為什麼 Python 集合看起來有一致的順序?
為什麼 Python 中的集合以看似一致的順序顯示?
雖然 Python 集合確實是無序的,但它們的顯示順序可能看起來是一致的。這個順序不是任意的,而是由底層哈希演算法和記憶體分配決定。
雜湊和記憶體放置
集合中的每個元素都經過雜湊處理,最後一個元素被雜湊處理。雜湊的 N 位元(其中 N 取決於集合大小)用作數組索引。然後將元素放置在記憶體中的這些索引處。因此,元素在記憶體中的順序決定了它們產生的順序。
衝突解決
但是,當多個元素具有相同的雜湊值時,就會出現衝突解決機制進入遊戲。這些機制將元素分配到不同的記憶體位置(備份位置)。發生這種情況的確切順序取決於首先到達的元素。
整數元素範例
考慮set_1 和set_2 的範例:
set_1 = set([5, 2, 7, 2, 1, 88]) set_2 = set([5, 2, 7, 2, 1, 88])
元素的雜湊中具有唯一的最後3 位,因此可以避免衝突。兩個集合中元素的順序被保留,因為它們是以相同的順序添加的。
字串元素範例
在set_3 和set_4 的情況下:
set_3 = set('abracadabra') set_4 = set('abracadabra')
再,由於雜湊中唯一的最後3 位元,避免了衝突。元素按照新增的順序生成,這兩個集合中的順序恰好相同。
不保證插入順序
需要注意的是,不保證集合中元素的順序。如果輸入清單重新排序,順序可能會有所不同,尤其是在發生衝突時。
效能影響
雜湊和記憶體分配過程可能會影響集合效能。例如,當具有相似雜湊值的元素數量增加時,衝突解決變得更加複雜,影響集合查找和插入操作。
以上是為什麼 Python 集合看起來有一致的順序?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
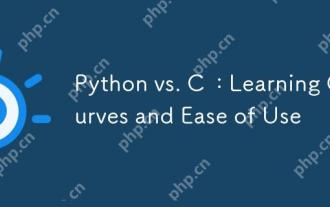
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
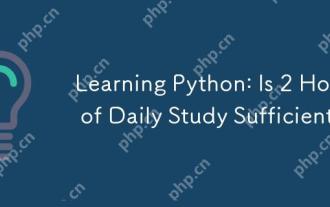
每天學習Python兩個小時是否足夠?這取決於你的目標和學習方法。 1)制定清晰的學習計劃,2)選擇合適的學習資源和方法,3)動手實踐和復習鞏固,可以在這段時間內逐步掌握Python的基本知識和高級功能。
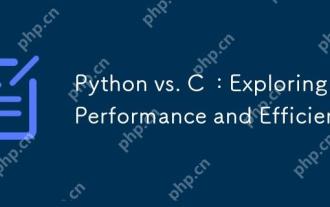
Python在開發效率上優於C ,但C 在執行性能上更高。 1.Python的簡潔語法和豐富庫提高開發效率。 2.C 的編譯型特性和硬件控制提升執行性能。選擇時需根據項目需求權衡開發速度與執行效率。
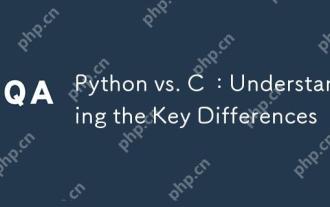
Python和C 各有優勢,選擇應基於項目需求。 1)Python適合快速開發和數據處理,因其簡潔語法和動態類型。 2)C 適用於高性能和系統編程,因其靜態類型和手動內存管理。
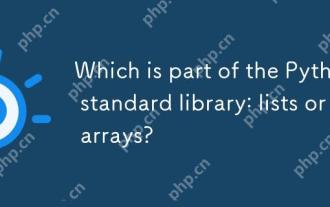
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
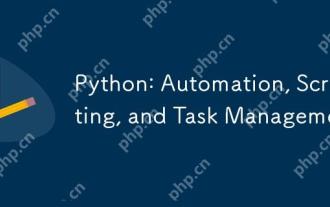
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
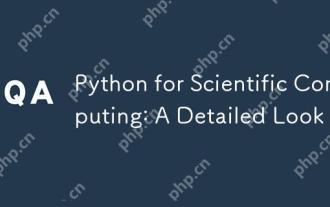
Python在科學計算中的應用包括數據分析、機器學習、數值模擬和可視化。 1.Numpy提供高效的多維數組和數學函數。 2.SciPy擴展Numpy功能,提供優化和線性代數工具。 3.Pandas用於數據處理和分析。 4.Matplotlib用於生成各種圖表和可視化結果。
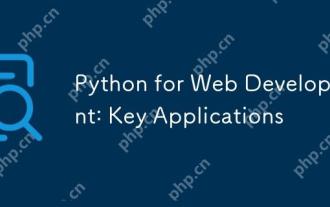
Python在Web開發中的關鍵應用包括使用Django和Flask框架、API開發、數據分析與可視化、機器學習與AI、以及性能優化。 1.Django和Flask框架:Django適合快速開發複雜應用,Flask適用於小型或高度自定義項目。 2.API開發:使用Flask或DjangoRESTFramework構建RESTfulAPI。 3.數據分析與可視化:利用Python處理數據並通過Web界面展示。 4.機器學習與AI:Python用於構建智能Web應用。 5.性能優化:通過異步編程、緩存和代碼優
