如何在Python中計算目錄大小?
使用Python 計算目錄大小
在Python 中計算目錄的大小對於管理儲存空間或分析資料來說是一項有用的分析資料任務。讓我們探索如何有效地計算此大小。
使用 os.walk 求和檔案大小
一種方法涉及遍歷目錄及其子目錄,對檔案大小求和。這可以使用 os.walk 函數來實現:
<code class="python">import os def get_size(start_path='.'): total_size = 0 for dirpath, dirnames, filenames in os.walk(start_path): for f in filenames: fp = os.path.join(dirpath, f) if not os.path.islink(fp): total_size += os.path.getsize(fp) return total_size print(get_size(), 'bytes')</code>
此函數遞歸計算目錄大小,提供以位元組為單位的總大小。
單行使用os. listdir
要快速計算目錄大小而不考慮子目錄,可以使用單行程式碼:
<code class="python">import os sum(os.path.getsize(f) for f in os.listdir('.') if os.path.isfile(f))</code>
此表達式使用os.listdir 列出目錄中的所有檔案目前目錄,並使用os.path.getsize 確定其大小。
使用 os.stat 和 os.scandir
或者,您可以使用 os.stat 或os.scandir 來計算檔案大小。 os.stat 提供額外的文件信息,包括大小:
<code class="python">import os nbytes = sum(d.stat().st_size for d in os.scandir('.') if d.is_file())</code>
os.scandir 在 Python 3.5 中提供了改進的性能,並提供了更有效的方法來迭代目錄。
Pathlib解決方案
如果您使用的是Python 3.4 ,pathlib 庫提供了一種方便的方法來處理目錄操作:
<code class="python">from pathlib import Path root_directory = Path('.') sum(f.stat().st_size for f in root_directory.glob('**/*') if f.is_file())</code>
這個pathlib 解決方案結合了前面的方法,以實現簡潔和高效計算。
以上是如何在Python中計算目錄大小?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
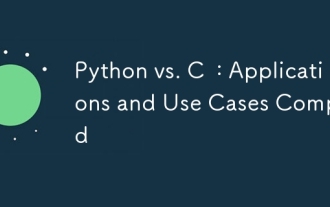
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
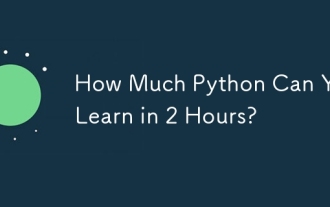
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
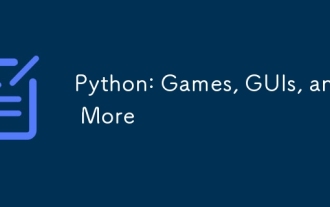
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
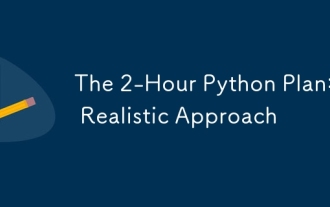
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
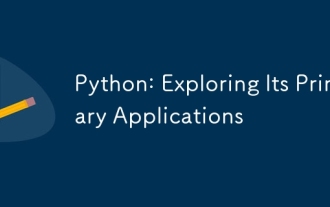
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
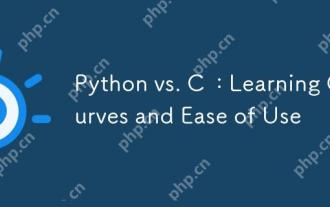
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
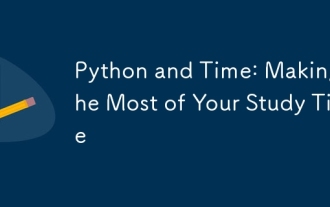
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
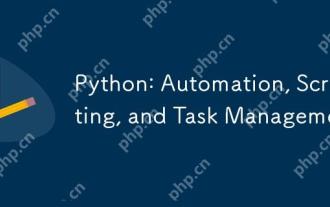
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
