如何使用 MySQLdb 和 MySQL Connector/Python 在 Python 中重新連接 MySQL 用戶端?
Nov 13, 2024 pm 02:23 PM重新連接 MySQL 用戶端與 MySQLdb
在資料庫連線領域,維持持久連線對於不間斷的資料存取至關重要。 MySQLdb 是一個流行的與 MySQL 資料庫介面的 Python 函式庫,它提供了一種在連線失敗時自動重新連線客戶端的方法。
在先前的版本中啟用自動重新連接
在早期版本的MySQLdb 中,可以透過mysql_options() 函數啟動自動重新連接功能,如下所示:
1 2 |
|
但是,隨著MySQL Connector/Python的出現,底層實作發生了變化,這種方式不再適用。
MySQL Connector/的解決方案Python
要在 MySQL Connector/Python 中實作自動重連,需要自訂 Cursor 物件的行為。以下程式碼片段示範了這個方法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
|
說明
此程式碼定義了包裝Cursor.execute() 方法的自訂query() 方法。如果發生連線錯誤(AttributeError 或 MySQLdb.OperationalError),query() 方法會嘗試重新連線到資料庫,然後重新執行查詢。
透過在遊標對象,可以確保你的Python腳本即使MySQL連線間歇性遺失也能繼續運作。這種方法提供了一種更強大、更可靠的方式來維護資料庫連線。
以上是如何使用 MySQLdb 和 MySQL Connector/Python 在 Python 中重新連接 MySQL 用戶端?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱門文章

熱門文章

熱門文章標籤

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
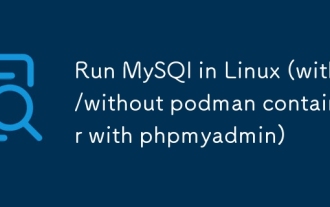
在 Linux 中運行 MySQl(有/沒有帶有 phpmyadmin 的 podman 容器)
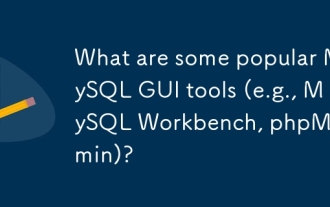
哪些流行的MySQL GUI工具(例如MySQL Workbench,PhpMyAdmin)是什麼?
