Golang如何實作多通道並發讀取?
在Golang 中同時從多個通道讀取
在Golang 中,可以建立一個「任意對一」通道,其中多個goroutine 可以同時寫入同一個頻道。讓我們探討如何實現此功能。
一種方法是使用select 語句,它允許您等待多個通道接收資料:
<code class="go">func main() { // Create input channels c1 := make(chan int) c2 := make(chan int) // Create output channel out := make(chan int) // Start a goroutine that reads from both input channels and sums the received values go func(in1, in2 <-chan int, out chan<- int) { for { sum := 0 select { case sum = <-in1: sum += <-in2 case sum = <-in2: sum += <-in1 } out <- sum } }(c1, c2, out) }</code>
這個goroutine 無限期運行,讀取來自兩個通道並將接收到的值的總和會傳送到輸出通道。要終止 goroutine,需要關閉兩個輸入通道。
作為替代方法,您可以使用以下程式碼:
<code class="go">func addnum(num1, num2, sum chan int) { done := make(chan bool) go func() { n1 := <-num1 done <- true // Signal completion of one channel read }() n2 := <-num2 // Read from the other channel <-done // Wait for the first read to complete sum <- n1 + n2 }</code>
此函數使用單獨的「done」通道當一個通道已成功讀取時發出通知。但是,這種方法不太靈活,因為它需要修改寫入輸入通道的 goroutine。
適當的方法取決於應用程式的特定要求。無論您選擇哪種方法,Golang 的並發特性都提供了同時處理多個通道的強大工具。
以上是Golang如何實作多通道並發讀取?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
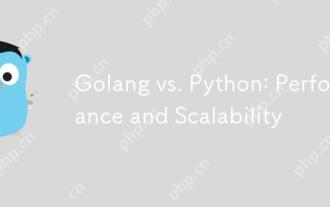
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
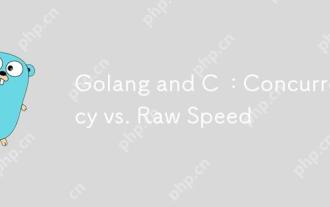
Golang在並發性上優於C ,而C 在原始速度上優於Golang。 1)Golang通過goroutine和channel實現高效並發,適合處理大量並發任務。 2)C 通過編譯器優化和標準庫,提供接近硬件的高性能,適合需要極致優化的應用。
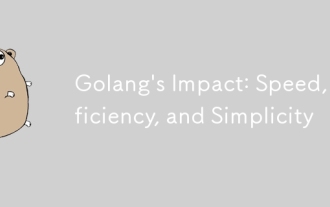
goimpactsdevelopmentpositationality throughspeed,效率和模擬性。 1)速度:gocompilesquicklyandrunseff,IdealforlargeProjects.2)效率:效率:ITScomprehenSevestAndardArdardArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增強的Depleflovelmentimency.3)簡單性。
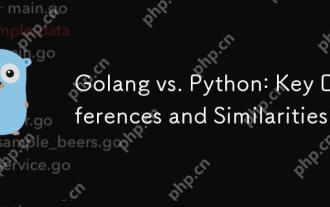
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。Golang以其并发模型和高效性能著称,Python则以简洁语法和丰富库生态系统著称。
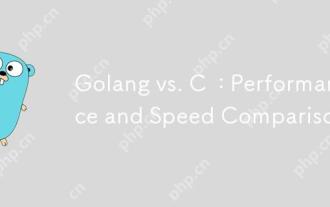
Golang適合快速開發和並發場景,C 適用於需要極致性能和低級控制的場景。 1)Golang通過垃圾回收和並發機制提升性能,適合高並發Web服務開發。 2)C 通過手動內存管理和編譯器優化達到極致性能,適用於嵌入式系統開發。
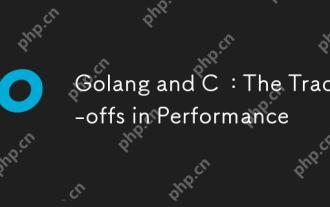
Golang和C 在性能上的差異主要體現在內存管理、編譯優化和運行時效率等方面。 1)Golang的垃圾回收機制方便但可能影響性能,2)C 的手動內存管理和編譯器優化在遞歸計算中表現更為高效。
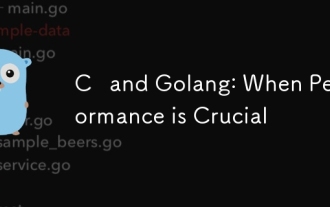
C 更適合需要直接控制硬件資源和高性能優化的場景,而Golang更適合需要快速開發和高並發處理的場景。 1.C 的優勢在於其接近硬件的特性和高度的優化能力,適合遊戲開發等高性能需求。 2.Golang的優勢在於其簡潔的語法和天然的並發支持,適合高並發服務開發。
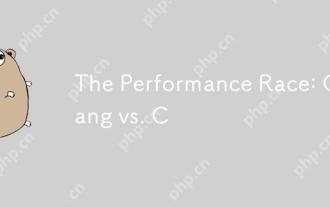
Golang和C 在性能競賽中的表現各有優勢:1)Golang適合高並發和快速開發,2)C 提供更高性能和細粒度控制。選擇應基於項目需求和團隊技術棧。
