如何避免Java中日期字串解析異常?
用 Java 解決日期字串解析異常
將日期字串解析為 Date 物件是 Java 程式設計中的常見任務。但是,不正確的模式可能會導致異常。
請考慮以下範例:
此程式碼因模式不正確而引發 java.text.ParseException。要解決此問題,需要修改模式。
在這種特定情況下,應使用日 (EEE) 和月 (MMM) 的縮寫,而不是更緊湊的形式(E 和 MM) 。此外,該模式應明確指定語言環境為英語。這是因為預設語言環境在所有平台上可能不是英語。
以下是更正後的程式碼:
此更新的程式碼成功解析日期字串並產生正確的Date 物件:
在解析日期字串時使用正確的模式並指定區域設定以避免異常非常重要。
以上是如何避免Java中日期字串解析異常?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
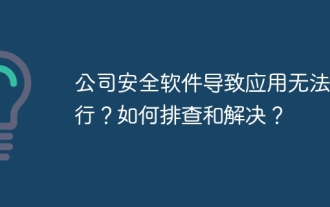
公司安全軟件導致部分應用無法正常運行的排查與解決方法許多公司為了保障內部網絡安全,會部署安全軟件。 ...
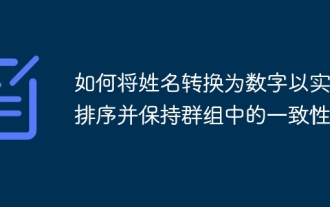
將姓名轉換為數字以實現排序的解決方案在許多應用場景中,用戶可能需要在群組中進行排序,尤其是在一個用...
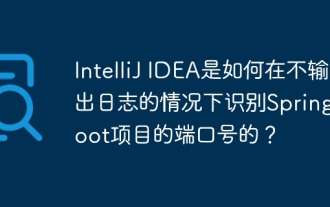
在使用IntelliJIDEAUltimate版本啟動Spring...
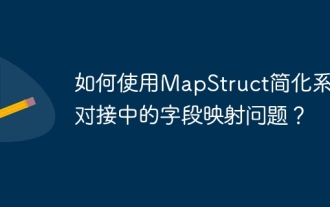
系統對接中的字段映射處理在進行系統對接時,常常會遇到一個棘手的問題:如何將A系統的接口字段有效地映�...
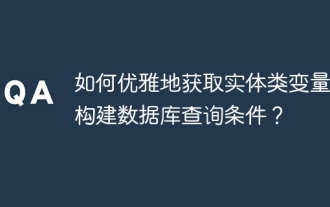
在使用MyBatis-Plus或其他ORM框架進行數據庫操作時,經常需要根據實體類的屬性名構造查詢條件。如果每次都手動...
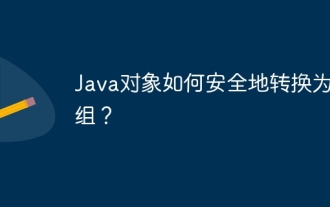
Java對象與數組的轉換:深入探討強制類型轉換的風險與正確方法很多Java初學者會遇到將一個對象轉換成數組的�...
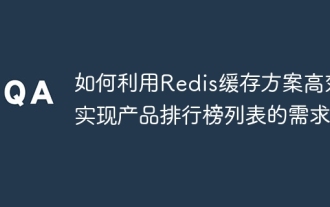
Redis緩存方案如何實現產品排行榜列表的需求?在開發過程中,我們常常需要處理排行榜的需求,例如展示一個�...
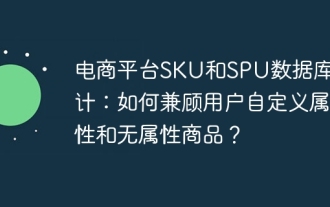
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
