錄。 5.性能優化和最佳實踐包括使用合適的簽名算法、合理設置有效期、
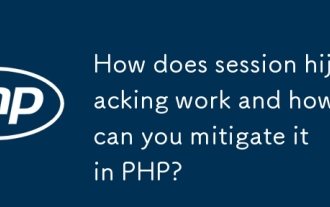
會話劫持可以通過以下步驟實現:1.獲取會話ID,2.使用會話ID,3.保持會話活躍。在PHP中防範會話劫持的方法包括:1.使用session_regenerate_id()函數重新生成會話ID,2.通過數據庫存儲會話數據,3.確保所有會話數據通過HTTPS傳輸。
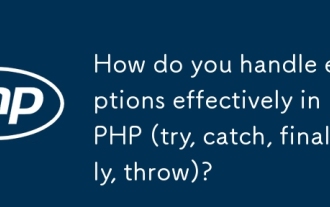
在PHP中,異常處理通過try,catch,finally,和throw關鍵字實現。 1)try塊包圍可能拋出異常的代碼;2)catch塊處理異常;3)finally塊確保代碼始終執行;4)throw用於手動拋出異常。這些機制幫助提升代碼的健壯性和可維護性。
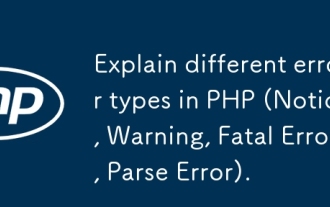
PHP中有四種主要錯誤類型:1.Notice:最輕微,不會中斷程序,如訪問未定義變量;2.Warning:比Notice嚴重,不會終止程序,如包含不存在文件;3.FatalError:最嚴重,會終止程序,如調用不存在函數;4.ParseError:語法錯誤,會阻止程序執行,如忘記添加結束標籤。
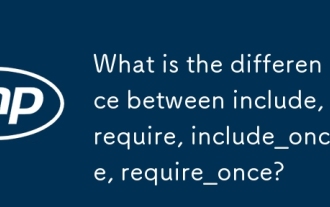
在PHP中,include,require,include_once,require_once的區別在於:1)include產生警告並繼續執行,2)require產生致命錯誤並停止執行,3)include_once和require_once防止重複包含。這些函數的選擇取決於文件的重要性和是否需要防止重複包含,合理使用可以提高代碼的可讀性和可維護性。
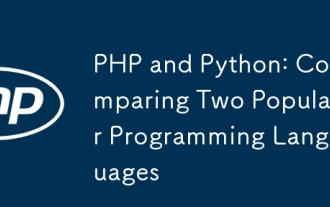
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
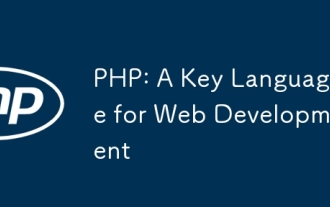
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
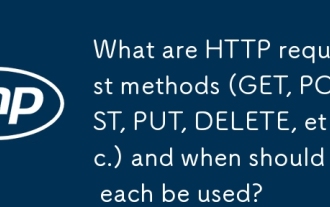
HTTP請求方法包括GET、POST、PUT和DELETE,分別用於獲取、提交、更新和刪除資源。 1.GET方法用於獲取資源,適用於讀取操作。 2.POST方法用於提交數據,常用於創建新資源。 3.PUT方法用於更新資源,適用於完整更新。 4.DELETE方法用於刪除資源,適用於刪除操作。
