如何使用內省、點擊和豐富格式為 Python CLI 建立互動式聊天
如果您曾經想讓您的 CLI 更具互動性和動態性,建立即時命令互動系統可能是答案。透過利用 Python 的自省功能、用於管理命令的 Click 以及用於格式化輸出的 Rich,您可以建立一個強大、靈活的 CLI,以智慧地回應使用者輸入。您的 CLI 可以自動發現並執行命令,而不是手動硬編碼每個命令,使用戶體驗更流暢、更具吸引力。
多彩的控制台混亂:點擊命令與豐富的輸出相遇 - 因為即使是終端也喜歡炫耀風格!
為什麼要使用 Click 和 Markdown?
Click 簡化了命令管理、參數解析和幫助產生。它還允許輕鬆的命令結構和選項處理。
Rich 讓您能夠直接在終端中輸出格式精美的 Markdown,使結果不僅實用,而且具有視覺吸引力。
透過將這兩個函式庫與 Python 自省結合,您可以建立互動式聊天功能,該功能可以動態發現和執行命令,同時以豐富、可讀的格式顯示輸出。 有關實際範例,請了解 StoryCraftr 如何使用類似的方法來簡化 AI 驅動的寫作工作流程: https://storycraftr.app。
建構互動聊天系統
1. 設定基本聊天指令
聊天指令初始化會話,允許使用者與 CLI 互動。在這裡,我們捕獲用戶輸入,這些輸入將動態映射到適當的 Click 命令。
import os import click import shlex from rich.console import Console from rich.markdown import Markdown console = Console() @click.command() @click.option("--project-path", type=click.Path(), help="Path to the project directory") def chat(project_path=None): """ Start a chat session with the assistant for the given project. """ if not project_path: project_path = os.getcwd() console.print( f"Starting chat for [bold]{project_path}[/bold]. Type [bold green]exit()[/bold green] to quit." ) # Start the interactive session while True: user_input = console.input("[bold blue]You:[/bold blue] ") # Handle exit if user_input.lower() == "exit()": console.print("[bold red]Exiting chat...[/bold red]") break # Call the function to handle command execution execute_cli_command(user_input)
2. 自省以發現和執行命令
使用Python內省,我們動態地發現可用的指令並執行它們。這裡的一個關鍵部分是 Click 指令是修飾函數。為了執行實際的邏輯,我們需要呼叫未修飾的函數(即回呼)。
以下是如何使用內省動態執行指令並處理 Click 的裝飾器:
import os import click import shlex from rich.console import Console from rich.markdown import Markdown console = Console() @click.command() @click.option("--project-path", type=click.Path(), help="Path to the project directory") def chat(project_path=None): """ Start a chat session with the assistant for the given project. """ if not project_path: project_path = os.getcwd() console.print( f"Starting chat for [bold]{project_path}[/bold]. Type [bold green]exit()[/bold green] to quit." ) # Start the interactive session while True: user_input = console.input("[bold blue]You:[/bold blue] ") # Handle exit if user_input.lower() == "exit()": console.print("[bold red]Exiting chat...[/bold red]") break # Call the function to handle command execution execute_cli_command(user_input)
這是如何運作的?
