建立用於動態檔案上傳和動態映像處理的 Express Web 應用程式
指南:建立用於檔案上傳和動態映像處理的 Express Web 應用程式
在本教學中,我們將向您展示如何使用Express.js 建立一個伺服器,該伺服器處理檔案上傳並使用Sharp.
先決條件在開始之前,請確保您已安裝
Node.js 和 npm。我們將在本教程中使用以下函式庫:
- Express.js - 用於設定伺服器。
- Multer - 用於處理檔案上傳。
- Sharp - 用於影像處理。
- CORS - 允許跨域請求。
第 1 步:設定項目
首先為您的專案建立一個新目錄:
mkdir image-upload-server cd image-upload-server npm init -y
您可以透過執行來安裝所有依賴項:
npm install express multer sharp cors
我們需要兩個目錄:
-
Original-image 用於儲存原始上傳的映像。
- 變換影像來儲存處理後的影像。
mkdir original-image transform-image
第 2 步:設定 Express 伺服器
現在,讓我們使用
Express.js 設定基本伺服器。在專案的根目錄中建立一個名為index.js的文件,並加入以下程式碼來設定伺服器:
const express = require('express'); const cors = require('cors'); const multer = require('multer'); const path = require('path'); const sharp = require('sharp'); const fs = require('fs'); const app = express(); // Middleware for CORS and JSON parsing app.use(cors()); app.use(express.json()); app.use(express.urlencoded({ extended: true }));
- CORS 允許跨域請求。
- express.json() 和 express.urlencoded() 用於解析傳入的請求資料。
步驟 3:設定 Multer 進行檔案上傳
我們將使用
Multer來處理檔案上傳。 Multer允許我們將上傳的檔案儲存在指定的目錄中。
加入以下程式碼來設定Multer:
// Configure multer for file storage const storage = multer.diskStorage({ destination: function (req, file, cb) { cb(null, 'original-image'); // Ensure the 'original-image' directory exists }, filename: function (req, file, cb) { const uniqueSuffix = Date.now() + '-' + Math.round(Math.random() * 1E9); cb(null, file.fieldname + '-' + uniqueSuffix + path.extname(file.originalname)); } }); const upload = multer({ storage: storage });
- 上傳的檔案儲存在original-image資料夾中。
- 每個檔案都會根據當前時間戳記和隨機數獲得一個唯一的名稱。
步驟 4:建立檔案上傳端點
接下來,建立一個用於檔案上傳的
POST 端點。使用者將檔案傳送到伺服器,伺服器會將檔案儲存在original-image目錄中。
加入以下程式碼來處理檔案上傳:
// File upload endpoint app.post('/upload', upload.single('file'), (req, res) => { const file = req.file; if (!file) { return res.status(400).send({ message: 'Please select a file.' }); } const url = `http://localhost:3000/${file.filename}`; // Store file path with original filename as the key db.set(file.filename, file.path); res.json({ message: 'File uploaded successfully.', url: url }); });
- 接收單一檔案上傳(帶有欄位名稱檔案)。
- 返回上傳文件的 URL。
第5步:提供上傳的文件
現在,讓我們建立一個 GET 端點來提供上傳的檔案。如果提供任何查詢參數(例如調整大小、格式轉換),伺服器將相應地處理映像。
加入以下程式碼來服務上傳的檔案:
mkdir image-upload-server cd image-upload-server npm init -y
此端點:
- 根據檔案名稱從資料庫對應檢索檔案。
- 如果指定了調整大小、格式轉換或品質調整,則處理影像。
- 快取處理後的影像以提高效能。
第 6 步:使用 Sharp 處理影像
Sharp 函式庫將允許我們對影像執行各種轉換,例如調整大小、格式轉換和品質調整。
加入處理這些轉換的 processImage 函數:
npm install express multer sharp cors
此功能:
- 根據 h(高度)和 w(寬度)參數調整影像大小。
- 根據f參數轉換影像格式(JPEG、PNG、WebP等)。
- 根據 q 參數調整影像品質(可選)。
- 將處理後的影像保存在transform-image資料夾中。
第7步:啟動伺服器
最後,加入以下程式碼啟動伺服器:
mkdir original-image transform-image
這將在連接埠 3000 上啟動伺服器。
第 8 步:測試伺服器
1. 使用Postman測試檔上傳
要使用 Postman 測試檔案上傳功能,請依照下列步驟操作:
1.1 打開郵差
在您的電腦上啟動 Postman。如果您沒有安裝 Postman,可以在這裡下載。
1.2 建立POST請求
- 將請求類型設定為POST。
- 在URL欄位中,輸入:http://localhost:3000/upload。
1.3 在Body中新增文件
- 選擇正文選項卡。
- 選擇表單資料選項。
- 在表單中,將鍵設為 file(這必須與您的 multer 配置中的欄位名稱相符)。
- 點擊選擇檔案按鈕並從您的電腦中選擇圖像檔案。
1.4 發送請求
- 點選發送。
- 如果上傳成功,您應該會收到包含上傳圖像 URL 的回應。
回應範例:
mkdir image-upload-server cd image-upload-server npm init -y
