LRU(最近最少使用)快取資料結構
LRU(最近最少使用)快取是一種緩存,當快取超出其容量時,它會逐出最近最少訪問的項目。它在記憶體有限且您只想快取最常存取的資料的場景中非常有用。
在 JavaScript 中,LRU 快取可以使用 Map(用於快速尋找和維護插入順序)和雙向鍊錶(用於兩端高效插入和刪除)的組合來實現。但是,為了簡單起見,我們將在以下實作中使用 Map。
這是 LRU 快取的 JavaScript 實作:
class LRUCache { constructor(capacity) { this.capacity = capacity; this.cache = new Map(); // Using Map to maintain key-value pairs } // Get the value from the cache get(key) { if (!this.cache.has(key)) { return -1; // If the key is not found, return -1 } // Key is found, move the key to the most recent position const value = this.cache.get(key); this.cache.delete(key); // Remove the old entry this.cache.set(key, value); // Reinsert to update its position (most recently used) return value; } // Add or update the value in the cache put(key, value) { if (this.cache.has(key)) { // If the key already exists, remove it to update its position this.cache.delete(key); } else if (this.cache.size >= this.capacity) { // If the cache is at capacity, delete the least recently used item const leastRecentlyUsedKey = this.cache.keys().next().value; this.cache.delete(leastRecentlyUsedKey); } // Insert the new key-value pair (most recent) this.cache.set(key, value); } }
說明:
建構子:LRUCache類別用給定的容量進行初始化,它使用Map來儲存快取的鍵值對。地圖會追蹤插入順序,這有助於識別最近最少使用 (LRU) 的項目。
取得(金鑰):
- 如果快取中存在該鍵,則該方法將返回其值,並透過先刪除該鍵然後重新插入該鍵將其移動到最近的位置。
- 如果鍵不存在,則回傳-1。
put(鍵,值):
- 如果快取中已存在該金鑰,則會刪除該金鑰並重新插入它(將其位置更新為最近使用的位置)。
- 如果快取達到其容量,它將刪除最近最少使用的鍵(Map 中的第一個鍵)。
- 最後,新的鍵值對被加入到快取中。
使用範例:
const lruCache = new LRUCache(3); // Cache with a capacity of 3 lruCache.put(1, 'one'); // Add key 1 lruCache.put(2, 'two'); // Add key 2 lruCache.put(3, 'three'); // Add key 3 console.log(lruCache.get(1)); // Output: 'one' (key 1 becomes the most recently used) lruCache.put(4, 'four'); // Cache is full, so it evicts key 2 (least recently used) console.log(lruCache.get(2)); // Output: -1 (key 2 has been evicted) console.log(lruCache.get(3)); // Output: 'three' (key 3 is still in the cache) console.log(lruCache.get(4)); // Output: 'four' (key 4 is in the cache)
以上是LRU(最近最少使用)快取資料結構的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
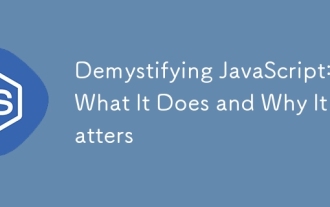
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
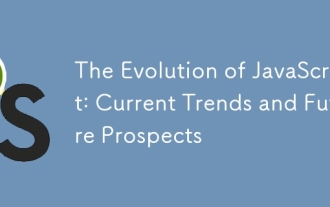
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
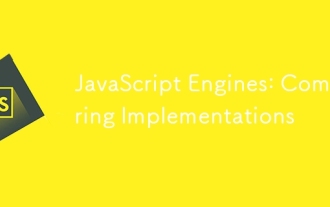
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
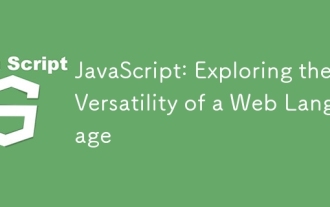
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
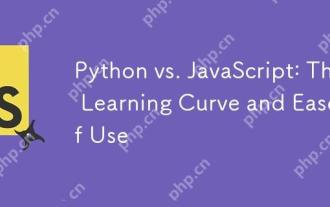
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
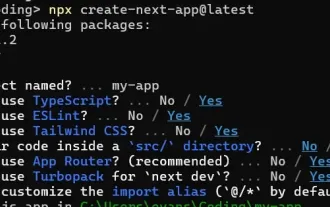
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
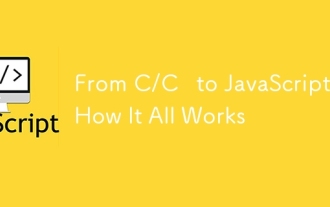
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
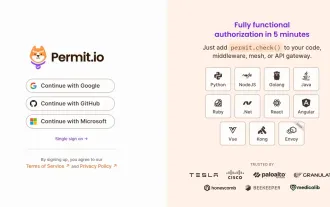
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