- 輸入解析:我們使用 shlex.split 來處理命令列參數等輸入。這可確保正確處理帶引號的字串和特殊字元。
- 模組和指令尋找:輸入分為 module_name 和 command_name。命令名稱經過處理,將連字符替換為下劃線,以匹配 Python 函數名稱。
- 內省:我們使用 getattr() 從模組動態取得指令函數。如果是 Click 命令(即具有回調屬性),我們透過剝離 Click 裝飾器來存取實際的函數邏輯。
- 命令執行:一旦我們檢索到未修飾的函數,我們就傳遞參數並呼叫它,就像直接呼叫 Python 函數一樣。
3. CLI 命令範例
讓我們考慮專案模組中的一些範例命令,使用者可以透過聊天互動來呼叫這些命令:
import inspect import your_project_cmd # Replace with your actual module containing commands command_modules = {"project": your_project_cmd} # List your command modules here def execute_cli_command(user_input): """ Function to execute CLI commands dynamically based on the available modules, calling the undecorated function directly. """ try: # Use shlex.split to handle quotes and separate arguments correctly parts = shlex.split(user_input) module_name = parts[0] command_name = parts[1].replace("-", "_") # Replace hyphens with underscores command_args = parts[2:] # Keep the rest of the arguments as a list # Check if the module exists in command_modules if module_name in command_modules: module = command_modules[module_name] # Introspection: Get the function by name if hasattr(module, command_name): cmd_func = getattr(module, command_name) # Check if it's a Click command and strip the decorator if hasattr(cmd_func, "callback"): # Call the underlying undecorated function cmd_func = cmd_func.callback # Check if it's a callable (function) if callable(cmd_func): console.print( f"Executing command from module: [bold]{module_name}[/bold]" ) # Directly call the function with the argument list cmd_func(*command_args) else: console.print( f"[bold red]'{command_name}' is not a valid command[/bold red]" ) else: console.print( f"[bold red]Command '{command_name}' not found in {module_name}[/bold red]" ) else: console.print(f"[bold red]Module {module_name} not found[/bold red]") except Exception as e: console.print(f"[bold red]Error executing command: {str(e)}[/bold red]")
執行聊天介面
運行互動式聊天系統:
- 確保您的模組(如項目)已在 command_modules 中列出。
- 運行指令:
@click.group() def project(): """Project management CLI.""" pass @project.command() def init(): """Initialize a new project.""" console.print("[bold green]Project initialized![/bold green]") @project.command() @click.argument("name") def create(name): """Create a new component in the project.""" console.print(f"[bold cyan]Component {name} created.[/bold cyan]") @project.command() def status(): """Check the project status.""" console.print("[bold yellow]All systems operational.[/bold yellow]")
會話開始後,使用者可以輸入以下命令:
python your_cli.py chat --project-path /path/to/project
輸出將使用 Rich Markdown 以格式良好的方式顯示:
You: project init You: project create "Homepage"
結論
透過結合 Click 指令管理、Rich for Markdown 格式和 Python 自省,我們可以為 CLI 建立一個強大的互動式聊天系統。這種方法可讓您動態發現和執行命令,同時以優雅、可讀的格式呈現輸出。
主要亮點:
- 動態命令執行:內省使您能夠發現和運行命令,而無需對其進行硬編碼。
- 豐富的輸出:使用 Rich Markdown 可確保輸出易於閱讀且具有視覺吸引力。
- 靈活性:此設定允許命令結構和執行的靈活性。
以上是如何使用內省、點擊和豐富格式為 Python CLI 建立互動式聊天的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
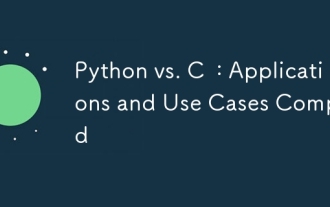
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
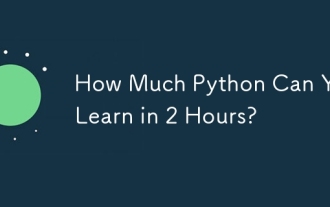
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
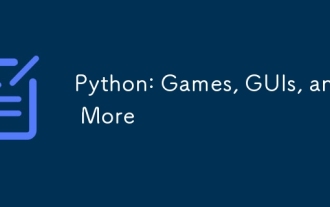
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
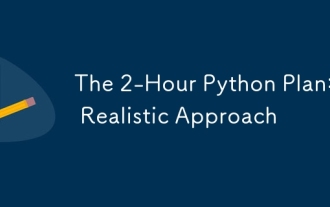
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
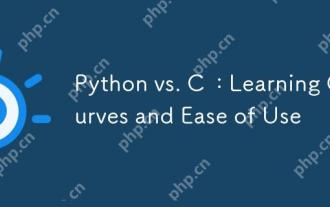
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
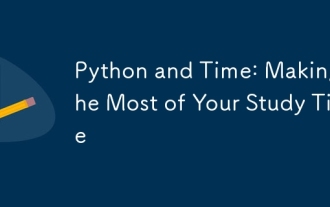
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
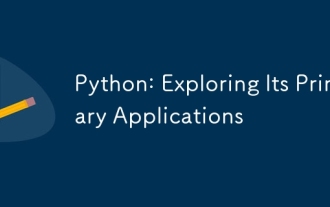
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
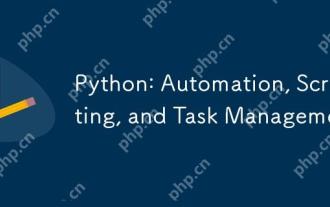
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
