如何在 ReactJS 中使用 Axios - GET 和 POST 請求
如何在 ReactJS 中使用 Axios
介紹
Axios 是一個流行的函式庫,用於執行 GET、POST、PUT、DELETE 等 HTTP 請求。 Axios 非常適合在 React 應用程式中使用,因為它提供簡單的語法並支援 Promises。本文將討論如何在 ReactJS 應用程式中使用 Axios。
Axios 安裝
確保你的 React 專案中安裝了 Axios:
npm install axios
在 React 元件中使用 Axios
例如,我們希望使用 GET 方法從 API 檢索資料並將其顯示在 React 元件中。
- 取得請求:
import React, { useEffect, useState } from 'react'; import axios from 'axios'; const App = () => { const [data, setData] = useState([]); const [loading, setLoading] = useState(true); const [error, setError] = useState(null); useEffect(() => { const fetchData = async () => { try { const response = await axios.get('https://jsonplaceholder.typicode.com/posts'); setData(response.data); setLoading(false); } catch (error) { setError(error); setLoading(false); } }; fetchData(); }, []); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <div> <h1>Posts</h1> <ul> {data.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> </div> ); }; export default App;
說明:
- 在元件首次載入時使用useEffect呼叫fetchData函數。
- axios.get 用於從 API URL 檢索資料。
- 狀態資料、載入和錯誤用於儲存檢索到的資料、載入狀態和錯誤。
- POST 請求: 若要傳送數據,可以使用POST方法,如下所示:
import React, { useState } from 'react'; import axios from 'axios'; const App = () => { const [title, setTitle] = useState(''); const [body, setBody] = useState(''); const handleSubmit = async (e) => { e.preventDefault(); try { const response = await axios.post('https://jsonplaceholder.typicode.com/posts', { title, body, }); console.log('Response:', response.data); alert('Post successfully created!'); } catch (error) { console.error('Error posting data:', error); } }; return ( <div> <h1>Create a Post</h1> <form onSubmit={handleSubmit}> <input type="text" placeholder="Title" value={title} onChange={(e) => setTitle(e.target.value)} /> <textarea placeholder="Body" value={body} onChange={(e) => setBody(e.target.value)} ></textarea> <button type="submit">Submit</button> </form> </div> ); }; export default App;
說明:
- axios.post 方法用於將標題和正文資料傳送到 API。
- handleSubmit 函數處理表單提交並將資料傳送到伺服器。
以上是如何在 ReactJS 中使用 Axios - GET 和 POST 請求的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
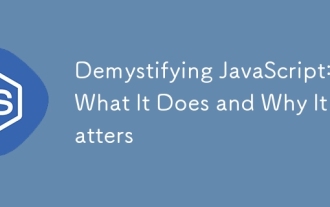
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
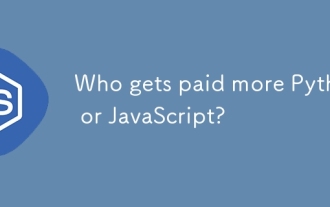
Python和JavaScript開發者的薪資沒有絕對的高低,具體取決於技能和行業需求。 1.Python在數據科學和機器學習領域可能薪資更高。 2.JavaScript在前端和全棧開發中需求大,薪資也可觀。 3.影響因素包括經驗、地理位置、公司規模和特定技能。
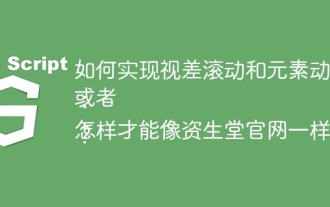
實現視差滾動和元素動畫效果的探討本文將探討如何實現類似資生堂官網(https://www.shiseido.co.jp/sb/wonderland/)中�...
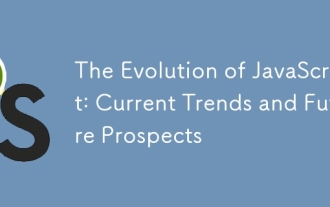
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
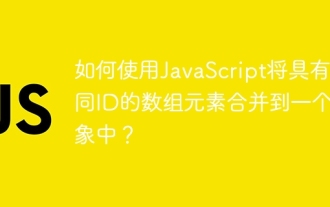
如何在JavaScript中將具有相同ID的數組元素合併到一個對像中?在處理數據時,我們常常會遇到需要將具有相同ID�...
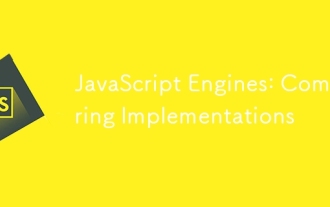
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
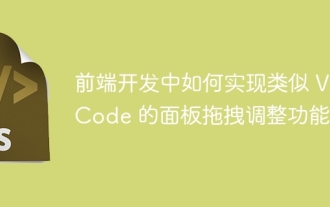
探索前端中類似VSCode的面板拖拽調整功能的實現在前端開發中,如何實現類似於VSCode...
