理解插入排序:問題驅動的方法
In this blog post, we'll take a question-driven approach to understand the fundamentals of the insertion sort algorithm. I came up with this approach when I was trying to find a better way to understand the insertion algorithm and others that I will soon be learning about. I wanted to build a strategy that I could apply to most, if not all, of the algorithms I will be learning. While I was thinking about this, I was sure that I might have to use first principles thinking
Inspired by first principles thinking, this approach involves first trying to grasp the algorithm, whether our initial understanding is vague or clear. We then identify the tiny concepts or mechanics involved that make up the algorithm. By forming questions around these mechanics or tiny concepts. We are essentially trying to understand the working of the algorithm from small different perspectives with a focus on trying to solve the questions that we formed on our own.
The answer you form may or may not initially resemble the syntax used in the actual algorithm. The goal should be to answer the question on your own, regardless of whether the syntax is close or not. Once you have a clear understanding, you can then convert, merge your answer(s) to use syntax, similar to the actual implementation of the algorithm. I believe this process allows you to explore alternative forms of code , grasp why a specific syntax is being used, deal with edge cases in a better way on your own.
I believe this method ensures that we understand the theory and the reasoning behind each line of code, making the implementation process more intuitive and meaningful. The following questions and the thought process which i went through helped me understand Insertion Sort better and enabled me to code it effectively.
For you, the questions might be different; they could be more, fewer, or entirely different. Some might say this is akin to reverse engineering, whatever you call it, this method enabled me get a thorough understanding of the Insertion Sort algorithm. I hope it does the same for you for any other other algorithm. Soo, let’s dive in!
Insertion Sort Implementation
This is the form of code we will eventually implement for Insertion Sort.
def insertion_sort(values): for new_value_index in range(1,len(values)): new_value = values[new_value_index] index = new_value_index-1 while index>=0: if values[index]<new_value:break values[index+1] = values[index] index-=1 values[index+1] = new_value
Questions
Given a sorted list, using while loop, print values from right to left.
values = [4,8,12,16,20,24,30] # given a sorted list, using while loop, print values from right to left. index = len(values)-1 while index>=0: print(values[index],end = " ") index-=1
Given a sorted list and a new value, find the index at which the new value is to be inserted to keep the list sorted.
values = [4, 8, 12, 16, 20, 24] new_value = 14 # using while loop, if traversing from right to left index = len(values)-1 while index>=0: if values[index]<new_value: break index-=1 print(values,new_value,index)
Given a sorted list and a new value, insert the new value to the list so it remains sorted.
values = [4, 8, 12, 16, 20, 24] new_value = 14 # if traversal from right to left index = len(values)-1 while index>=0: if values[index]<new_value:break index-=1 values = values[:index+1] + [new_value] + values[index+1:] print(values)
Given a sorted list, then appended with a new value, move the new value to the given index position.
values = [4, 8, 12, 16, 20, 24, 30] new_value = 14 values.append(new_value) given_index = 3 # above given n = len(values)-1 index = n-1 while index>given_index: values[index+1] = values[index] index-=1 print(values) values[given_index+1] = new_value print(values)
Given a sorted list, then appended with a new value, sort the list.
values = [4, 8, 12, 16, 20, 24, 30] new_value = 14 values.append(new_value) print(values) ### given a sorted list, then appended with new value, sort the list #### n = len(values)-1 new_value = values[-1] # find the index at which the value is to be inserted # right to left index = n-1 while index>=0: if values[index]<new_value:break index-=1 given_index = index print("given_index : " , given_index) # move the values forward by one step until we reach the given index index = n-1 while index>given_index: values[index+1] = values[index] index-=1 values[index+1] = new_value print(values)
Given a sorted list, then appended with a new value(s), sort the list.
values = [4, 8, 12, 16, 20, 24, 30] new_values = [14,32] values += new_values print(values) # given a sorted list, then appended with two new value(s), sort the list n = len(values)-1 new_value_start_index = n - 1 print(new_value_start_index, values[new_value_start_index]) for new_value_index in range(new_value_start_index,len(values)): new_value = values[new_value_index] index = new_value_index-1 while index>=0: if values[index]<new_value: break values[index+1] = values[index] index-=1 values[index+1] = new_value print(values)
Given a list, sort it.
import random values = random.sample(range(10,90), k = 10) values
print(values) for new_value_index in range(1,len(values)): new_value = values[new_value_index] index = new_value_index-1 while index>=0: if values[index]<new_value:break values[index+1] = values[index] index-=1 values[index+1] = new_value print(values)
Insertion Sort Implementation
def insertion_sort(values): for new_value_index in range(1,len(values)): new_value = values[new_value_index] index = new_value_index-1 while index>=0: if values[index]<new_value:break values[index+1] = values[index] index-=1 values[index+1] = new_value
Additional Resources
While I initially worked through a comprehensive set of questions to understand the algorithm better, the above are a set of questions that I think are important to understand Insertion Sort in a better way. Including all of the questions that I worked on would make the post quite lengthy.
For those interested in seeing all of the questions, I have created a Jupyter Notebook containing the full set of questions with my own answers, which enabled me to understand the implementation of Insertion Sort completely.
I encourage you to check out the notebook if you want to delve further.
Corrections and suggestions are welcome.
以上是理解插入排序:問題驅動的方法的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
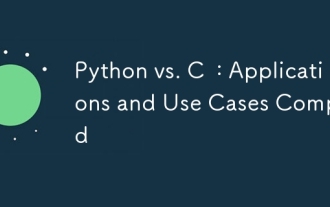
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
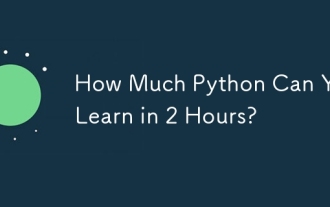
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
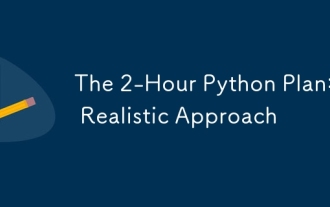
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
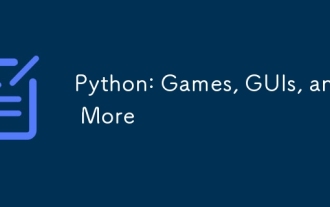
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
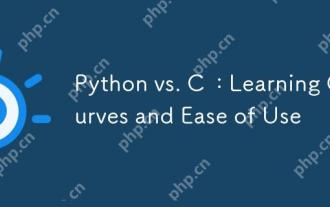
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
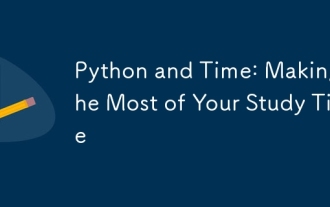
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
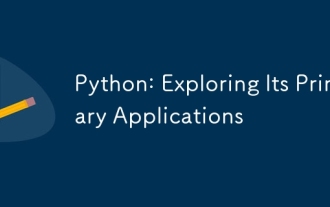
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
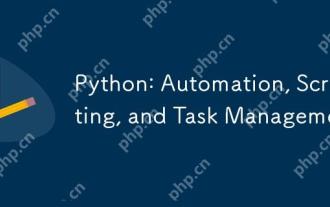
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
