從 JavaScript 發送 JSON 資料到 FastAPI 如何處理錯誤?
將JSON 資料從JavaScript 發佈到FastAPI 時的錯誤處理
要將資料從JavaScript 前端傳送到FastAPI 後端,您必須確保資料以正確的格式傳遞到適當的端點。如果遇到 422 Unprocessable Entity 錯誤,很可能是因為資料格式不正確造成的。
查詢參數與 JSON 參數
預設情況下,FastAPI 將未包含在路徑中的函數參數解釋為 查詢參數。但是,對於JSON 數據,您需要使用以下方法之一明確指定它:
1. Pydantic 模型:
定義一個Pydantic 模型來表示JSON 資料結構:
from pydantic import BaseModel class Item(BaseModel): eth_addr: str @app.post('/ethAddress') def add_eth_addr(item: Item): return item
2. Body類型:
使用Body類型指定應從請求正文中解析參數:
from fastapi import Body @app.post('/ethAddress') def add_eth_addr(eth_addr: str = Body()): return {'eth_addr': eth_addr}
3. Body Embed:
對於單一body 參數,您可以使用embed=True參數自動解析請求正文中的資料:
from fastapi import Body @app.post('/ethAddress') def add_eth_addr(eth_addr: str = Body(embed=True)): return {'eth_addr': eth_addr}
JavaScript Fetch API
在JavaScript中使用Fetch API傳送JSON資料時,必須將Content-Type header設定為application/json並在body中指定資料欄位:
fetch("http://localhost:8000/ethAddress", { method: "POST", headers: { 'Accept': 'application/json', 'Content-Type': 'application/json' }, body: JSON.stringify({ "eth_addr": "some address" }), });
其他資源
有關更多資訊和詳細範例,請參閱以下文件和資源:
- [FastAPI JSON 請求和回應] (https://fastapi.tiangolo.com/tutorial/body/)
- 【透過POST請求發送JSON資料在JavaScript 中](https://stackoverflow.com/questions/44832885/sending-json- data-with-post-requests-in-javascript)
- [使用Fetch 在JavaScript 中傳送包含JSON內容的請求API](https://stackoverflow.com/questions/55749929/post-request-with-json-content-in-javascript-using-fetch-api)
以上是從 JavaScript 發送 JSON 資料到 FastAPI 如何處理錯誤?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
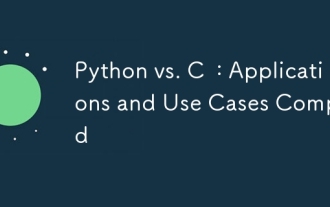
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
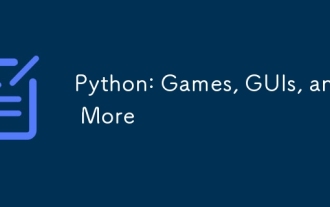
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
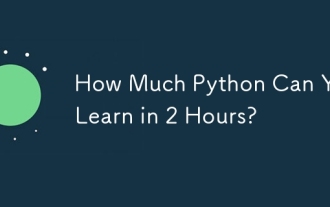
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
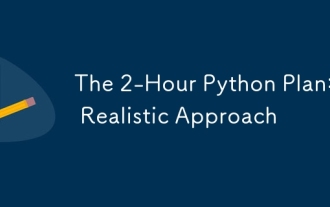
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
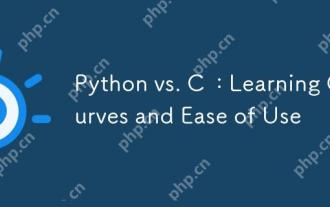
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
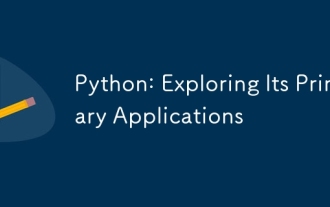
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
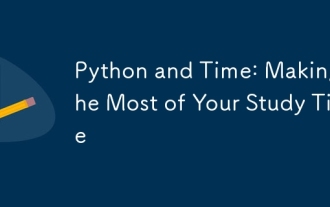
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
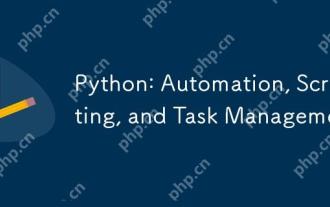
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
