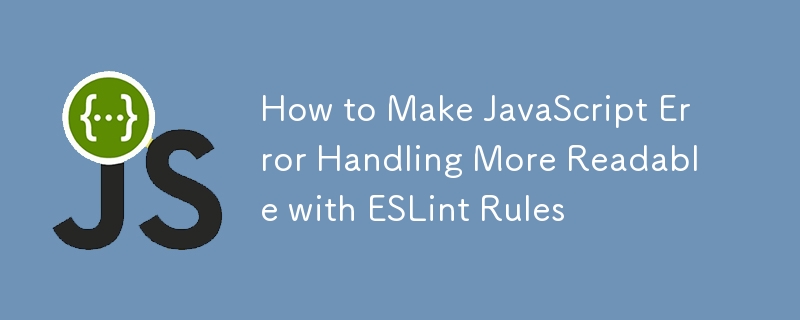
簡介:掌握 JavaScript 中的錯誤處理
有效的錯誤處理對於任何健全的 JavaScript 應用程式都至關重要。它有助於快速識別問題、簡化調試並增強軟體可靠性。本指南深入探討透過 ESLint 改進 JavaScript 錯誤處理,ESLint 是一種增強程式碼品質並標準化錯誤處理實作的工具。
為什麼要關注可讀的錯誤處理?
可讀的錯誤處理提供了對問題的即時洞察,幫助開發人員有效地理解和解決問題。這種做法在團隊環境中至關重要,對於長期維護程式碼也至關重要。
實施更好的錯誤處理實踐
要增強 JavaScript 錯誤處理能力,請考慮以下策略:
1. 有效使用 Try-Catch 區塊
1 2 3 4 5 6 | try {
const data = JSON.parse(response);
console.log(data);
} catch (error) {
console.error( "Failed to parse response:" , error);
}
|
登入後複製
2. 開發自訂錯誤類
1 2 3 4 5 6 7 8 9 10 11 12 | class ValidationError extends Error {
constructor(message) {
super(message);
this.name = "ValidationError" ;
}
}
try {
throw new ValidationError( "Invalid email address" );
} catch (error) {
console.error(error.name, error.message);
}
|
登入後複製
3. 確保詳細的錯誤記錄
1 2 3 | function handleError(error) {
console.error(`${ new Date ().toISOString()} - Error: ${error.message}`);
}
|
登入後複製
4. 區分投擲和非投擲函數
投擲版本:
1 2 3 | function calculateAge(dob) {
if (!dob) throw new Error( "Date of birth is required" );
}
|
登入後複製
非投擲版本:
1 2 3 4 5 6 | function tryCalculateAge(dob) {
if (!dob) {
console.error( "Date of birth is required" );
return null;
}
}
|
登入後複製
使用 ESLint 強制執行錯誤處理
設定 ESLint 來強制執行這些實踐涉及以下步驟和配置:
1. 安裝並設定 ESLint
1 2 | npm install eslint --save-dev
npx eslint --init
|
登入後複製
2. 設定 ESLint 錯誤處理規則
有效的錯誤處理對於開發健壯的 JavaScript 應用程式至關重要。以下是 ESLint 規則,可協助您在程式碼庫中實作良好的錯誤處理實務。
1. No Unhandled Promises
1 2 | "promise/no-return-in-finally" : "warn" ,
"promise/always-return" : "error"
|
登入後複製
-
Explanation:
This configuration ensures that promises always handle errors and don’t unintentionally suppress returned values in finally blocks.
2. No Await Inside a Loop
1 | "no-await-in-loop" : "error"
|
登入後複製
-
Explanation:
Awaits inside loops can lead to performance issues, as each iteration waits for a promise to resolve sequentially. It's better to use Promise.all() for handling multiple promises.
-
Example:
1 2 3 4 5 6 7 8 9 10 11 12 | async function processArray( array ) {
for (let item of array ) {
await processItem(item);
}
}
async function processArray( array ) {
const promises = array .map(item => processItem(item));
await Promise.all(promises);
}
|
登入後複製
3. Proper Error Handling in Async Functions
1 2 | "promise/catch-or-return" : "error" ,
"async-await/space-after-async" : "error"
|
登入後複製
-
Explanation:
Enforce that all asynchronous functions handle errors either by catching them or by returning the promise chain.
4. Consistent Return in Functions
1 | "consistent-return" : "error"
|
登入後複製
-
Explanation:
This rule enforces a consistent handling of return statements in functions, making it clear whether functions are expected to return a value or not, which is crucial for error handling and debugging.
5. Disallowing Unused Catch Bindings
1 2 | "no-unused-vars" : [ "error" , { "args" : "none" }],
"no-unused-catch-bindings" : "error"
|
登入後複製
-
Explanation:
Ensures that variables declared in catch blocks are used. This prevents ignoring error details and encourages proper error handling.
6. Enforce Throwing of Error Objects
1 | "no-throw-literal" : "error"
|
登入後複製
-
Explanation:
This rule ensures that only Error objects are thrown. Throwing literals or non-error objects often leads to less informative error messages and harder debugging.
-
Example:
1 2 3 4 5 | throw 'error' ;
throw new Error( 'An error occurred' );
|
登入後複製
7. Limiting Maximum Depth of Callbacks
1 | "max-nested-callbacks" : [ "warn" , 3]
|
登入後複製
-
Explanation:
Deeply nested callbacks can make code less readable and error-prone. Limiting the nesting of callbacks encourages simpler, more maintainable code structures.
8. Avoiding Unused Expressions in Error Handling
1 | "no-unused-expressions" : [ "error" , { "allowShortCircuit" : true, "allowTernary" : true}]
|
登入後複製
-
Explanation:
This rule aims to eliminate unused expressions which do not affect the state of the program and can lead to errors being silently ignored.
9. Require Error Handling in Callbacks
1 | "node/handle-callback-err" : "error"
|
登入後複製
-
Explanation:
Enforces handling error parameters in callbacks, a common pattern in Node.js and other asynchronous JavaScript code.
10. Disallowing the Use of Console
-
Explanation:
While not strictly an error handling rule, discouraging the use of console helps in avoiding leaking potentially sensitive error details in production environments and encourages the use of more sophisticated logging mechanisms.
3. Integrate ESLint into Your Development Workflow
Ensure ESLint runs automatically before code commits or during CI/CD processes.
Conclusion: Enhancing Code Quality with ESLint
By adopting these ESLint rules and error-handling strategies, you elevate the readability and reliability of your JavaScript applications. These improvements facilitate debugging and ensure a smoother user experience.
Final Thought
Are you ready to transform your error handling approach? Implement these practices in your projects to see a significant boost in your development efficiency and code quality. Embrace these enhancements and lead your projects to success.
以上是如何使用 ESLint 規則讓 JavaScript 錯誤處理更具可讀性的詳細內容。更多資訊請關注PHP中文網其他相關文章!