2. 透過瀏覽器測試影像檢索處理
現在,讓我們測試使用瀏覽器來擷取轉換後的影像。
2.1 取得上傳的圖片
要檢索圖像,只需打開瀏覽器並導航到上傳文件後收到的 URL。例如,如果回應 URL 為:
npm install express multer sharp cors
只需在瀏覽器的網址列中輸入此 URL,然後按 Enter。您應該會看到顯示的原始影像。
3. 使用查詢參數測試影像轉換
現在,讓我們透過附加用於調整大小、格式轉換和品質調整的查詢參數來測試動態影像轉換。
3.1 新增查詢參數進行轉換
在瀏覽器中,將查詢參數附加到圖像 URL 以測試轉換。以下是一些例子:
- 將影像大小調整為寬度 200px、高度 300px:
mkdir original-image transform-image
- 將影像轉換為PNG格式:
const express = require('express'); const cors = require('cors'); const multer = require('multer'); const path = require('path'); const sharp = require('sharp'); const fs = require('fs'); const app = express(); // Middleware for CORS and JSON parsing app.use(cors()); app.use(express.json()); app.use(express.urlencoded({ extended: true }));
- 將影像轉換為 WebP 格式,品質為 90%:
// Configure multer for file storage const storage = multer.diskStorage({ destination: function (req, file, cb) { cb(null, 'original-image'); // Ensure the 'original-image' directory exists }, filename: function (req, file, cb) { const uniqueSuffix = Date.now() + '-' + Math.round(Math.random() * 1E9); cb(null, file.fieldname + '-' + uniqueSuffix + path.extname(file.originalname)); } }); const upload = multer({ storage: storage });
- 將影像大小調整為寬度 400px、高度 500px,並轉換為 80% 品質的 JPEG:
// File upload endpoint app.post('/upload', upload.single('file'), (req, res) => { const file = req.file; if (!file) { return res.status(400).send({ message: 'Please select a file.' }); } const url = `http://localhost:3000/${file.filename}`; // Store file path with original filename as the key db.set(file.filename, file.path); res.json({ message: 'File uploaded successfully.', url: url }); });
3.2 預期行為
- 當您造訪任何帶有查詢參數的 URL 時,伺服器將相應地處理映像。
- 如果映像之前已經使用相同的參數進行過處理,它將提供快取版本。
- 如果尚未處理,它將處理圖像(調整大小,轉換格式,調整品質)並將其保存在transform-image資料夾中以供將來請求。
瀏覽器將顯示處理後的影像,您可以確認轉換是否已正確套用。
範例工作流程
- 透過 Postman 上傳圖片。
- 使用 Postman 提供的 URL 在瀏覽器中檢索上傳的圖像。
- 在瀏覽器中修改 URL,新增查詢參數,例如 ?h=300&w=200 以查看實際調整大小或 ?f=webp&q=90 以進行格式轉換。
結論
此映像上傳和處理伺服器為處理影像上傳、轉換和檢索提供了強大的解決方案。使用 Multer 進行檔案處理,使用 Sharp 進行影像處理,支援透過查詢參數調整大小、格式轉換和品質調整。該系統有效地快取處理後的圖像以優化性能,確保快速、響應靈敏的圖像傳輸。這種方法簡化了需要動態影像轉換的應用程式的影像管理,使其成為開發人員的多功能工具。
以上是建立用於動態檔案上傳和動態映像處理的 Express Web 應用程式的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
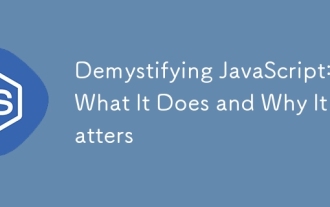
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
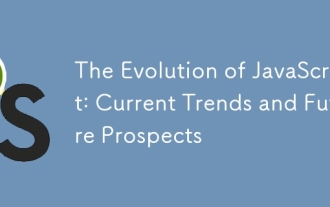
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
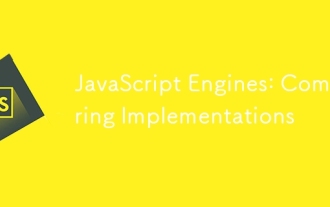
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
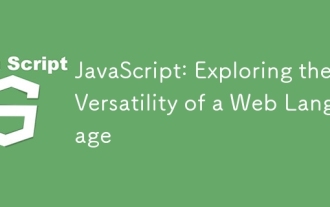
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
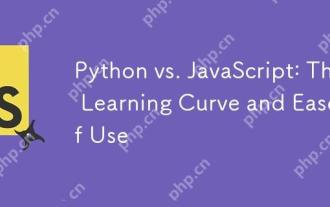
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
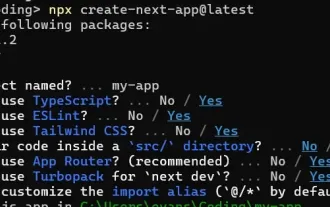
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
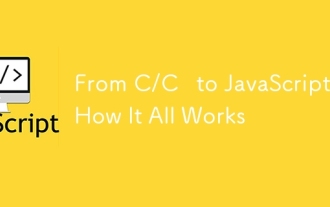
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
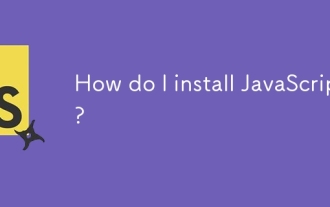
JavaScript不需要安裝,因為它已內置於現代瀏覽器中。你只需文本編輯器和瀏覽器即可開始使用。 1)在瀏覽器環境中,通過標籤嵌入HTML文件中運行。 2)在Node.js環境中,下載並安裝Node.js後,通過命令行運行JavaScript文件。
